The formatDate()
function formats a date as a string using various formatting tokens.
formatDate(date, string [must conform to token format], timezone [optional])
date.formatDate(string [must conform to token format], timezone [optional])
Code language: JavaScript (javascript)
It accepts three arguments in the following order:
- A date (can be passed via properties – Date, Created Time, Last Edited Time – or via date functions such as now, fromTimestamp, dateAdd, dateSubtract)
- A string specifying the formatting tokens for the date output
- (Optional) A string specifying a timezone for the date.
By default, formatDate()
will display dates in your system’s timezone, even if the date with the Date property was created from another timezone or explicitly given that timezone. (This matches how Date properties also display dates and times; a date with a time of 02:30
in PST
will show as 05:30
to a user located in the EST
time zone. When they click into the Date property, they’ll see the actual 02:30
time with the timezone displayed).
If you want, you can specify another timezone, which will cause the function to display the date in that timezone.
formatDate(prop("Date"), "YYYY-MM-DD HH:mm", "EST")
Code language: JavaScript (javascript)
You can use timezone formats such as America/Chicago
, EST
, GMT+1
, UTC-5
, and any other format supported by the Luxon date library.
Example Formulas
formatDate(now(), "MMMM DD YYYY") /* Output: June 24 2022 */
now().formatDate("dddd, MMMM DD, YYYY hh:mm A zz")
/* Output: Friday, June 24, 2022 10:45 AM MDT */
Code language: JavaScript (javascript)
You can also use brackets ([]
) to escape any characters that you’d like to render explicitly (i.e. not use a formatting command):
formatDate(now(), "[Month of] MMMM, YYYY")
/* Output: Month of June, 2022 */
Code language: JavaScript (javascript)
By specifying the formatting options you want within the function’s second argument, you can customize your date format.
Notion uses Luxon to handle date formatting, but retains a lot of the tokens used by Luxon’s predecessor, Moment. Refer to the token lists below to see all possible formatting options.
