Notion supports the use of regular expressions in three functions:
- test – tests whether a string contains a regular expression. Outputs a Boolean true or false value.
- match — returns all matches of the regular expression as a list.
- replace – matches a single instance of a regular expression within a string and replaces it with a specified replacement string.
- replaceAll – matches all instances of a regular expression and replaces them with a specified replacement string.
By using regular expressions within these functions, it is possible to do many kinds of string manipulation within Notion formulas.
What Is a Regular Expression?
A regular expression (often called a regex) is simply a set of instructions that tells a regular expression engine how to search through an input string in order to find one or more matches.
Regular expressions can often look very complex:
replace("Bruce Thomas Wayne", "^[-\w]+\b\s?(.*)\b\s[-\w]+$", "$1")
/* Output: "Thomas" */
However, they can also be very simple. This is also a regular expression:
replace("My cat is cute", "cat", "dog")
/* Output: "My dog is cute" */
This expression would tell the regex engine to search the input string for a sub-string that matches “cat”. The replace function then replaces it with “dog”.
A simple match like this could also be found using the contains function:
contains("My cat is cute", "cat")
/* Output: true */
But what if you need to be more flexible with your search criteria?
Take this problem, for instance: Which of these strings contains the word “cat”?
- I have six cats.
- My dog catches fish.
- Cat food is expensive.
Here, contains()
runs into trouble (see this in an example database):
contains("I have six cats.", "cat") /* Output: true */
contains("My dog catches fish.", "cat") /* Output: true (should be false) */
contains("Cat food is expensive", "cat") /* false (should be true) */
contains()
gets the first one right, but fails on the other two.
On the second string, it sees “cat” inside the word “catches”. On the third string, it fails to see “Cats” because contains()
is case-sensitive.
This is where a regular expression can help us!
Regular expressions let us define character groups, make characters optional, check for word boundaries, and so much more.
Here’s how you could check all three of these strings correctly using the test function:
test("I have six cats.", "\b[Cc]ats?\b") /* true */
test("My dog catches fish.", "\b[Cc]ats?\b") /* false */
test("Cat food is expensive", "\b[Cc]ats?\b") /* true */
The regular expression we’re checking for here is \b[Cc]ats?\b
. Let’s break it down:
\b
is a special character that translates to “word boundary”. It’s not a space character; it’s the boundary between a word character and a non-word character. In Notion, word characters includeA-z
,0-9
, and_
.[Cc]
is a character class. The brackets[]
define a group of characters (C
andc
), and the regex engine will try to match any one of them. This allows us to check for both “Cat” and “cat”.?
denotes that the preceding character is optional. It can appear zero or one times in the match. Since thes
precedes it, this allows us to include the plural “cats” as well as the singular “cat”.
Breaking all this down to plain English, our regular expression is essentially saying:
Match any of the words “Cat, cat, Cats, or cats”.
Doing this with contains()
would be really inefficient. You’d need to string together many, many contains()
instances using or clauses in order to account for the many variables – not just plurality and capitalization, but word boundaries as well!
By giving us special characters to work with, regular expressions essentially give us a new language that we can use to define exactly what we’re looking for in the input string.
Once we’ve got our match (or matches), we can use Notion’s test, replace, and replaceAll functions to do incredibly useful things with them.
Learn Regular Expressions
This page isn’t intended to be a full tutorial on writing regular expressions. It’s a reference on how to use them in Notion formulas, and on what particular regex characters are supported in Notion.
If you’re interested in learning regular expressions, here are a few resources I recommend:
- RegexOne – start here. This is the best beginner tutorial you’ll find.
- Regular-Expressions.info – a gold mine of regular expression tutorials and explanations.
- RexEgg – another fantastic general resource for learning regex.
- .Net Regular Expressions Quick Reference – intended for .NET developers, but still quite handy as a general resource.
- Regex101 – a tool for building and testing regular expressions.
Supported Regular Expression Characters
This section includes one or more examples for every regular expression feature supported within Notion’s formula editor.
See working examples for all of these features here:
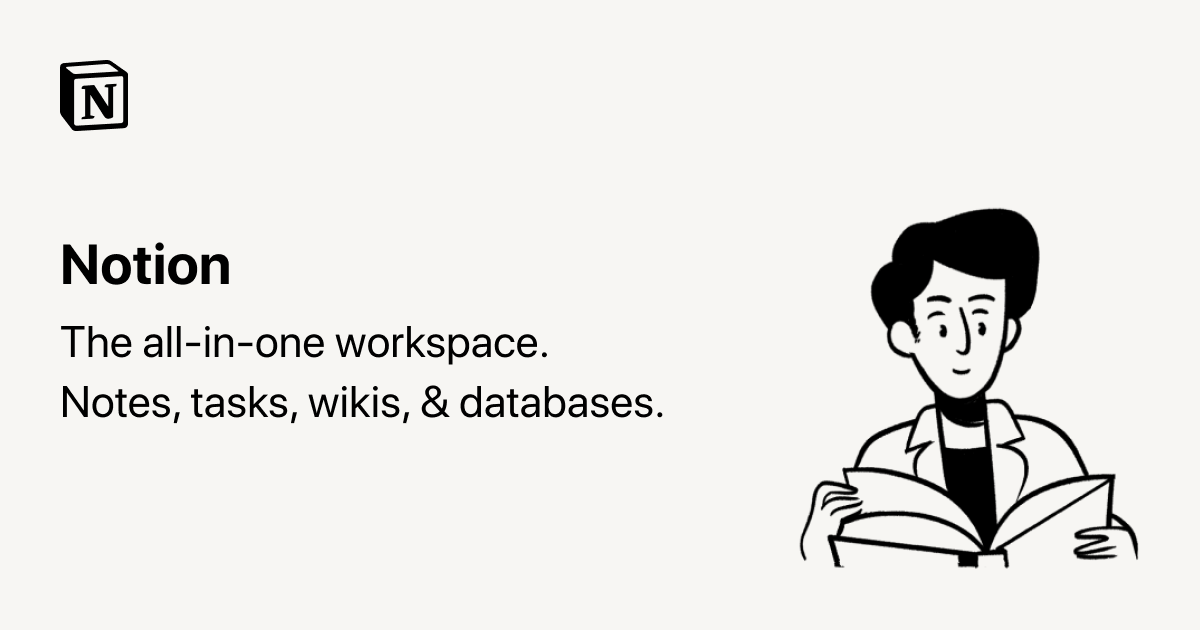
Character Escapes
Working examples:
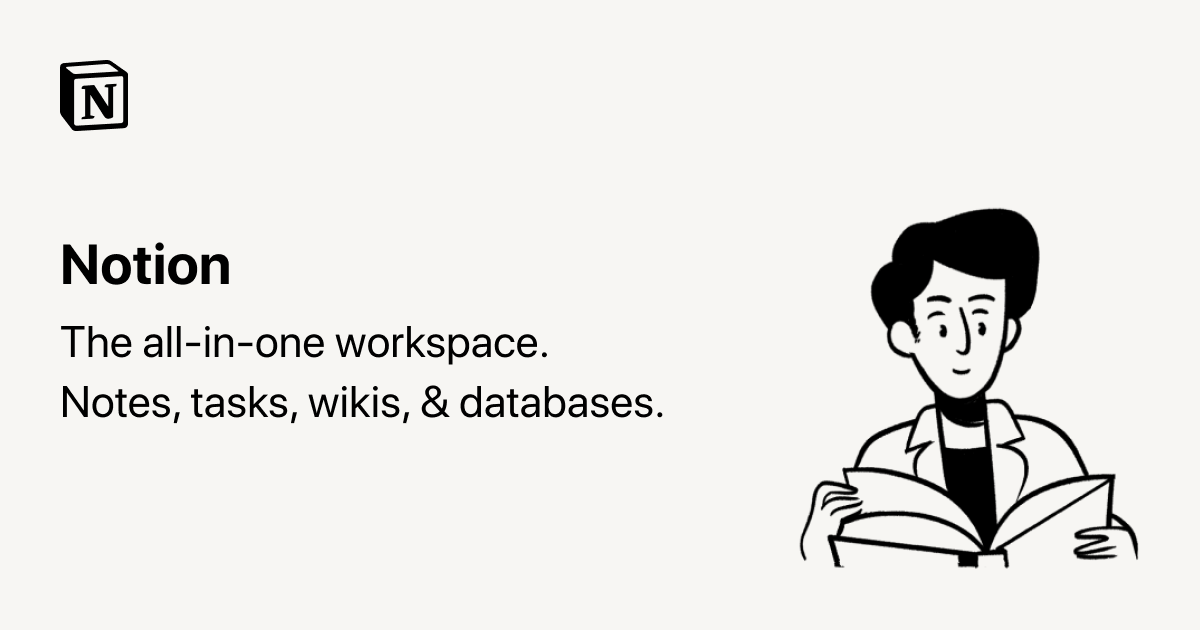
\u0000
– escaped Unicode reference
test("A", "\u0041") /* Output: true */
\000
– octal character reference
Note: Only works in the regular expression argument. Doesn’t work in the input string or replacement string arguments.
test("A", "\101") /* Output: true */
\x00
– hexadecimal character reference
Note: Only works in the regular expression argument. Doesn’t work in the input string or replacement string arguments.
test("A", "\x41") /* Output: true */
You can find a list of all Unicode, octal, and hexadecimal reference codes here:
\n
– new line
replaceAll("Apple\nBanana\nOrange", "\n", "\n\n")
/* Output:
"Apple
Banana
Orange" */
There are also several characters that must escaped with single backslashes (\
) in order to be represented normally. These characters are used as special characters within regular expressions if they are not escaped.
Character | Escape |
---|---|
Period — . | \. |
Question mark — ? | \? |
Dollar sign — $ | \$ |
Asterisk — * | \* |
Plus sign — + | \+ |
Caret — ^ | \^ |
Left parenthesis — ( | \( |
Right parenthesis — ) | \) |
Left bracket — [ | \[ |
Right bracket — ] | \] |
Left curly brace — { | \{ |
Right curly brace — } | \} |
Pipe — | | \| |
Forward slash — / | \/ |
Backslash — \ | \\ |
Double quotations — " | \" |
Character Classes
Learn about character classes:
Working examples:
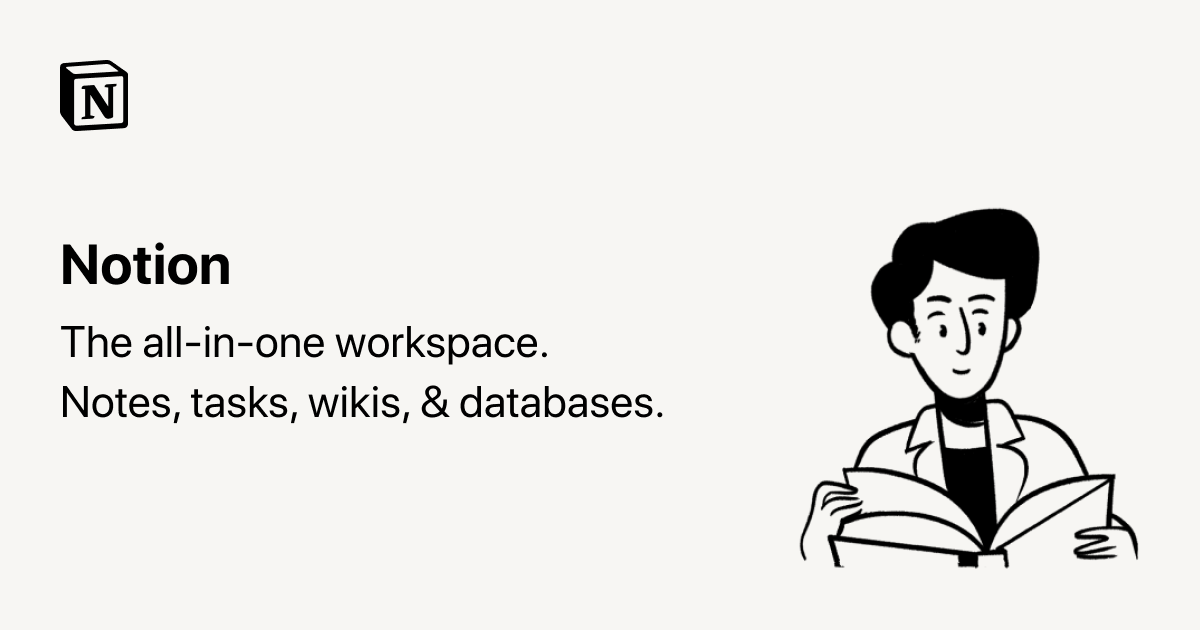
\w
– alphanumeric character
Notion considers non-spacing marks to be non-alphanumeric characters. Other regex engines (.NET, for example) do the opposite.
