The test()
function allows you to test whether a string contains a substring, the latter of which can be a regular expression. If it does, the function returns true.
test(string, string [regex supported])
Code language: JavaScript (javascript)
test()
accepts two string arguments and outputs a Boolean result.
Good to know: test()
, replace, and replaceAll are able to automatically convert numbers and Booleans (but not dates) to strings. Manual type conversion is not needed.
Example Formulas
test("Monkey D. Luffy", "Luffy") // Output: true
// test() is case-sensitive
test("Monkey D. Luffy", "luffy") // Output: false
// You can use brackets [] to create a set of characters,
// any of which will be matched
test("Monkey D. luffy", "[Ll]uffy") // Output: true
// You can also create a group with () and then use the | (OR) operator
test("Monkey D. luffy", "(L|l)uffy") // Output: true
Code language: JavaScript (javascript)
Check out the regular expressions page to see a lot more that you can accomplish using them!
Example Database
This example database contains some file attachments. The Meta formula property displays information about each file, including its media type and hosting location (within Notion or hosted externally).
Good to know: This exact example is also used for the contains function. I recommend checking them both out to see how test()
is a much more flexible tool.
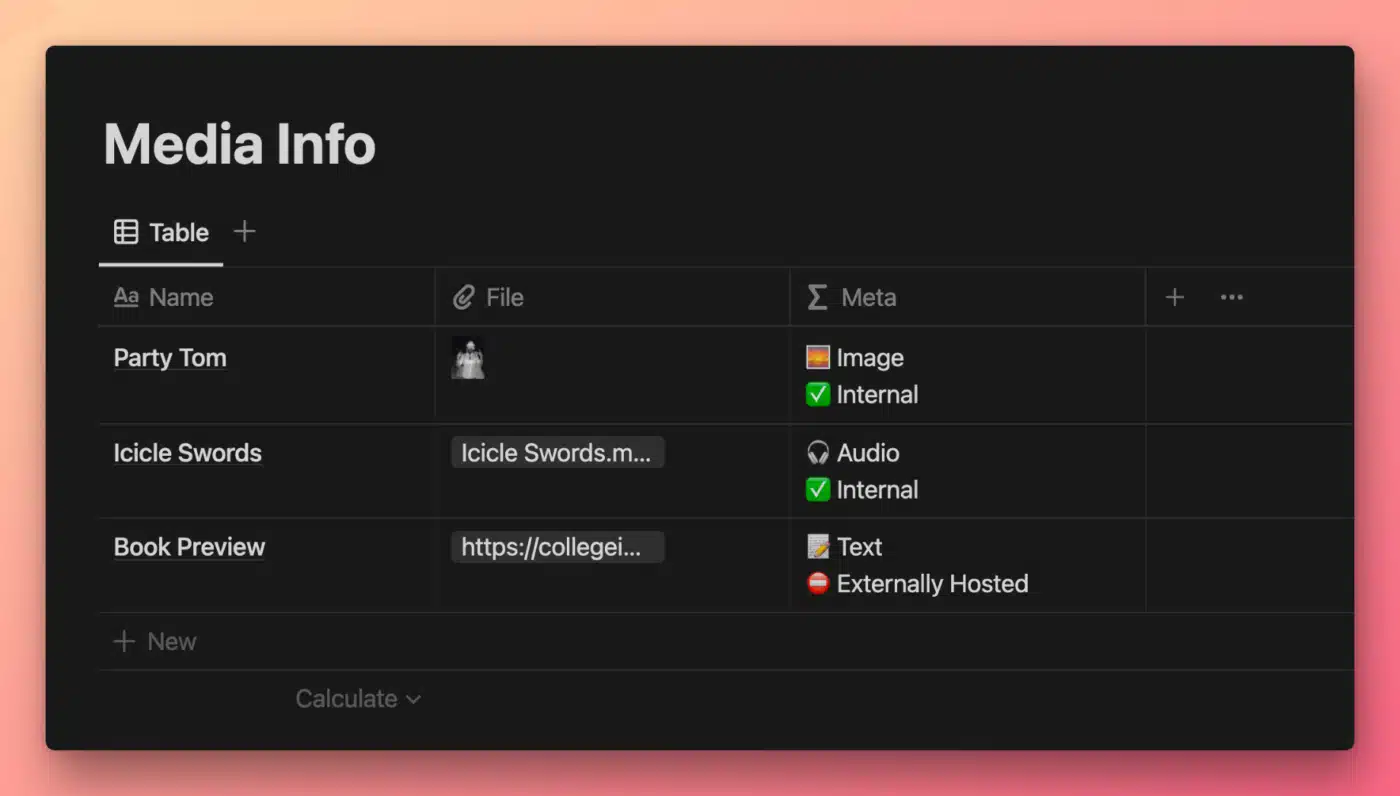
View and Duplicate Database
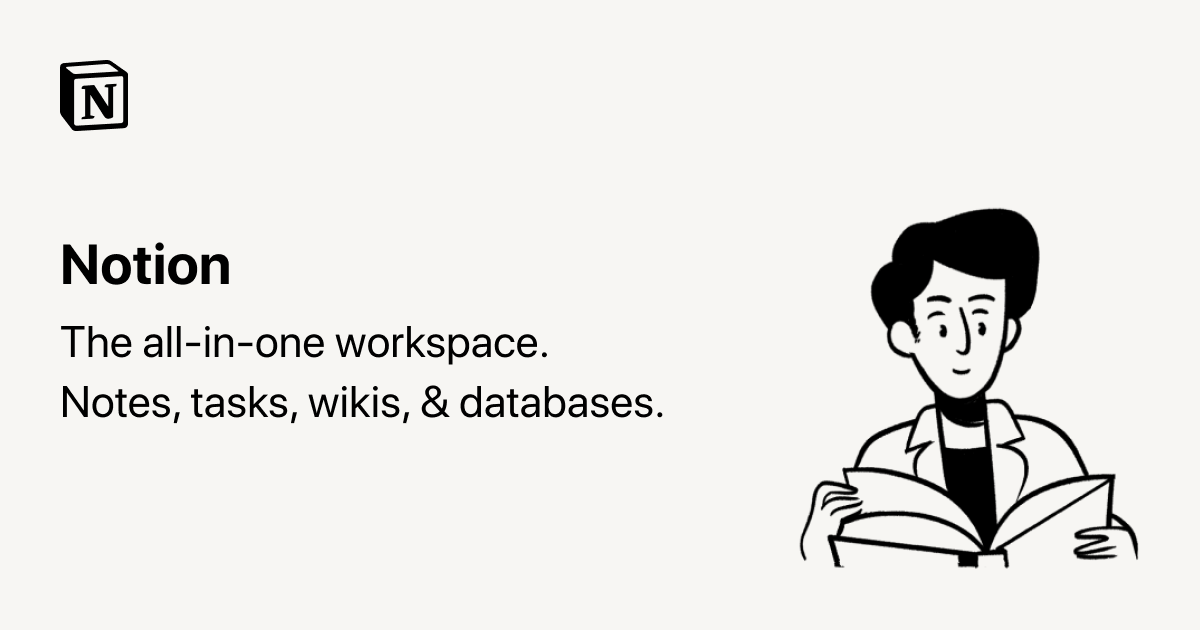
“Meta” Property Formula
// Compressed
if(test(prop("File"),"([jJ][pP][eE]?[gG]|[gG][iI][fF]|[pP][nN][gG])"),"🌅 Image",if(test(prop("File"),"([mM][pP]3|[wW][aA][vV]|[aA][iI][fF]{2})"),"🎧 Audio","📝 Text")) + "\n" + if(test(prop("File"),"secure.notion-static.com"),"✅ Internal","⛔️ Externally Hosted")
// Expanded
if(
test(
prop("File"),
"([jJ][pP][eE]?[gG]|[gG][iI][fF]|[pP][nN][gG])"
),
"🌅 Image",
if(
test(
prop("File"),
"([mM][pP]3|[wW][aA][vV]|[aA][iI][fF]{2})"
),
"🎧 Audio",
"📝 Text"
)
) +
"\n" +
if(
test(
prop("File"),
"secure.notion-static.com"
),
"✅ Internal",
"⛔️ Externally Hosted"
)
Code language: JavaScript (javascript)
test()
is essentially the same as the contains function, except it allows for the use of regular expressions.
