The divide (/
) operator allows you to divide two numbers and get their quotient.
number / number
divide(number, number)
number.divide(number)
Code language: JavaScript (javascript)
The /
operator follows the standard mathematical order of operations (PEMDAS). For more detail, see Operator Precedence.
You can also use the function version, divide()
.
Example Formulas
12 / 4 /* Output: 3 */
divide(12, -4) /* Output: -3 */
12.divide(4) /* Output: 3 */
Code language: JavaScript (javascript)
Working with 3 or More Operands
Since divide is a binary operator, it can only work on two operands – the objects which are being operated on (if – the ternary operator – is the only operator that works on three operands).
If you need to work with more than two operands, the shorthand /
is by far the easiest way to do it.
40 / 2 / 5 /* Output: 4 */
divide(divide(40,2),5) /* Output: 4 */
40.divide(2).divide(5) /* Output: 4 */
Code language: JavaScript (javascript)
No – unlike addition and multiplication, which are commutative, division won’t work if you switch around the numbers you’re working with. 8/2 = 4
, but 2/8 = 0.25
.
The same applies when working with 3 or more operands.
100/2/4/2 = 6.25
, while (100/2)/(4/2) = 50/2 = 25
.
Example Database
The example database below shows the per-person split for several heists carried out by a certain crew of classy thieves. The simple Split formula shows the /
operator in action, and simply divides Total by Shares.
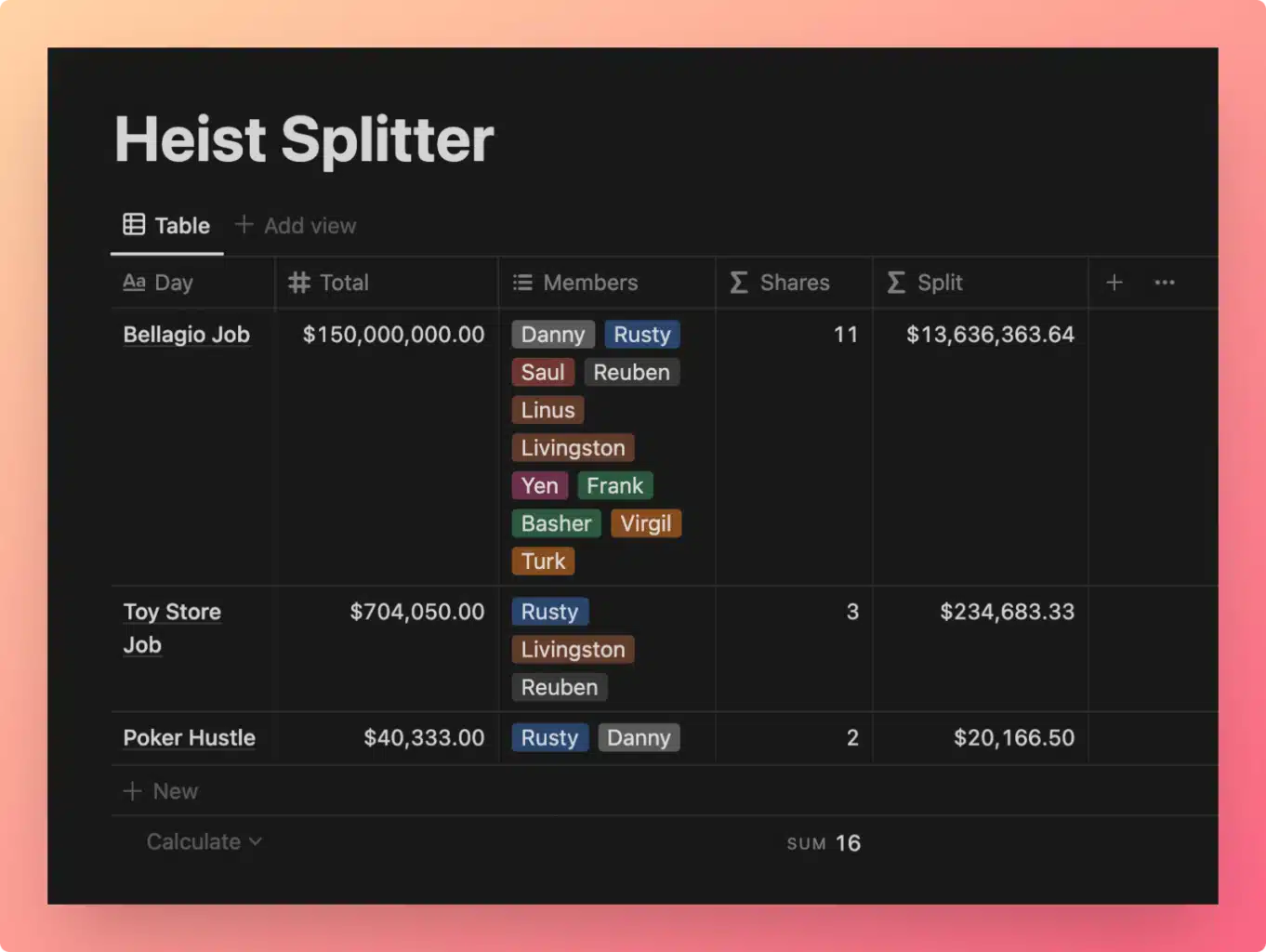
View and Duplicate Database
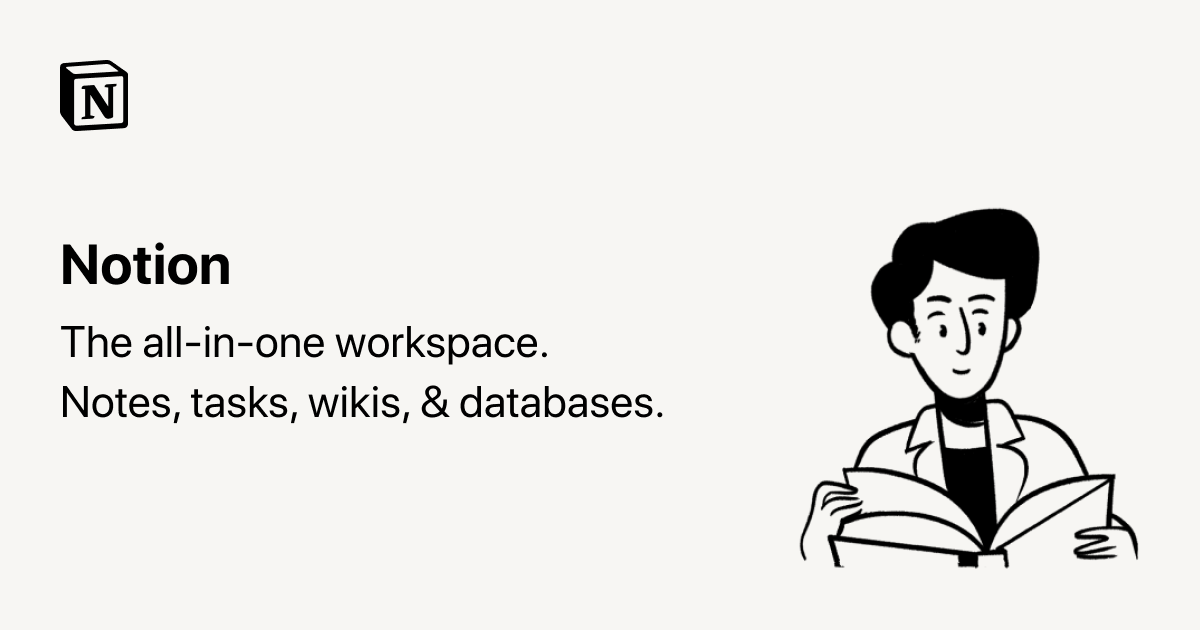
“Split” Property Formula
prop("Total") / prop("Members").length()
Code language: JavaScript (javascript)
Instead of using hard-coded numbers, I’ve called in each property using the prop()
function.