The empty()
function returns true if its argument is empty, or has a value that equates to empty – including 0
, false
, ""
, and []
.
In truth, empty()
is a function that checks for falsiness, not true emptiness.
empty(string)
empty(number)
empty(boolean)
empty(date)
empty(list)
string.empty()
number.empty()
boolean.empty()
date.empty()
list.empty()
Code language: JavaScript (javascript)
empty()
accepts all data types, including strings, numbers, Booleans, and dates.
Example Formulas
empty("") /* Output: true */
0.empty() /* Output: true */
empty(false) /* Output: true */
[].empty() /* Output: true */
empty([""]) /* Output: false */
/* Assume a row where the Name property is currently blank */
prop("Name").empty() /* Output: true */
Code language: JavaScript (javascript)
empty()
can be preceded by the not operator to ensure that a property or value is not empty:
/* Assume a row where the Name property contains text */
not empty(prop("Name")) /* Output: true */
!prop("Title").empty() /* Output: true */
/* The same result can be accomplished with conditional operators (Assume the Name property contains text in this row) */
empty(prop("Name")) ? false : true /* Output: true */
Code language: JavaScript (javascript)
Checking for True “Emptiness”
By default, empty()
checks for falsiness, and there are four possible falsy values in a Notion database property:
To check for true emptiness, you can use the And operator to check that a property’s value isn’t one of the three falsy, but not empty, values:
/* Outputs true only if the Number property's value is truly empty */
empty(prop("Number")) and prop("Number") != 0
/* Outputs true only if the String property's value is truly empty */
empty(prop("String")) and prop("String") != ""
/* Outputs true only if the Checkbox property's value is truly empty */
empty(prop("Checkbox")) and prop("Checkbox") != false
Code language: JavaScript (javascript)
For numbers, you can also use:
prop("Number") == toNumber("")
Code language: JavaScript (javascript)
Learn more about why this works: Return Null/Empty Values in Formulas
Example Database
The example database below shows how you can sort sub-tasks by their parent task’s due date. This pictured view is simplified for ease-of-use; view the database’s All Properties tab to see every property at work.
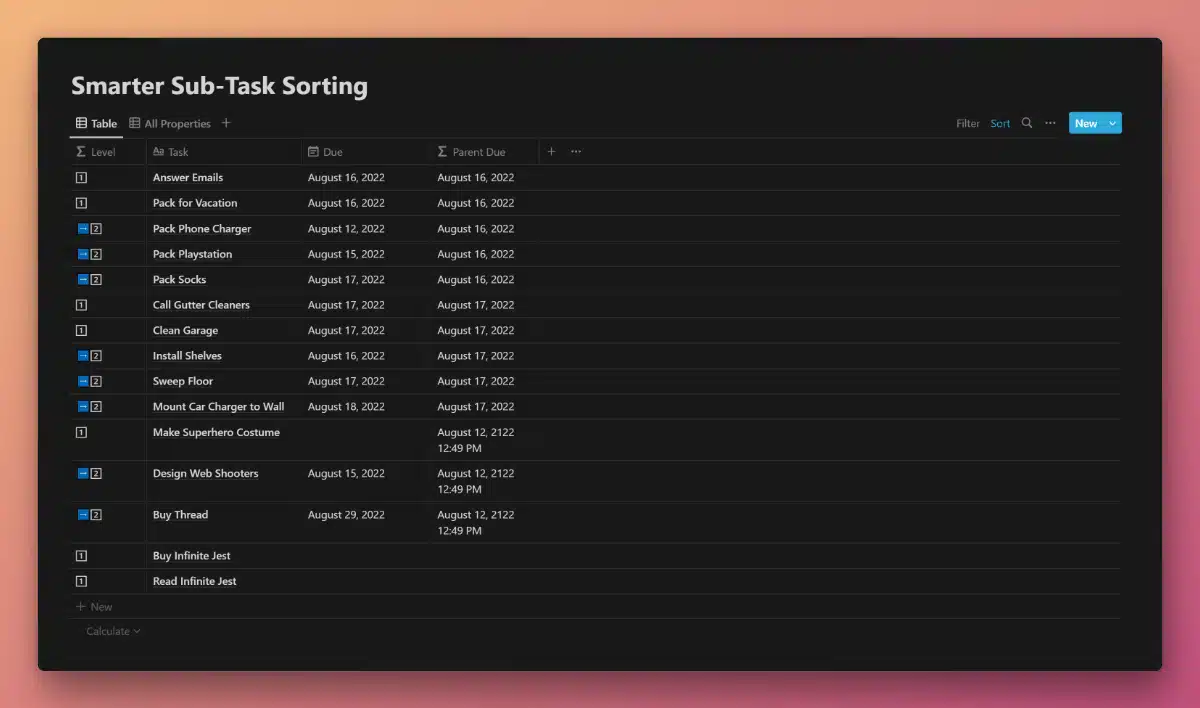
View and Duplicate Database
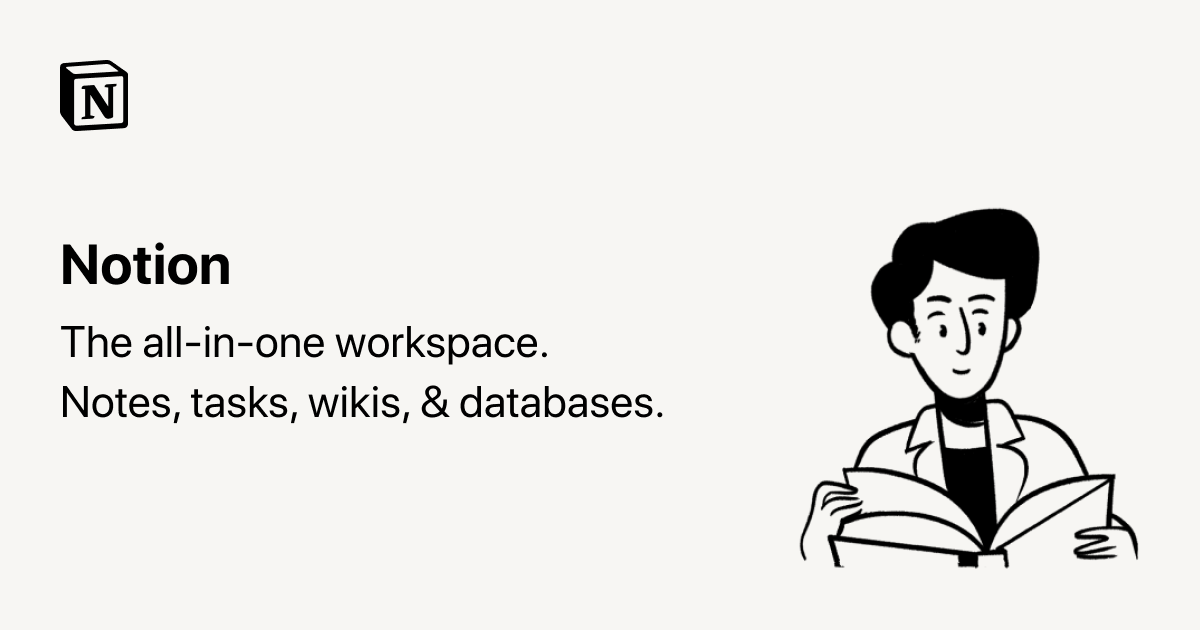
“Parent Due” Property Formula
if(
not empty(
prop("Parent Task")
),
prop("Parent Due Rollup"),
if(
empty(
prop("Sub-Tasks")
),
prop("Due"),
if(
not empty(
prop("Due")
),
prop("Due"),
dateAdd(
now(),
100,
"years"
)
)
)
)
Code language: JavaScript (javascript)
This formula uses both empty()
and not empty()
in conjunction with multiple nested if statements in order to determine a Parent Due Date.