This makes it far more powerful, and allows you to do things like case-insensitive matching. You can also include optional characters that don’t have to be included in the match.
Good to know: Regular expressions are incredibly powerful, but have a steep learning curve. Check out the regular expressions page for a primer on how to use them in Notion formulas, as well as resources for learning them more deeply.
This formula replicates the functionality of the example formula in the contains() function example database, but it uses test()
‘s regular expression capability to reduce the size of the formula and add case-insensitivity.
If you want to understand this formula fully, you should read the regular expressions page as well as the page on if statements.
But let’s break apart this small chunk of the formula here:
test(
prop("File"),
"([jJ][pP][eE]?[gG]|[gG][iI][fF]|[pP][nN][gG])"
)
Code language: JavaScript (javascript)
test()
returns true if the first string argument contains the substring specified in the second argument.
Here, our second argument is a regular expression. It is essentially saying:
Match “jpg”, “jpeg”, “gif”, or “png” – regardless of character case.
How is it doing that? Let’s break it down.
- The parentheses
()
form what’s called a capture group. - The pipe symbols
|
act as Boolean OR operators - The brackets
[]
essentially say, “Match any single character that is included here”.
To make all of this easier to understand, let’s start with the simplest version of a regular expression – a normal string.
"jpg"
Code language: JavaScript (javascript)
Here, test()
would only return true if the first argument’s string contains “jpg”.
But what if we want to match one of several options? We’ll need to use the OR operators |
for that, and they only work inside of a capture group created with parentheses ()
.
"(jpg|gif|png)"
Code language: JavaScript (javascript)
Now, our test()
function would return true if the first argument contains any of the following:
- jpg
- gif
- png
However, currently this would only happen if the substring was all lower-case. The substring “JPG” would not cause test()
to return true.
To make our regular expression case-insensitive, we’ll define a character class using brackets []
.
Here’s a very simple class:
"[Jj]"
Code language: JavaScript (javascript)
This will create a match if the first argument’s string contains “j” or “J”.
To make our entire set of potential file extensions case-insensitive, we simply need to define a similar character class for each letter:
"[Jj][Pp][Gg]|[Gg][Ii][Ff]|[Pp][Nn][Gg]"
Code language: JavaScript (javascript)
This regular expression will create a match for any of our three file extensions, regardless of the case (upper or lower) of any letter.
Good to know: Many programming languages and regex engines (such as JavaScript’s) support flags that you can set to more easily do things like marking your search as case-insensitive.
Unfortunately, in our testing we’ve found that Notion seems to support none of these flags. This is why we have to do things “the hard way” and define a character class for every letter that we want to be case-insensitive.
Finally, let’s deal with the fact that “jpg” is sometimes spelled “jpeg”. We could simply add another “or” case to our regular expression, but there’s a more efficient way to do it.
By adding a ?
character after another character (or character class), we can tell the regular expression engine (or “regex engine”) that character is optional. In other words, we’ll get a match if it shows up zero or one times.
To keep things simple, here’s how we’d match for jpg
or jpeg
, with case-sensitivity:
"jpe?g"
Code language: JavaScript (javascript)
Good to know: Since ?
is a special character, you need to “escape” it with a \
if you actually want to match for a question mark – e.g. \?
.
Now that you have that down, let’s add our ?
to the original regular expression:
"[Jj][Pp][Ee]?[Gg]|[Gg][Ii][Ff]|[Pp][Nn][Gg]"
Code language: JavaScript (javascript)
With [Ee]?
included, we’ll now match any version of “jpg” or “jpeg” regardless of any character’s case.
Making This Example Work in the Real World
This example deliberately keeps things simple in order to make the functionality of test()
easy to understand.
If you actually wanted to determine the media type of a file attachment, you’d first want to extract the file extension from its URL (otherwise, any other instance of letters such as “jpg” or “mov” might cause your formula to mis-identify the file type).
In the example database above, I’ve also included a File Extension property with the following formula:
replace(prop("File"), ".*\\.(\\w+)$", "$1")
Code language: JavaScript (javascript)
This uses the replace function along with another regular expression to replace the entire file URL with its file extension.
