Notion operators have a specific operator precedence order. Operators with a higher precedence (10 being the highest) will have their logic executed before operators of lower precedence.
Operators at the same precedence level are executed either left-to-right or right-to-left, depending on their associativity.
Refer to the table below to see the precedence level and associativity of each operator.
Precedence | Operator Name | Associativity | Symbol |
---|---|---|---|
10 | Parentheses | N/A | () |
9 | Not | right-to-left | not |
8 | Exponentiation (pow) | right-to-left | ^ |
7 | multiply, divide | left-to-right | * , / |
6 | add, subtract | left-to-right | + , - |
5 | Greater than, Greater than or equal, Less than, Less than or equal | N/A | > , >= , < , <= |
4 | unequal, equal | N/A | != , == |
3 | And | left-to-right | and |
2 | Or | left-to-right | or |
1 | Conditional (if) | right-to-left | ... ? ... : ... |
Since parentheses ()
have the highest precedence in a Notion formula, you can use them to define a specific order of operations within any formula.
It’s also good to understand that associativity can be thought of as an implied default setting of parentheses. For example:
/* Multiply has left-to-right associativity. The following are equivalent: */
2 * 3 * 4 /* Output: 24 */
(2 * 3) * 4 /* Output: 24 */
/* Exponentiation has right-to-left associativity (the "Tower Rule"). The following are equivalent: */
2 ^ 3 ^ 2 /* Output: 512 */
2 ^ (3 ^ 2) /* Output: 512 */
Code language: JavaScript (javascript)
Certain operators cannot be chained in Notion formulas; thus, they do not have associativity. These are marked with “N/A” in the associativity column.
For example, the following formulas will not work in Notion:
/* Invalid */
1 > prop("Number") > 5
/* Valid */
1 > prop("Number") and prop("Number") < 5 /* Output: true */
---
/* Invalid */
1 == toNumber(true) == toNumber("1")
/* Valid */
1 == toNumber(true) and 1 == toNumber("1") /* Output: True */
Code language: JavaScript (javascript)
If you want to understand operator precedence and associativity more thoroughly, I’ll recommend reading through the Operator Precedence guide for JavaScript on MDN’s web docs:
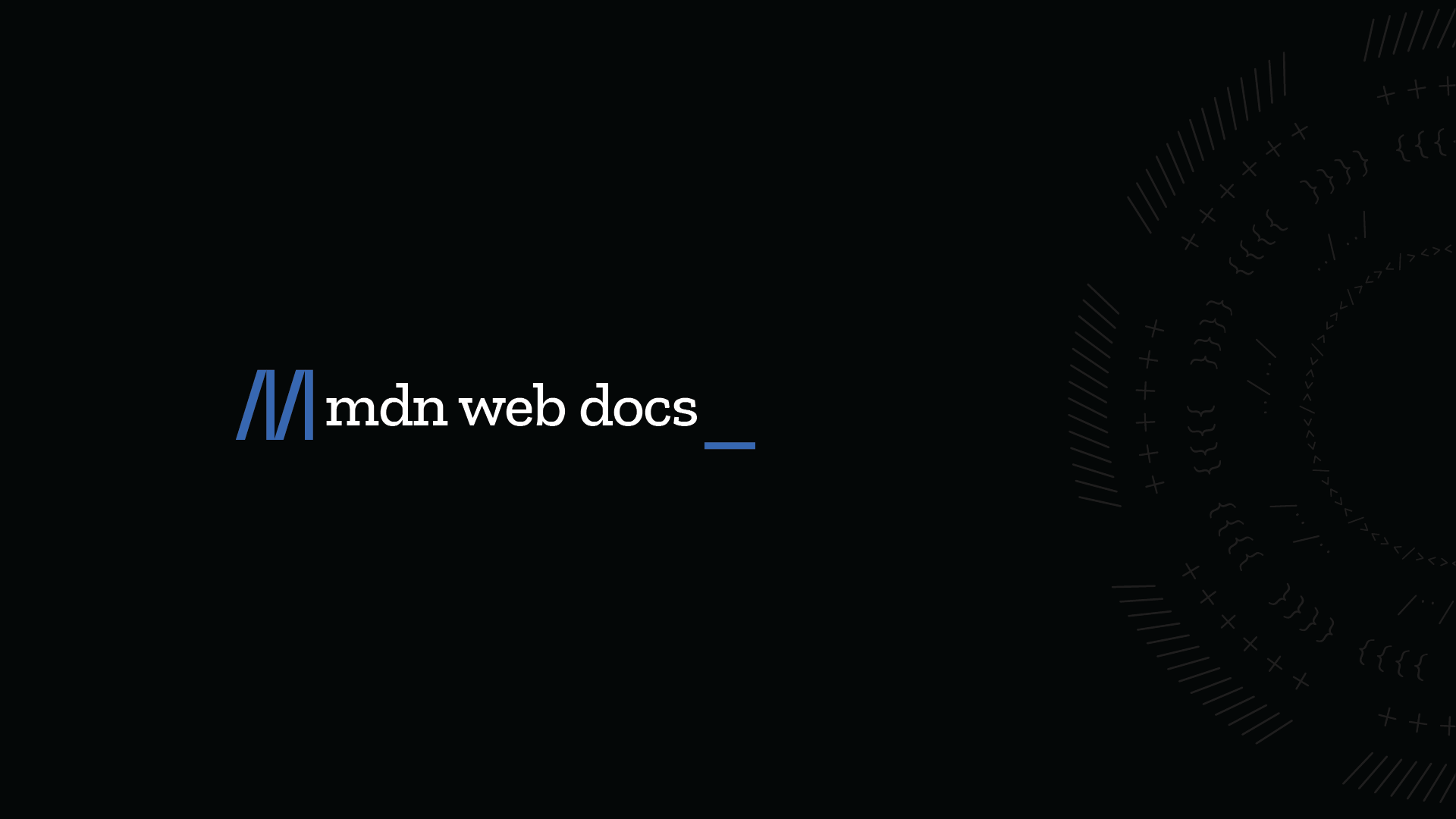