The entire database view is then sorted by:
- Parent Due Date
- Parent Task Name
- Due Date
Notice that the formula also calls in a Parent Due Rollup property, which is actually configured to pull in the Parent Due Date content of the Parent Task:
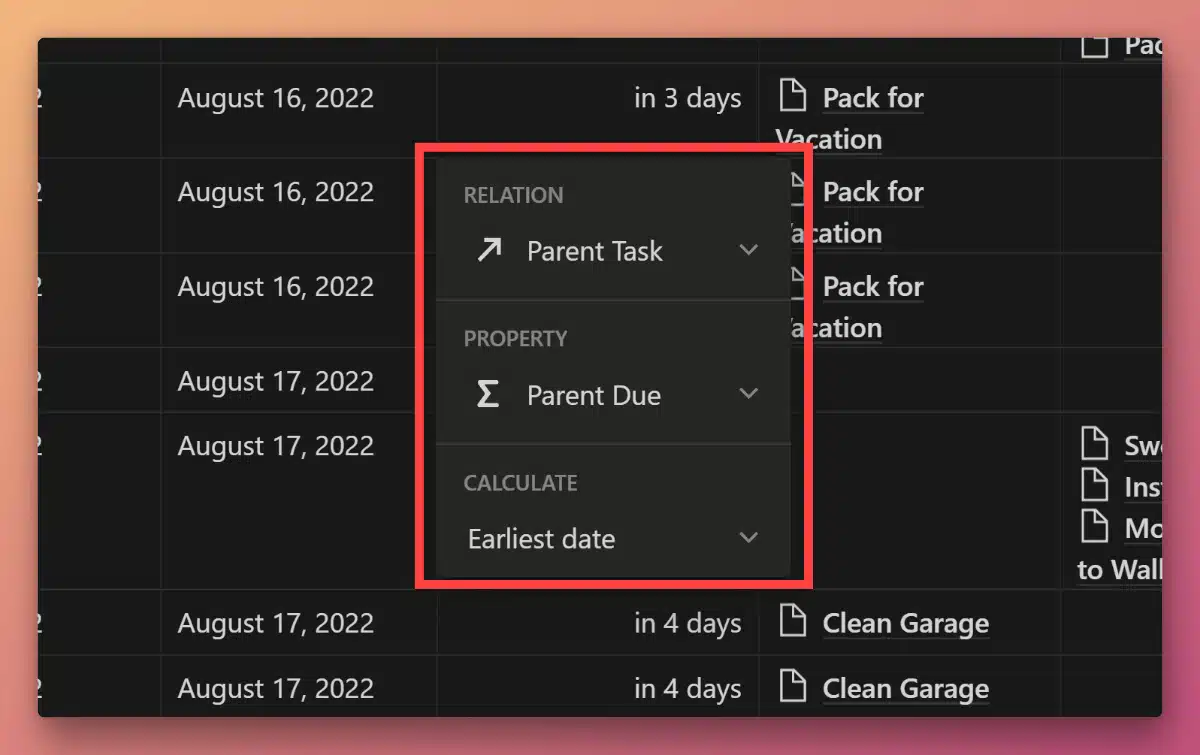
That’s right – this is a formula, which pulls in its own output (from other database rows)!
Let’s work through what this formula is doing:
- First, it checks if Parent Task is not empty. If so, we know we’re dealing with a sub-task, so we output the value of Parent Due Rollup. Note that Parent Due Rollup’s content is determined by the rest of the logic in this formula.
- If Parent Task is empty, then we check if Sub-Tasks is empty as well. If so, we’re dealing with a “lone” task – it’s not a parent task nor a sub-task. In this case, we output its Due date value.
- If Sub-Tasks is not empty, then we know we’re dealing with a parent task. Next, we check if its Due property is not empty.
- If it does have a date, we output that. If not, we output a standard date of now plus 100 years (using dateAdd). This ensures that parent tasks with no actual due date have a hidden “default” date, enabling sub-tasks to still be sorted underneath them as intended.