Limitations
formatDate()
returns a string, not a date. Therefore, it is not possible to do date math on its output. You can however use parseDate and a date formatted as YYYY-MM-DD to do this.
Good to Remember: Notion formulas are picky about data types. The formula editor will almost never do automatic type conversion, so you must pass the correct data types when you use functions.
For example, you cannot use dateAdd or dateSubtract on the output of formatDate()
:
/* ERROR: Argument of type text does not satisfy function dateAdd. */
dateAdd(formatDate(now(),"MMMM DD YYYY"), 4, "months")
/* Using parseDate */
dateAdd(parseDate("2023-09-07"), 4, "months")
/* Output: January 7, 2024 */
Code language: JavaScript (javascript)
It is also very difficult to perform date comparisons using the output of formatDate()
. While equality comparisons work well:
formatDate(now(), "MMM DD YYYY") == formatDate(prop("Date"), "MMM DD YYYY")
Code language: JavaScript (javascript)
…it is much more difficult to perform “earlier than” or “later than” comparisons.
Therefore, it is recommended to use functions such as timestamp, date, week, month, year, etc. to make these kinds of comparisons.
It is also not possible to create date-based filters in a database view using a formula property that outputs a date string via formatDate()
.
However, it is possible to work with the output of formatDate()
using the Notion API.
For an example, see this recurring tasks tutorial that uses Make.com and the Notion API to parse the output of formatDate()
:
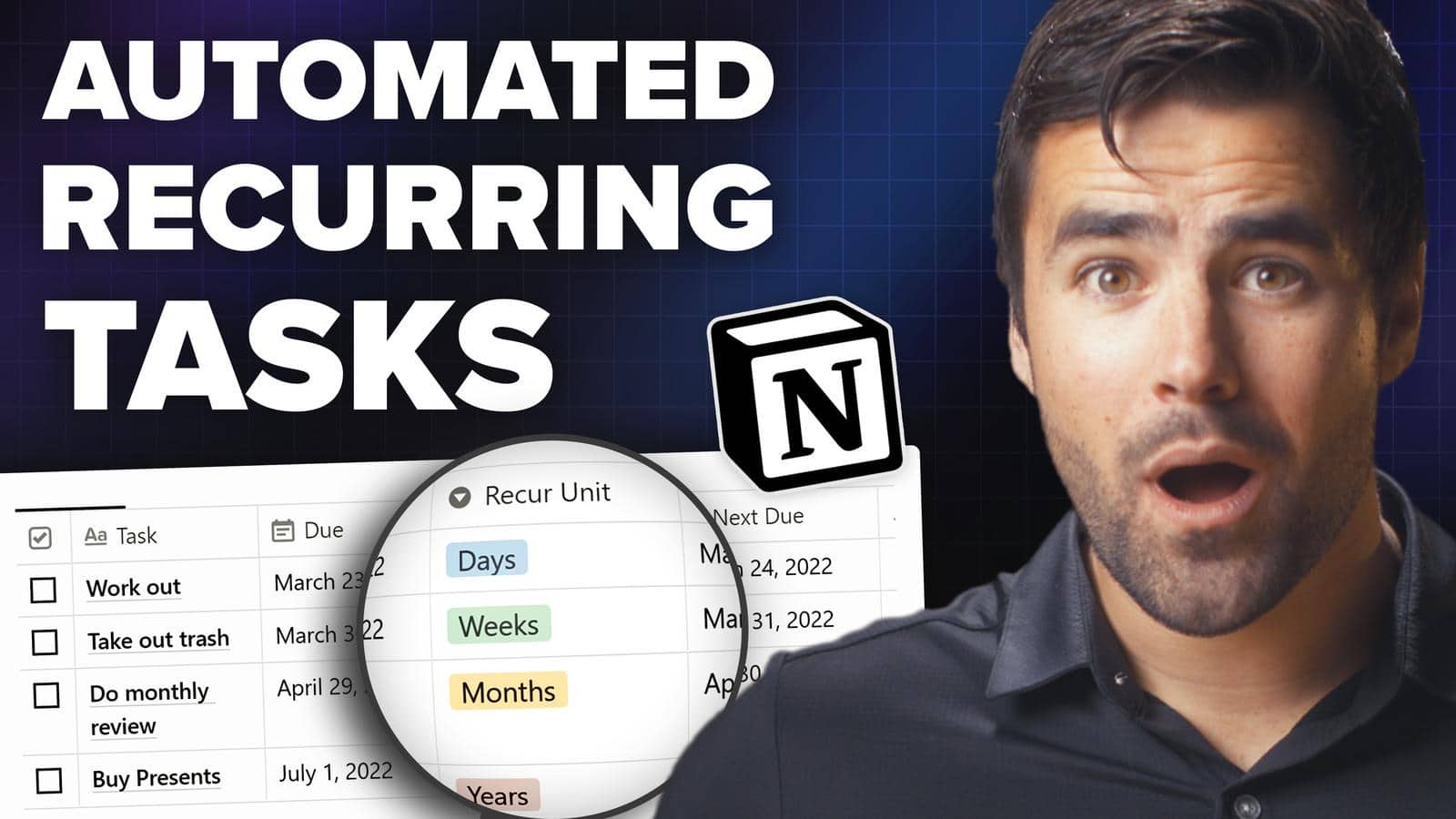
Example Database
This example database shows the numbered week of year that matches the date in the Date property.
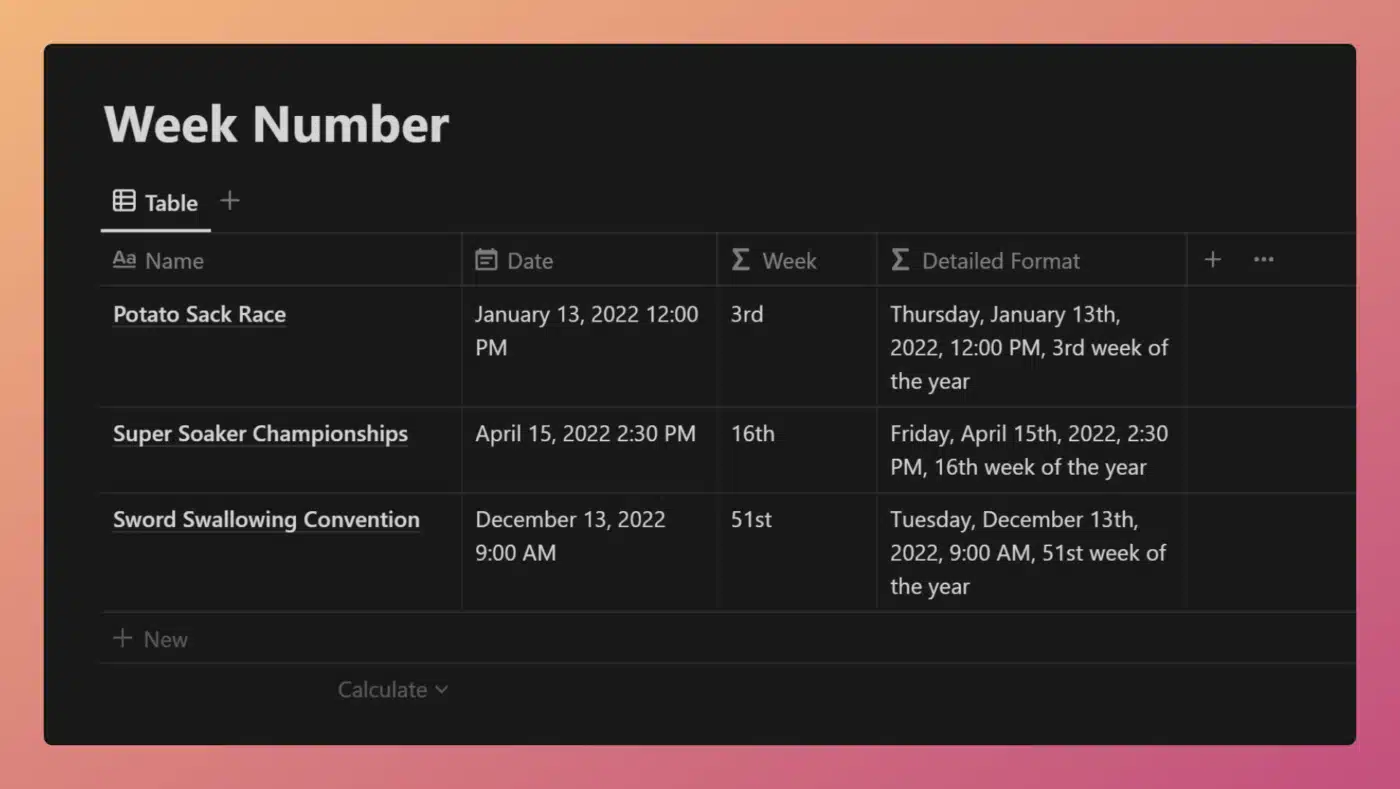
View and Duplicate Database
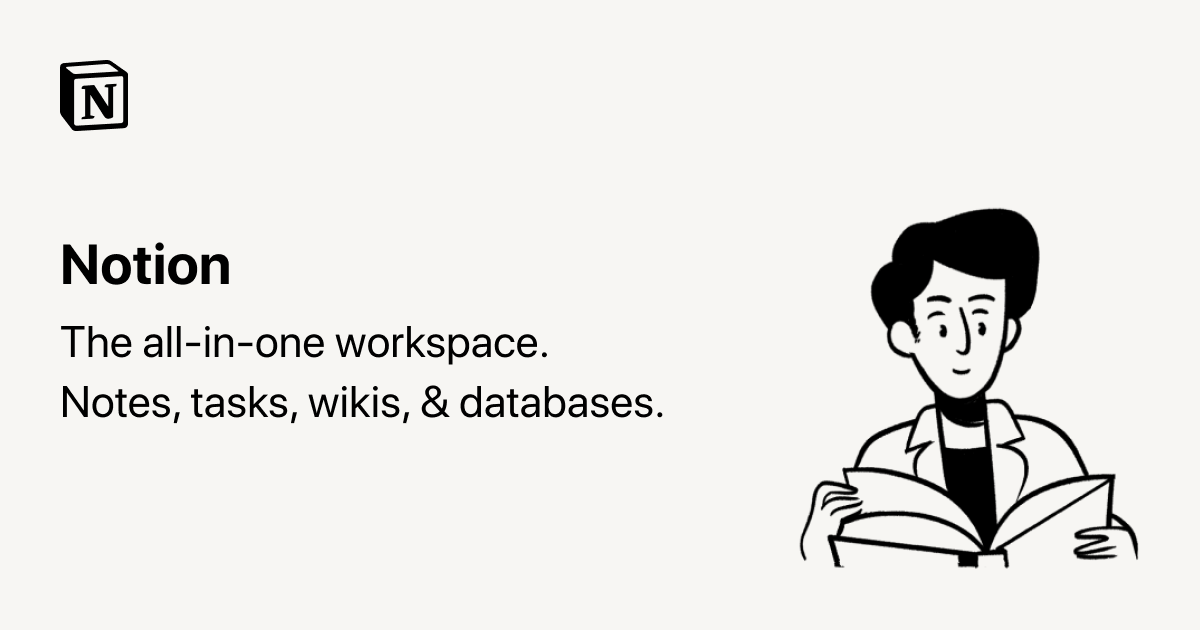
“Week” Property Formula
formatDate(prop("Date"), "wo")
Code language: JavaScript (javascript)
“Detailed Format” Property Formula
formatDate(prop("Date"), "dddd, MMMM Do, YYYY, HH:mm A, wo [week of the year]")
Code language: JavaScript (javascript)