Lowercase letters | a-z |
Uppercase letters | A-Z |
Numbers | 0-9 |
Punctuation, Connector symbols | Notion only supports _ |
replaceAll("CAPS_nocap 12345", "\w", "*")
/* Output: "********** *****" */
\W
– non-alphanumeric character
replaceAll("correct horse battery staple", "\W", "")
/* Output: "correcthorsebatterystaple" */
\d
– digit character (0-9)
replaceAll(id(), "\d", "")
/* Output: "ccadaecddeabaeeb" (where ID is ccad6aec4dd34e5a942334bae3e9b728) */
\D
– non-digit character
replaceAll(id(), "\D", "")
/* Output: "6434594233439728" (where ID is ccad6aec4dd34e5a942334bae3e9b728) */
\s
– whitespace character
replaceAll("charmander man bun", "\sman\s", " ")
/* Output: "charmander bun" */
\S
– non-whitespace character
replaceAll("charmander man bun", "\Sman\S", "ndl")
/* Output: "chandler man bun" */
.
– wildcard; matches any single character except newline (\n)
replaceAll("And blimey, if it ain't mutton again today!", ".", "😡")
/* Output: "😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡" */
/* Include newlines using (.|\n)
Assume prop "TwoLines" contains:
"And blimey,
if it ain't
mutton again today!" */
replaceAll(prop("TwoLines"), "(.|\n)", "😡")
/* Output: "😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡😡" */
[]
– character class (matches any single character included in the group)
Character classes support ranges such as A-Z
(all uppercase character), A-z
(all upper and lowercase characters), and 0-9
(all digits).
Commas may also be used to visually separate ranges and characters in your expression, but they are not needed.
replaceAll("gold fold bold", "[gfb]", "t")
/* Output: "told told told" */
replaceAll("27 dresses", "[a-z]", "👗")
/* Output: "27 👗👗👗👗👗👗👗" */
replaceAll("abcdefghijklmnopqrstuvwxyz123456789", "[a-ev-z1357-9]", "🙄")
/* With commas: */
replaceAll("abcdefghijklmnopqrstuvwxyz123456789", "[a-e,v-z,1,3,5,7-9]", "🙄")
/* Output: "🙄🙄🙄🙄🙄fghijklmnopqrstu🙄🙄🙄🙄🙄🙄2🙄4🙄6🙄🙄🙄" */
Character class subtraction is not supported.
[^]
– negated character class (matches any single character not included in the group)
replaceAll("123456789abcdefghijklmnopqrstuvwxyz", "[^a-z]", "")
/* Output: "abcdefghijklmnopqrstuvwxyz" */
replaceAll("abcdefghijklmnopqrstuvwxyz123456789", "[^cow]", "")
/* Output: cow ("c", "o", "w" are in alphabetical order naturally. The character class doesn't specify order.) */
Quantifiers
Learn about quantifiers:
Working examples:
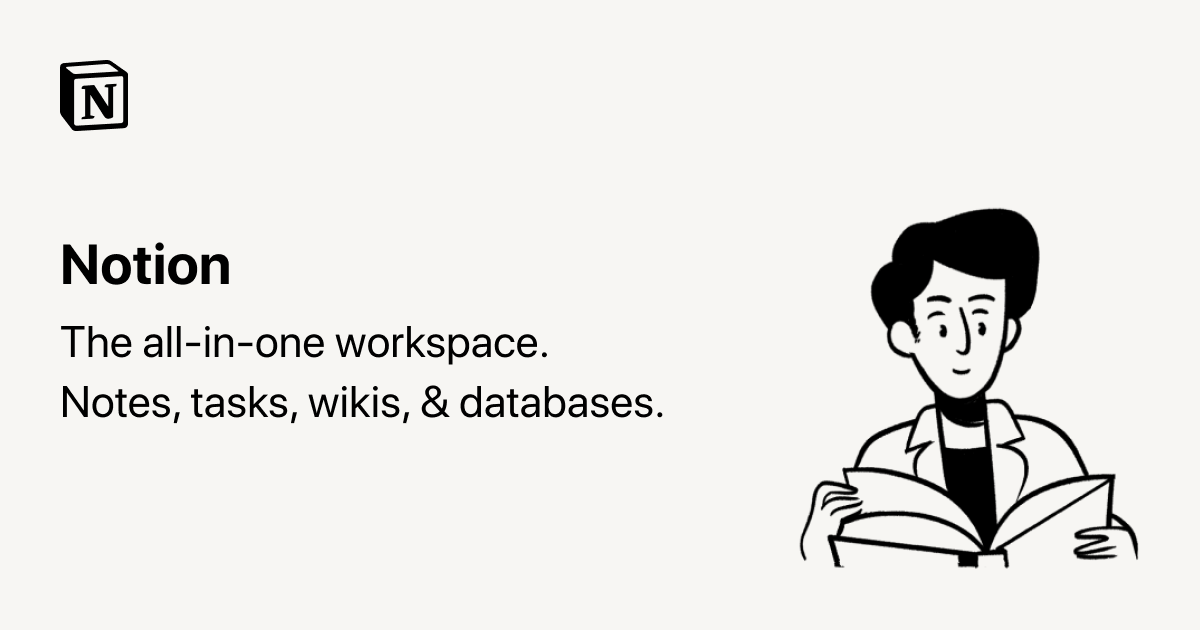
*
