The String data type holds and represents text content. Strings can hold nearly any character by default.
You can create a string by wrapping characters within quotation marks "
. This includes numbers, “true/false”, etc. If you wrap characters in quotation marks, you’ll create a string.
"Monkey D. Luffy"
"42"
"true"
Code language: JavaScript (javascript)
Strings can be concatenated, i.e. combined. You can do so with the join function and with the add operator +:
"Monkey D. Luffy" + " will be " + "King of the Pirates!"
/* Output: Monkey D. Luffy will be King of the Pirates! */
join(["Monkey D. Luffy", "will be", "King of the Pirates!"], " ")
/* Output: Monkey D. Luffy will be King of the Pirates! */
Code language: JavaScript (javascript)
You cannot perform mathematical operations on strings. Notion does not do string-to-number conversion, so you’ll need to convert strings to numbers manually.
If a string contains nothing but a number, you can convert it using the toNumber function:
/* Invalid: Will throw an error */
add(2, "2")
/* Valid */
add(2, toNumber("2"))
Code language: JavaScript (javascript)
Comparing Strings
You can compare strings using the equal ==
and unequal !=
operators and related functions.
Good to know: Equal and unequal operators test for strict equality. In JavaScript, this would be done with the ===
operator.
"Monkey" == "Monkey" /* Output: true */
"Monkey" == "monkey" /* Output: false (comparison is case-sensitive) */
"Goku" != "Vegeta" /* Output: true */
Code language: JavaScript (javascript)
Comparing Strings to Other Data Types
You can now compare strings to non-string values.
"1" == 1 /* Output: false */
"true" != true /* Output: true */
Code language: JavaScript (javascript)
Escaping Characters
Some special characters must be escaped with the backslash character \
in order to represented property in a Notion formula.
Character | Escape Sequence |
---|---|
Double Quote " | \" |
Backslash \ | \\ |
Newline | \n |
Tab | \t |
Important notes:
- Single quotes
'
do not need to be escaped. If you wrap a string in single quotes, you’ll get an invalid character error.
Here’s a reference database that contains all of these escaped characters, which you can duplicate:
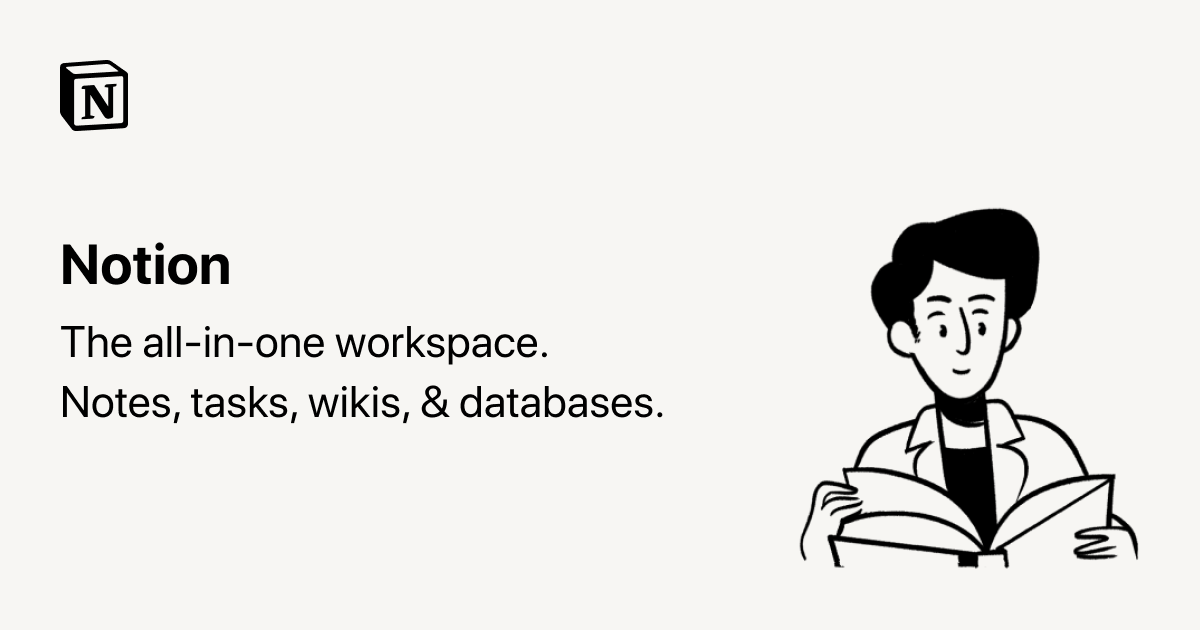