Formatting Tokens
Time
Token | Description | Example |
---|---|---|
A | Uppercase meridiem | PM |
a | Meridiem | pm |
H | Hour in 24-hour time, no padding | 4 |
HH | Hour in 24-hour time, padded to 2 | 04 |
h | Hour in 12-hour time, no padding | 4 |
hh | Hour in 12-hour time, padded to 2 | 04 |
k | 1-based hour in 24-hour time, no padding | 4 |
kk | 1-based hour in 24-hour time, padded to 2 | 04 |
m | Minute, no padding | 8 |
mm | Minute, padded to 2 | 08 |
s | Second, no padding * | 0 |
ss | Second, padded to 2 * | 00 |
S | Fractional seconds, between 0 and 9 * | 0 |
SS | Fractional seconds, between 0 and 99, padded to 2 * | 00 |
SSS | Fractional seconds, between 0 and 999, padded to 3 * | 000 |
LT | Localized 12-hour time (no padding), uppercase meridiem | 4:08 PM |
LTS | Localized 12-hour time with seconds (no padding), uppercase meridiem * | 4:08:00 PM |
0
.Days
Token | Description | Example |
---|---|---|
D | Day of month, no padding | 21 |
Do | Day of month, with ordinal suffix | 21st |
DD | Day of month, padded to 2 | 21 |
DDD | Day of year, no padding | 294 |
DDDo | Day of year, with ordinal suffix | 294th |
DDDD | Day of year, padded to 3 | 294 |
d | Zero-based day of week, no padding | 3 |
do | Zero-based day of week, with ordinal suffix | 3rd |
dd | Localized day of week name, 2-letter abbreviation | We |
ddd | Localized day of week name, 3-letter abbreviation | Wed |
dddd | Localized day of week name, unabbreviated | Wednesday |
E | ISO day of week, no padding | 3 |
e | Localized day of week, no padding | 3 |
Weeks
Token | Description | Example |
---|---|---|
w | Week of year, no padding | 4 |
wo | Week of year, with ordinal suffix | 4th |
ww | Week of year, padded to 2 | 04 |
W | ISO week of year, no padding | 4 |
Wo | ISO week of year, with ordinal suffix | 4th |
WW | ISO week of year, padded to 2 | 04 |
Months
Token | Description | Example |
---|---|---|
M | Month, no padding | 4 |
Mo | Month, with ordinal suffix | 4th |
MM | Month, padded to 2 | 04 |
MMM | Localized month name, 3-letter abbreviation | Oct |
MMMM | Localized month name, unabbreviated | October |