– match zero or more of the preceding element
replaceAll("Trs Tres Trees Treeeeees", "Tre*s", "🌳")
/* Output: "🌳 🌳 🌳 🌳" */
replaceAll("Trs Tres Trees Treees Treeees", "Tr(ee)*s", "🌳")
/* Output: "🌳 Tres 🌳 Treees 🌳" */
+
– match one or more of the preceding element
replaceAll("Trs Tres Trees Treeeeees", "Tre+s", "🌳")
/* Output: "Trs 🌳 🌳 🌳" */
replaceAll("Trs Tres Trees Treees Treeees", "Tr(ee)+s", "🌳")
/* Output: "Trs Tres 🌳 Treees" 🌳 */
?
– preceding element is optional; match it zero or one times
replaceAll("Trs Tres Trees Treeeeees", "Tre?s", "🌳")
/* Output: "🌳 🌳 Trees Treeeeees" */
replaceAll("Trs Tres Trees Treees Treeees","Tr(ee)?s","🌳")
/* Output: "🌳 Tres 🌳 Treees Treeees" */
??
– match preceding element zero or one times (as few times as possible)
replaceAll("Trs Tres Trees Treees Treeees", "Tre??s", "🌳")
/* Output: "🌳 🌳 Trees Treees Treeees" */
replaceAll("Trs Tres Trees Treees Treeees", "Tr(ee)??s", "🌳")
/* Output: "🌳 Tres 🌳 Treees Treeees" */
+?
– match preceding element one or more times (as few times as possible)
replace("Tree", "Tre+?", "🌳")
/* Output: "🌳e" */
*?
– match preceding element zero or more times (as few times as possible)
replace("Heeeeeeelp", "H.*?", "*")
/* Output: "*eeeeeeelp" */
replace("Heeeeeeelp", "H.*?l", "*")
/* Output: "*p" */
{n}
– match the preceding element n times
replace("Heeeeeeelp", "e{7}", "*")
/* Output: "H*lp" */
{n,}
– match the preceding element n or more times
replace("Heeeeeeelp", "e{1,}", "*")
/* Output: "H*lp" */
{n,m}
– match the preceding element between n and m times (inclusive)
replace("Heeeeeeelp", "e{1,6}", "*")
/* Output: "H*elp" */
{n}?
– match the preceding element n times (no difference from {n}
replace("Heeeeeeelp", "e{7}?", "*")
/* Output: "H*lp" */
{n,}?
– match the preceding element at least n times, but as few times as possible
replace("Heeeeeeelp", "e{1,}?", "*")
/* Output: "H*eeeeeelp" */
{n,m}?
– match the preceding element at least n times, no more than m times, and as few times as possible
replace("Heeeeeeelp", "e{1,6}?", "*")
/* Output: "H*eeeeeelp" */
Anchors
Learn about anchors:
Working examples:
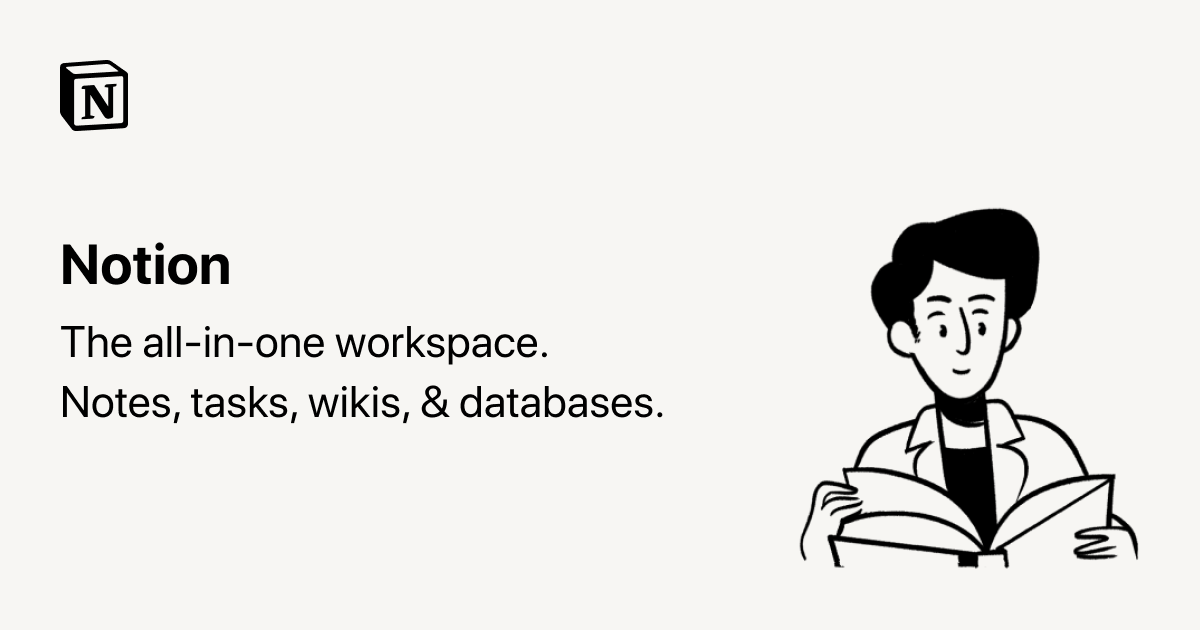
^
– start of line
replace("dogs dogs dogs", "^dogs", "cats")
/* Output: "cats dogs dogs" */
$
– end of line
replace("dogs dogs dogs", "dogs$", "cats")
/* Output: "dogs dogs cats" */
\b
– word boundary
This is not a space (that’s \s
). This is the boundary between a word character and a non-word character (including punctuation such as ,
, .
