The or operator returns true if either one of its operands is true. It accepts Boolean operands.
Boolean or Boolean
Boolean || Boolean
or(Boolean, Boolean)
Code language: JavaScript (javascript)
Good to know: Notion is no longer picky, so ||
, OR
, and Or
now also work.
You can also use the function version, or()
.
Example Formulas
true or false /* Output: true */
false || true /* Output: true */
false or false /* Output: false */
10 > 20 or "Cat" == "Cat" /* Output: true */
Code language: JavaScript (javascript)
The or
operator can also be chained together multiple times:
10 > 20 or "Cat" == "Dog" or true /* Output: true */
Code language: JavaScript (javascript)
Example Database
This example database records the results of several Rock, Paper, Scissors matches. The Winner formula declares the winner of each match.
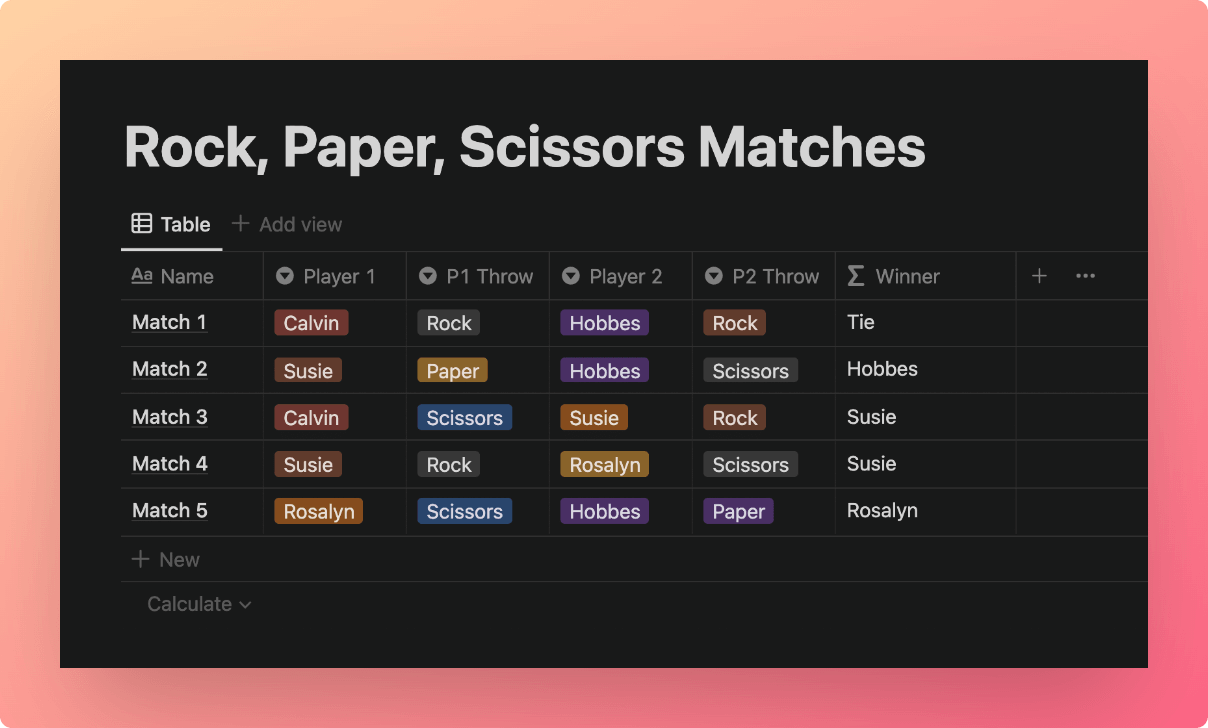
View and Duplicate Database
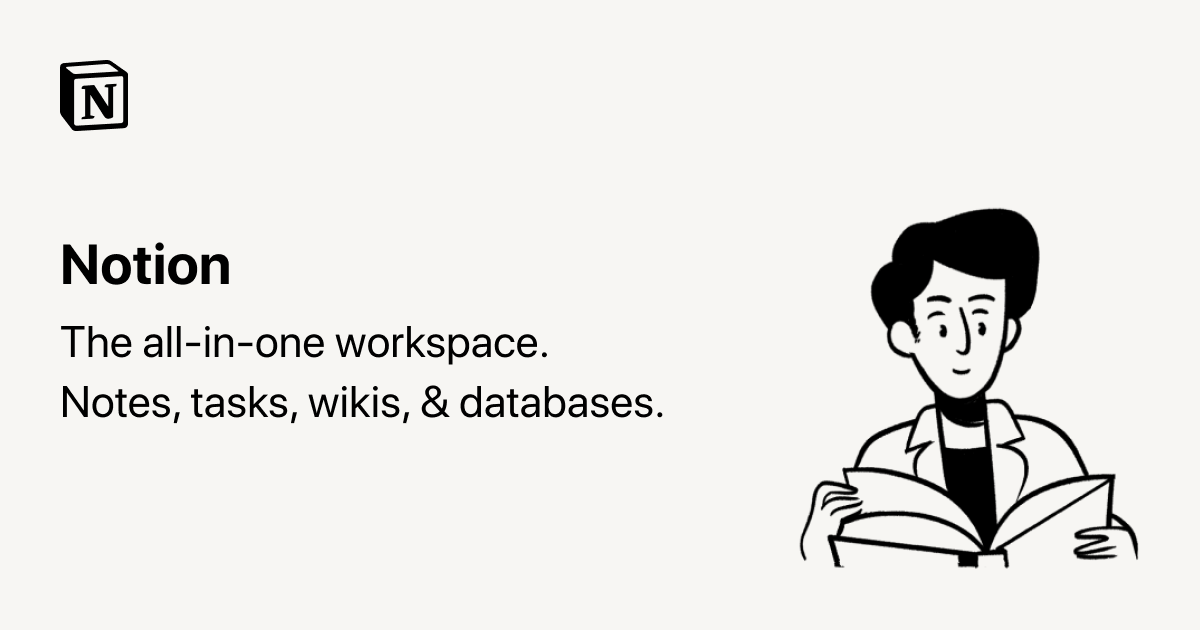
“Winner” Property Formula
(prop("P1 Throw") == prop("P2 Throw")) ? "Tie" :
( (prop("P1 Throw") == "Rock" and prop("P2 Throw") == "Scissors" or
prop("P1 Throw") == "Paper" and prop("P2 Throw") == "Rock" or
prop("P1 Throw") == "Scissors" and prop("P2 Throw") == "Paper") ?
prop("Player 1") :
prop("Player 2"))
/* ifs() Version */
ifs(
prop("P1 Throw") == prop("P2 Throw"),
"Tie",
prop("P1 Throw") == "Rock" and prop("P2 Throw") == "Scissors"
or prop("P1 Throw") == "Paper" and prop("P2 Throw") == "Rock"
or prop("P1 Throw") == "Scissors" and prop("P2 Throw") == "Paper",
prop("Player 1"),
prop("Player 2")
)
Code language: JavaScript (javascript)
The formula consists of a nested if-then statement (written with the conditional operators ?
and :
). It first checks to see if the players threw the same choice, and returns “Tie” if so. If not, it uses the or
and and
operators to determine the winner.
Since the win/lose value of a throw is determined by the other throw, we use a series of three statements to compare Player 1’s throw against Player 2’s.
Good to know: This example also shows how or
has a lower operator precedence than and
.