Quarters
Token | Description | Example |
---|---|---|
Q | Quarter, no padding | 4 |
Qo | Quarter, with ordinal suffix | 4th |
Quarter, padded to 2 | 04 |
Years
Token | Description | Example |
---|---|---|
Y | ISO year | 2015 |
YY | Year, 2 digits | 15 |
YYYY | Year, 4 digits | 2015 |
YYYYYY | Expanded year | +002015 |
gg | Week year, 2 digits | 15 |
gggg | Week year, 4 digits | 2015 |
GG | ISO week year, 2 digits | 15 |
GGGG | ISO week year, 4 digits | 2015 |
Timestamp
Token | Description | Example |
---|---|---|
X | Unix timestamp | 1445468880 |
x | Unix millisecond timestamp | 1445468880000 |
Time Zone
Token | Description | Example |
---|---|---|
Z | Time zone, short offset | -07:00 |
ZZ | Time zone, techie offset | -0700 |
ZZZ | Time zone, unabbreviated named offset | Pacific Daylight Time |
Full Date & Time
Token | Description | Example |
---|---|---|
L | Localized month (padded to 2), day of month (padded to 2), year (4 digits) | 10/21/2015 |
l | Localized month (no padding), day of month (no padding), year (4-digits) | 10/21/2015 |
LL | Localized month name (unabbreviated), day of month (no padding), year (4-digits) | October 21, 2015 |
ll | Localized month name (3-letter abbreviation), day of month (no padding), year (4-digits) | Oct 21, 2015 |
LLL | Localized month name (unabbreviated), day of month (no padding), year (4-digits), 12-hour time (no padding), uppercase meridiem | October 21, 2015 4:08 PM |
lll | Localized month name (3-letter abbreviation), day of month (no padding), year (4-digits), 12-hour time (no padding), uppercase meridiem | Oct 21, 2015 4:08 PM |
LLLL | Localized day of week name (unabbreviated), month name (unabbreviated), day of month (no padding), year (4-digits), time (no padding) | Wednesday, October 21, 2015 4:08 PM |
llll | Localized day of week name (3-letter abbreviation), month name (3-letter abbreviation), day of month (no padding), year (4-digits), time (no padding) | Wed, Oct 21, 2015 4:08 PM |
Other
Token | Description | Example |
---|---|---|
[Great Scott!] | Escaped characters (between square brackets) | Great Scott! |