, ;
, etc.). It is a zero-length match.
replaceAll("martini art artist", "\bart", "!!!")
/* Output: "martini !!! !!!ist" */
replaceAll("martini art artist", "\bart\b", "!!!")
/* Output: martini !!! artist */
\B
– not on a word boundary
replaceAll("martini art artist", "\Bart", "!!!")
/* Output: "m!!!ini art artist" */
Character Grouping
Learn about character grouping and capturing:
Working examples:
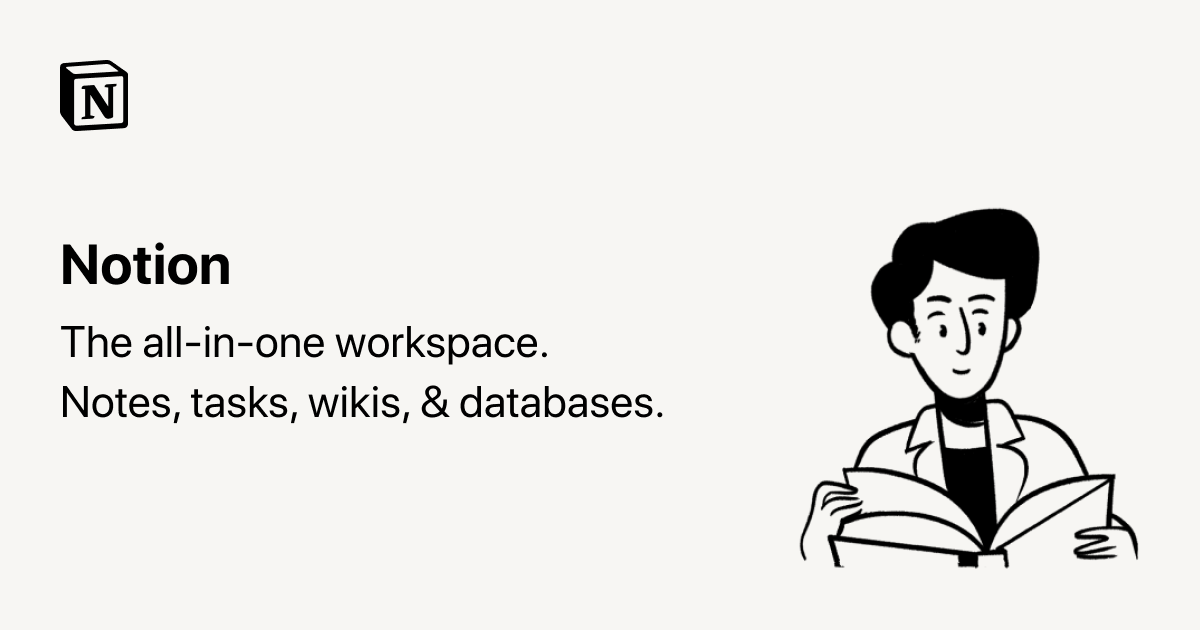
()
– capture group (is automatically assigned a sequential reference number)
replace("Dog Blog", "(Dog) Blog", "$1")
/* Output: "Dog" */
replace("Dog Blog", "(Dog) (Blog)", "$2")
/* Output: "Blog" */
replaceAll("Jack Sparrow", "^(\w+)\b.*", "$1")
/* Output: "Jack" */
(?<name>expression)
– named capture group
replace("Jack plays poker", "(?<sup>J).*(oker)", "$<sup>$2")
/* Output: "Joker" */
/* Named capture groups are still assigned their sequential number. Note the output when I reference "$<sup>$1" instead of "$<sup>$2" */
replace("Jack plays poker", "(?<sup>J).*(oker)", "$<sup>$1")
/* Output: "JJ" */
(?:)
– non-capturing group