Other formula components used in this example:
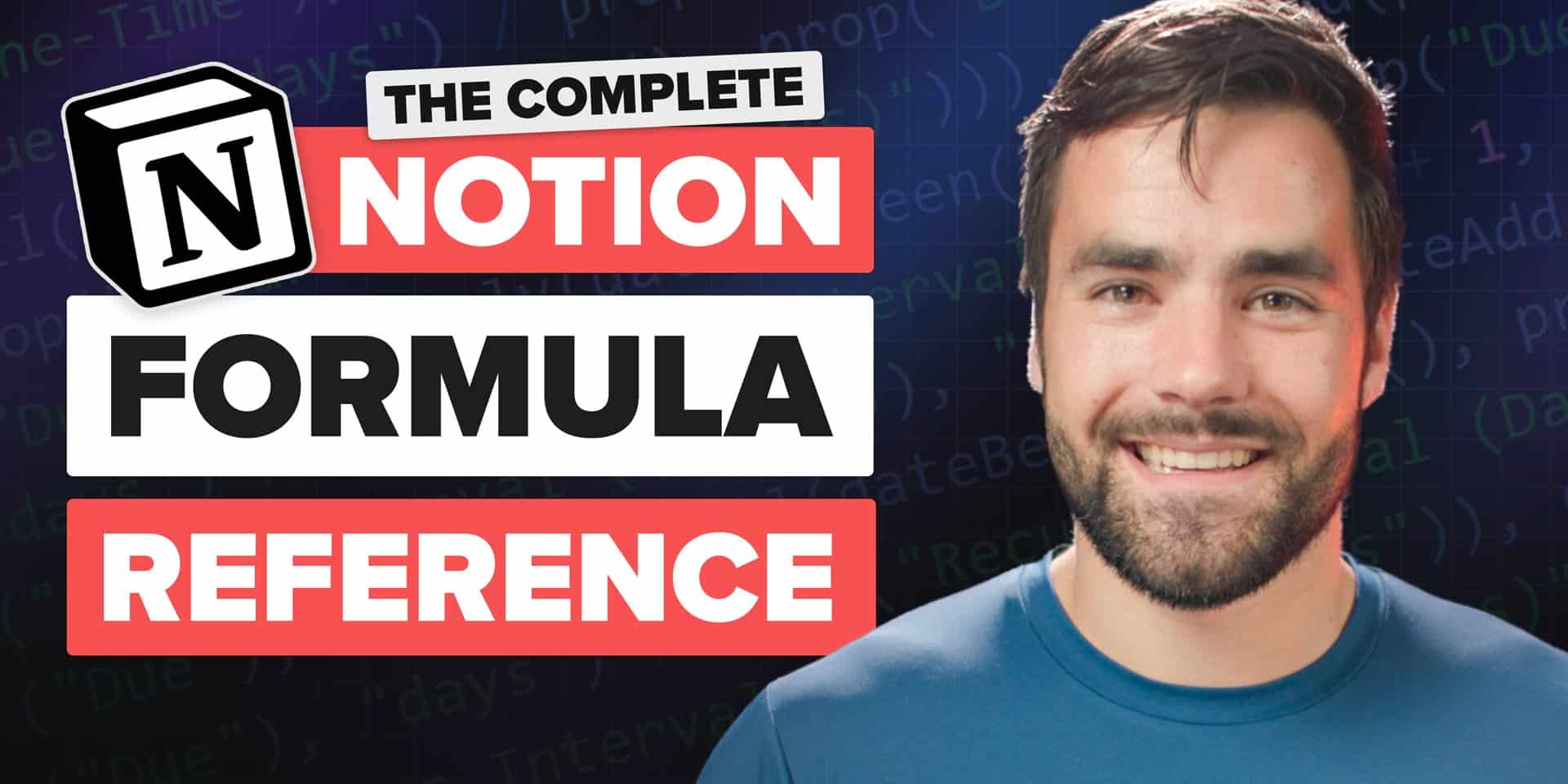
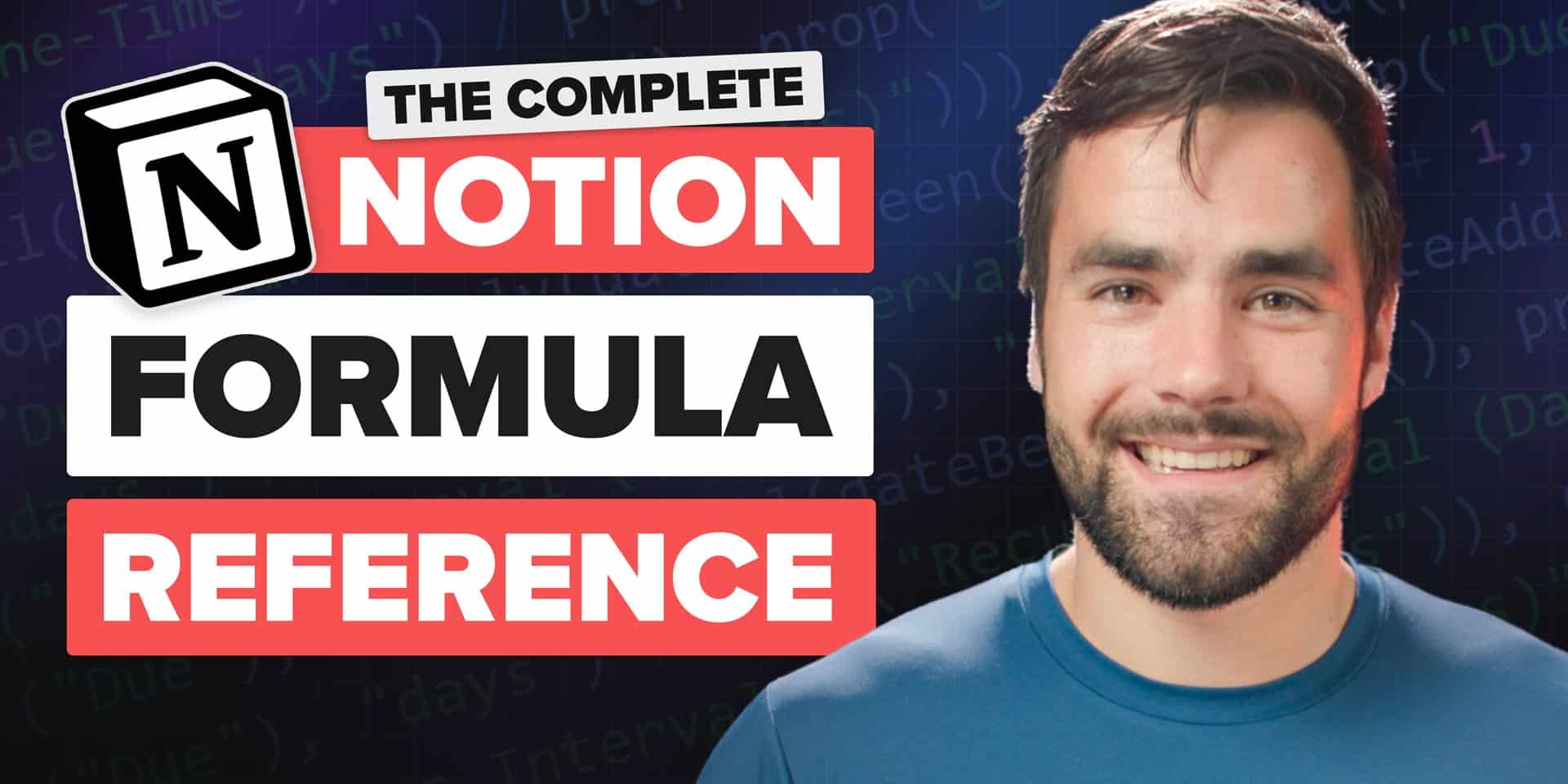