Want to understand how this regular expression works? Check out the replace function’s example database; I’ve explained it there.
To make this example error-free, you’d ultimately want to have the Meta property target the output of File Extension, rather than the file’s URL directly. Alternatively, you could combine these two formulas. I’ll leave that as an exercise for the reader.
Other formula components used in this example:
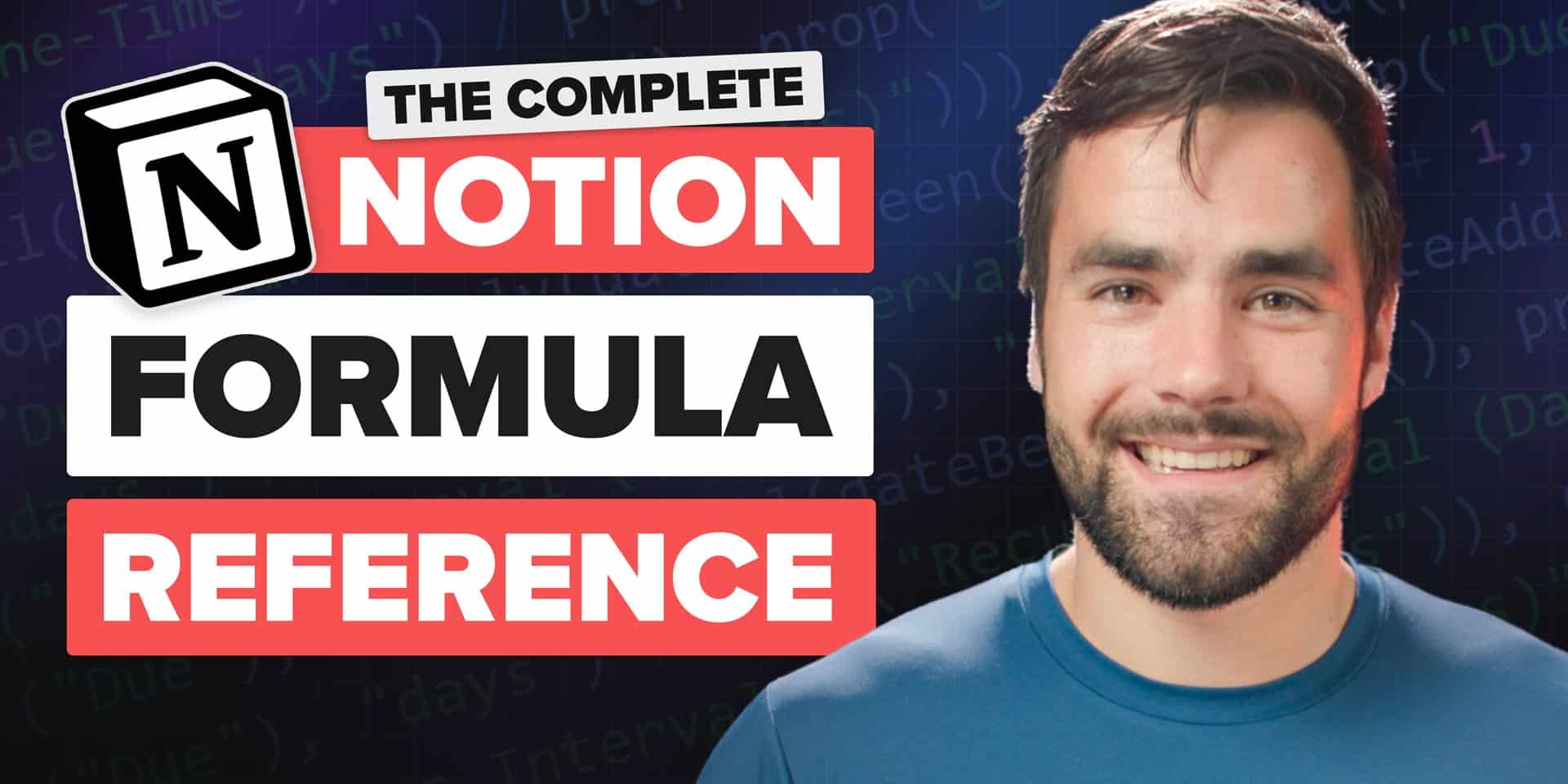
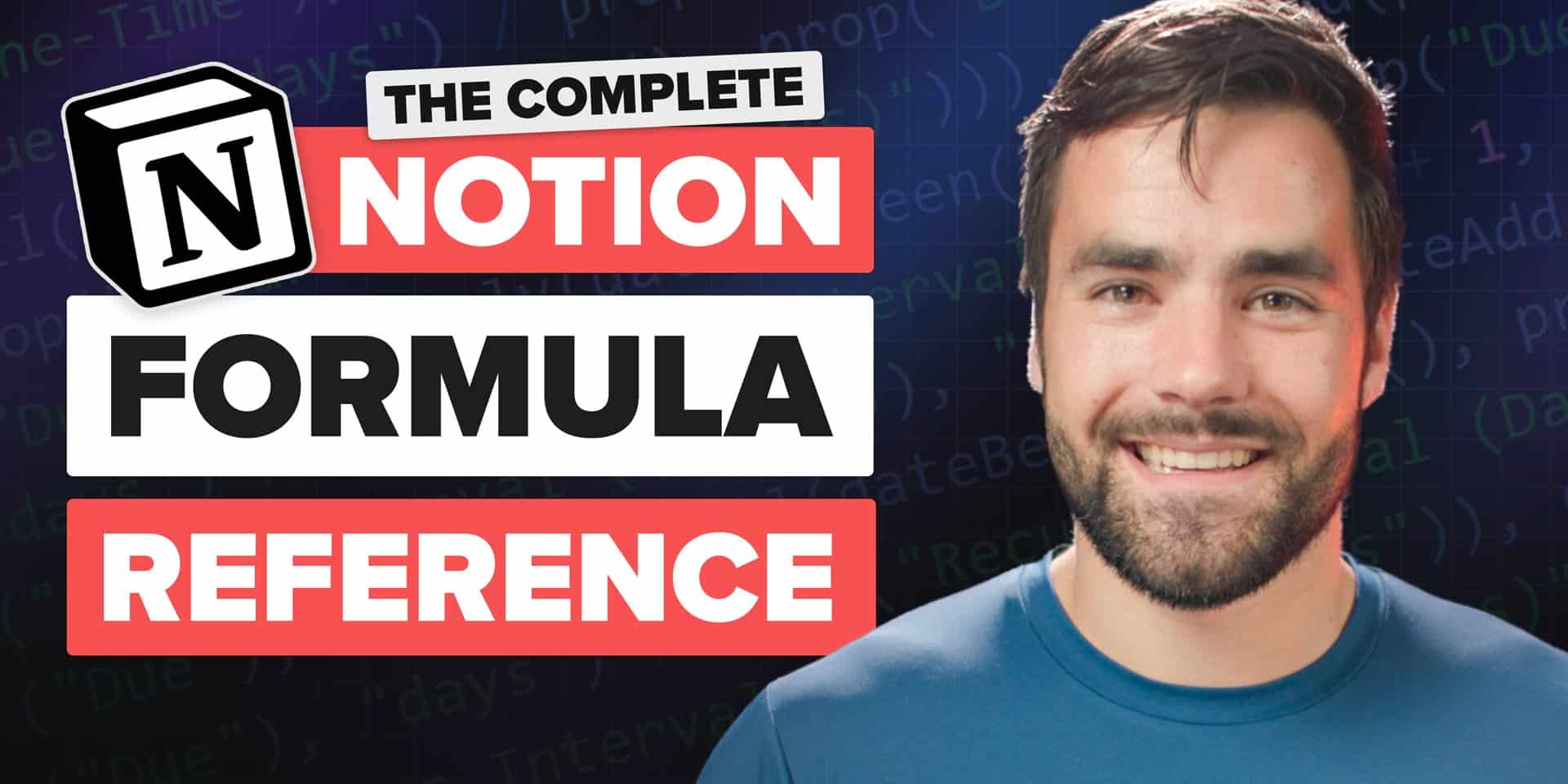