replace("Jack", "(?:Jack)", "$1")
/* Output: "$1" */
replace("123", "(\w)(?:\w)(\w)", "$2")
/* Output: "3" */
Substitutions
Learn about substitutions:
Working examples:
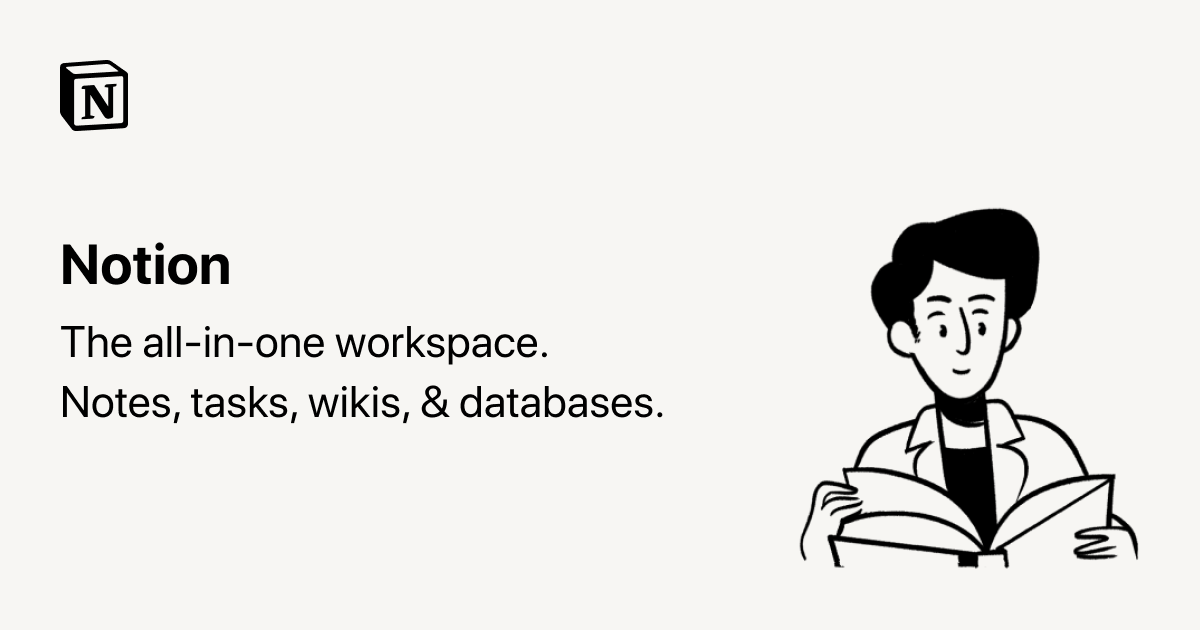
$n
– capture group numbers
replace("Dog Blog", "(Dog) Blog", "$1")
/* Output: "Dog" */
replace("Dog Blog", "(Dog) (Blog)", "$2")
/* Output: "Blog" */
$&
– copy of the whole match
replaceAll("Hello", ".*", "$& $& $&")
/* Output: "Hello Hello Hello" */
replaceAll("I sell pan and pan accessories", "pan", "pro$&e")
/* Output: "I sell propane and propane accessories" */
$`
– copy of the entire input string before the match
replace("badboy","boy","$`")
/* Output: "badbad" */
$'
– copy of the entire input string after the match
replace("badboy", "bad", "$'")
/* Output: "boyboy" */
Backreferences
Learn about backreferences:
Working examples:
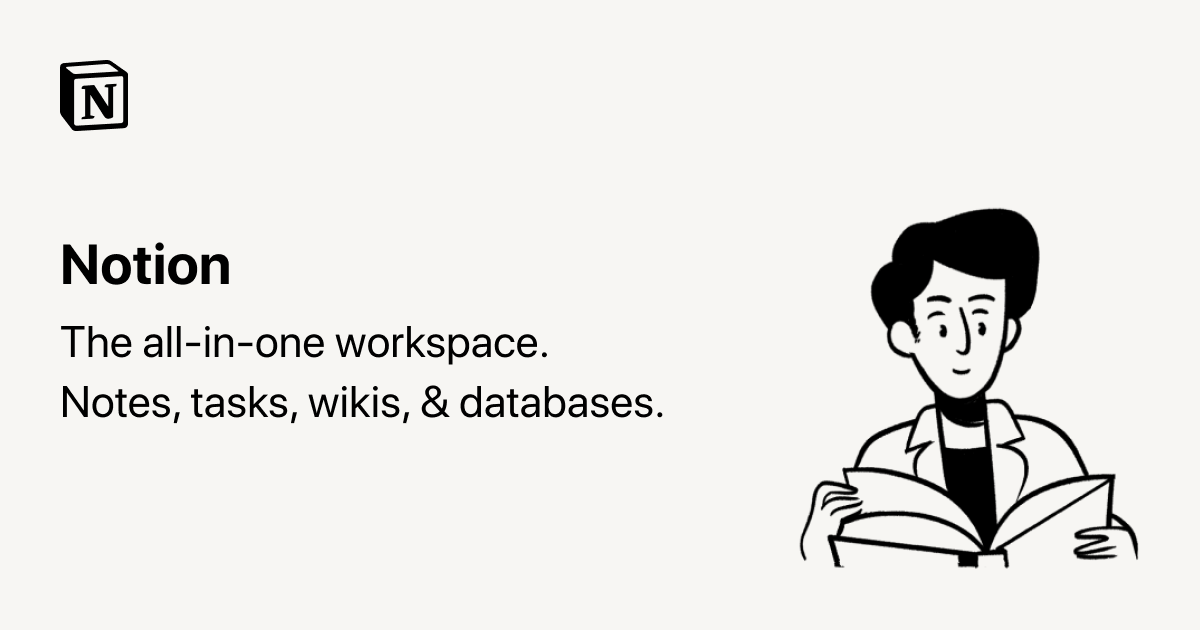
\n
– e.g. \1
– backreference. Must match an existing capture group
Note that the backreference looks for matches of the content of its capture group. It is not an alias for the expression within the capture group.