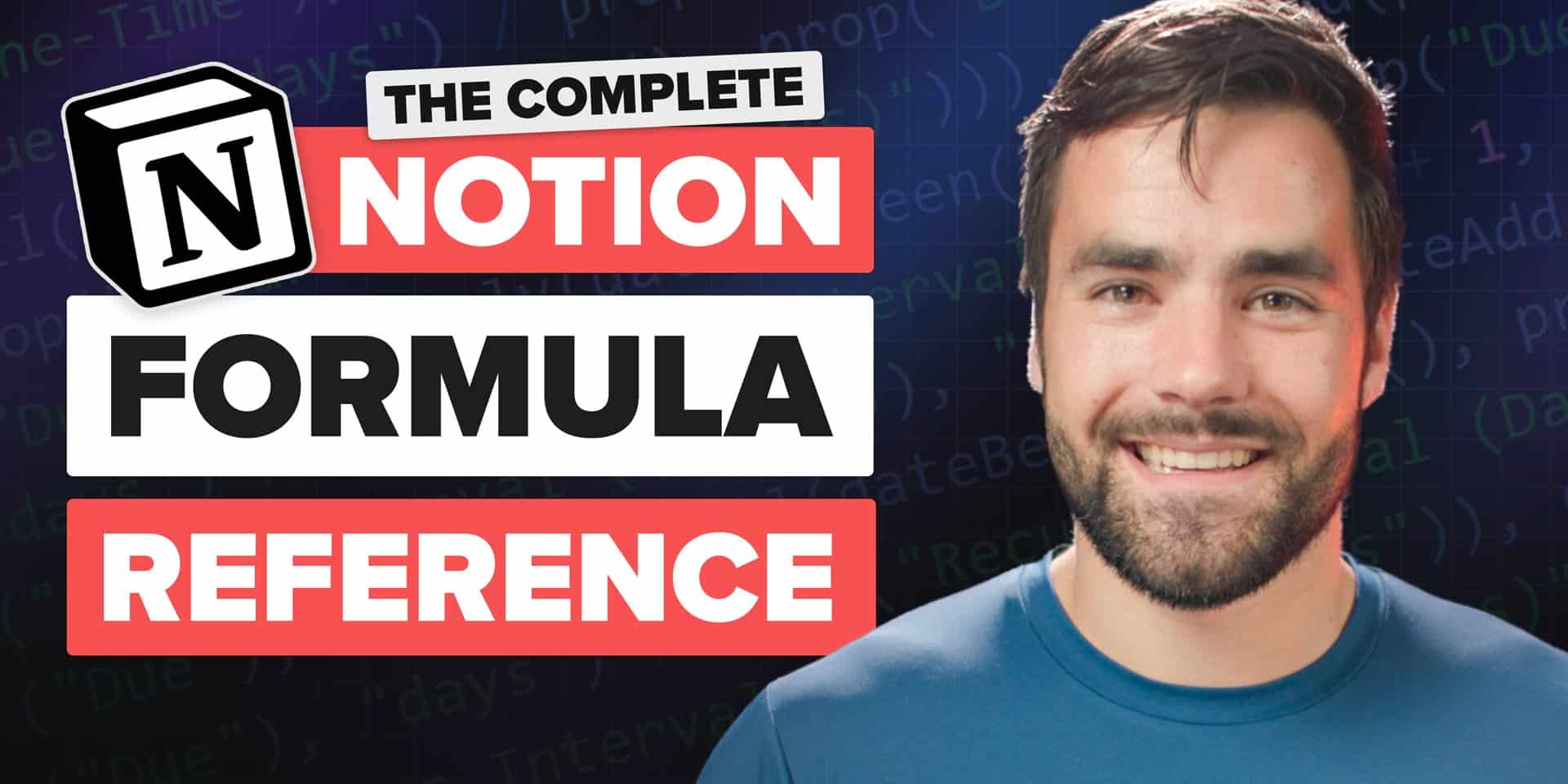