replace("12-12-12", "([0-9]+)-\1-\1", "Success")
/* Output: "Success" */
replace("12-34-56", "([0-9]+)-\1-\1", "Success")
/* Output: "12-34-56" */
replace("I have 56 apples, 35 bananas, and 35 grapes.", ".*(56).*(35).*\2.*", "Success")
/ Output: "Success" */
(?<name>\w) \k<name>
– named backreference
replace("I have 56 apples, 35 bananas, and 35 grapes.", ".*(56).*(?<two>35).*\k<two>.*", "Success")
/* Output: "Success" */
/* Named backreferences can still be called with their sequential number */
replace("I have 56 apples, 35 bananas, and 35 grapes.", ".*(56).*(?<two>35).*\2.*", "Success")
/* Output: "Success" */
Alternation
Learn about alternations:
Working examples:
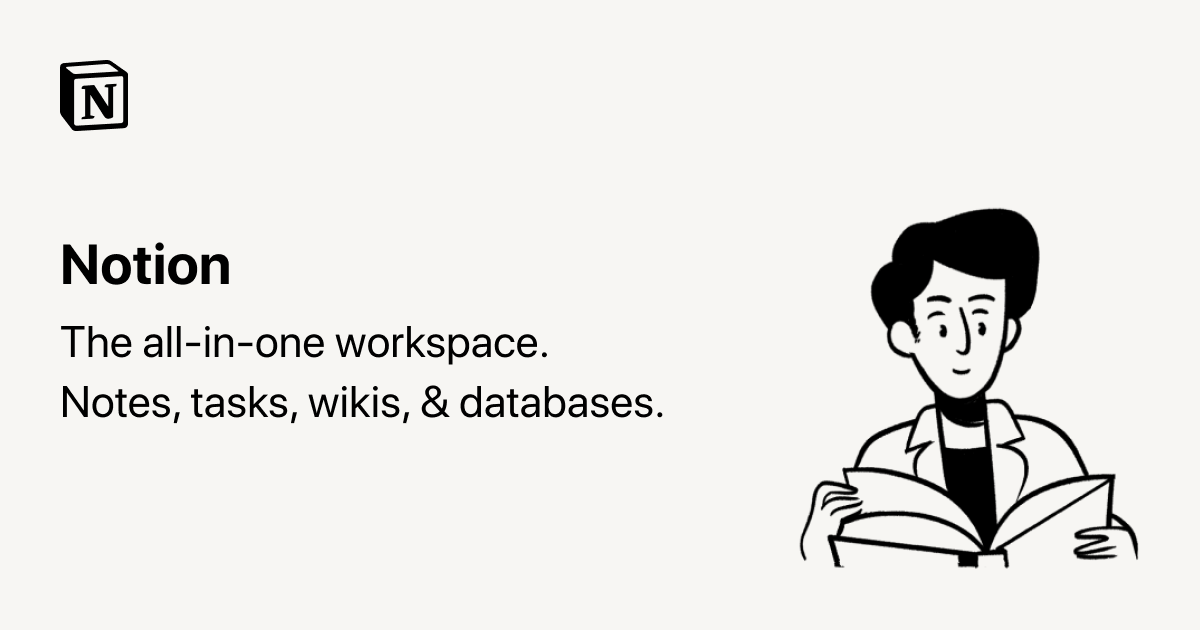
|
– either/or
replaceAll("jpg, jpeg, png, gif, wav", "jpg|jpeg|png|gif", "picture")
/* Output: "picture, picture, picture, picture, wav" */
/* Order matters! */
replace("mould", "ou|o", "😀")
/* Output: "m😀ld" */
replace("mould", "o|ou", "😀")
/* Output: "m😀uld" */
/* Alternation can also be done inside groups: */
replaceAll("My name is Bruce Wayne", "(Bruce|Wayne)", "*****")
/* Output: "My name is ***** *****" */
Unsupported Features
The following features are currently not supported in Notion’s flavor of regex:
\A
\z
\Z
\p{name}
\P{name}
$+
$_
(?>*subexpression*)
(?(expression) yes | no)
- Lookarounds are not fully supported due to lack of support in all variants of Safari. Not recommended to use them in your formulas.
- Flags/modifiers are not supported in Notion at all (which often makes case-insensitive matching very tedious).
Unicode Numbers in Regular Expressions
When writing regular expressions in Notion formulas, it is possible to “hard code” Unicode numbers into your expression. The regex engine will then parse these as their actual Unicode characters. (Thanks to Ben Borowski for pointing this out to me).
To do so, use double-backslashes \\
to escape the Unicode reference:
/* \u0027 escapes apostrophes. */
/* \u2018 and \u2019 handle left and right single quotes. */
replaceAll("Mike 'Iron Mike' Tyson", "[\u0027\u2018\u2019]","🥊")
/* Output: "Mike 🥊Iron Mike🥊 Tyson" */
See a working example of this:
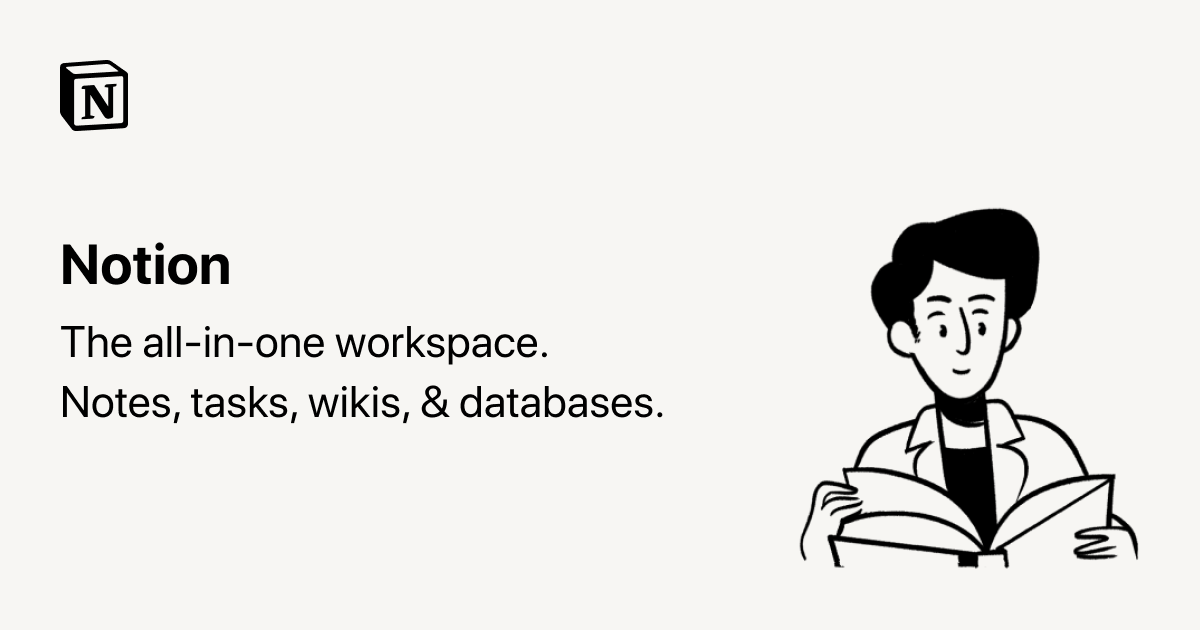
It is also possible to use octal or hex codes here. For example:
test("A", "\x41") /* Output: true */
test("A", "\101") /* Output: true */
These \
Unicode references don’t work in input string argument, nor the replacement argument within replace and replaceAll. They’ll only be interpreted correctly within the regular expression argument.
Escaping Double Quotations
You can usually escape a double quotation "
in a Notion formula using a single backslash \
:
"Mike \"Iron Mike\" Tyson"
/* Output: "Mike "Iron Mike" Tyson" */
This also works in the input-string and replacement arguments within Notion’s regular expression functions:
replace("Mike \"Iron Mike\" Tyson", "^(\w+)\b", "\"$1\"")
/* Output: ""Mike" "Iron Mike" Tyson" */
However, this does not work inside of regular expressions – i.e., the second argument of the test, replace, and replaceAll functions.
Fortunately, you can get around this by hard-coding their Unicode character codes into your expression:
\u0022
for a normal quotation mark\u201c
for a left double quotation mark\u201d
for a right double quotation mark- For example, here’s how you could extract
"Iron Mike"
fromMike "Iron Mike" Tyson
:
replace("Mike \"Iron Mike\" Tyson", ".*([\u0022\u201c\u201d][^\u0022\u201c\u201d]+[\u0022\u201c\u201d]).*", "$1")
/* Output: ""Iron Mike"" */
See a working example of this:
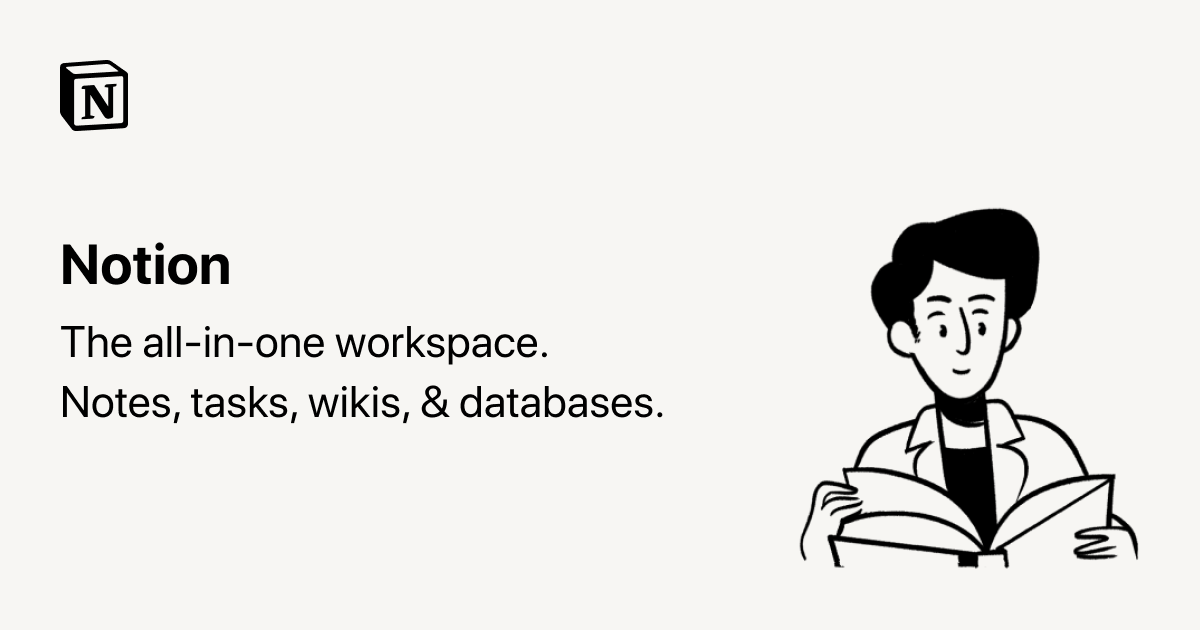
It’s best to ensure your character class includes all three common quotation marks: [\u0022\u201c\u201d]
When you type a quotation mark directly into the formula editor, you’ll get a normal quotation mark "
– however, when you type within text fields inside a Notion database, Notion intelligently uses left “
and right ”
quotation marks to wrap your text.
In the example above, I hard-coded Mike \"Iron Mike\" Tyson
within the formula editor. However, if that string had been pulled in via another property (e.g. prop("Name")
), then it would likely be using left “
and right ”
quotation marks.