Other formula components used in this example:
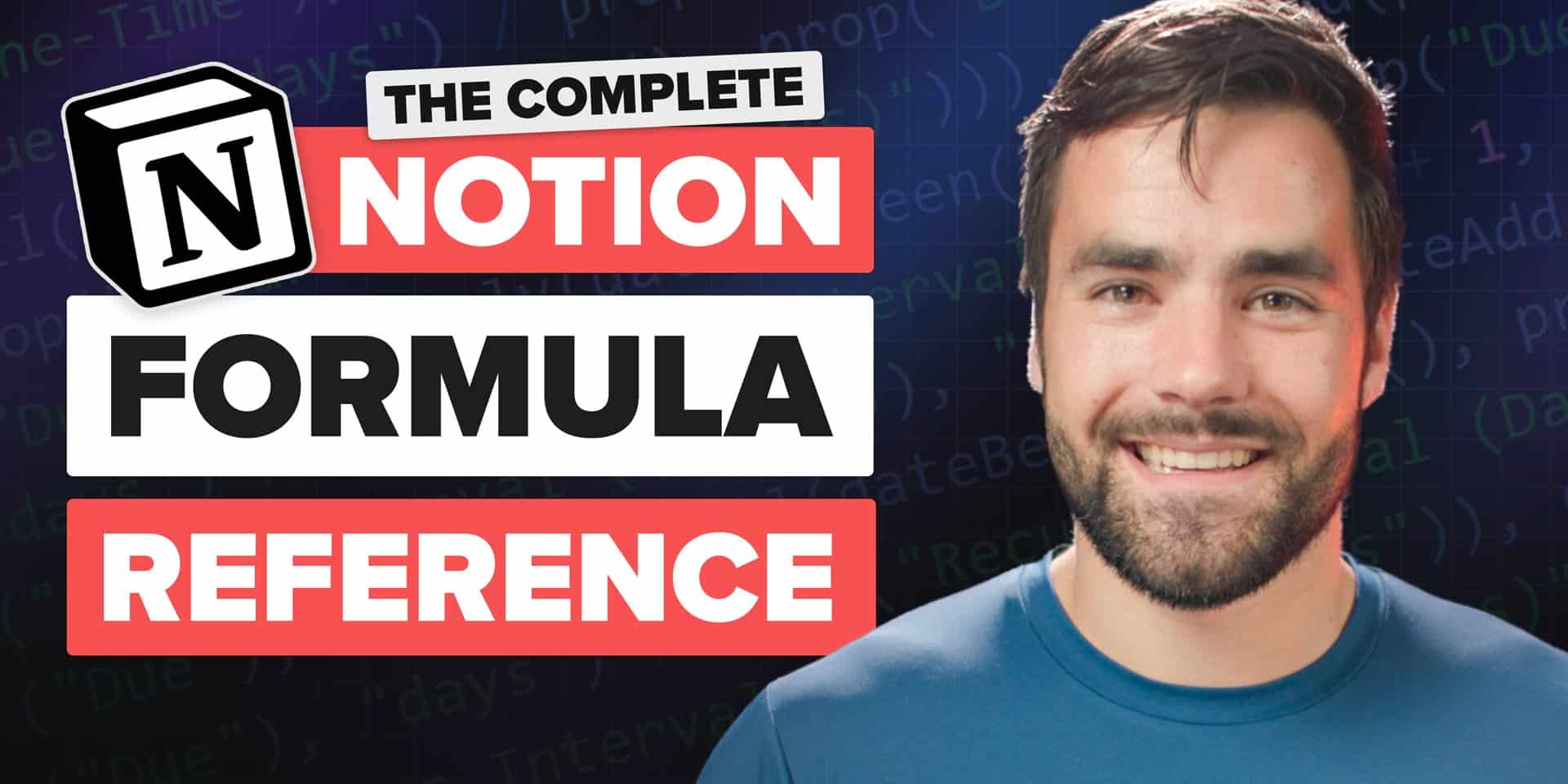
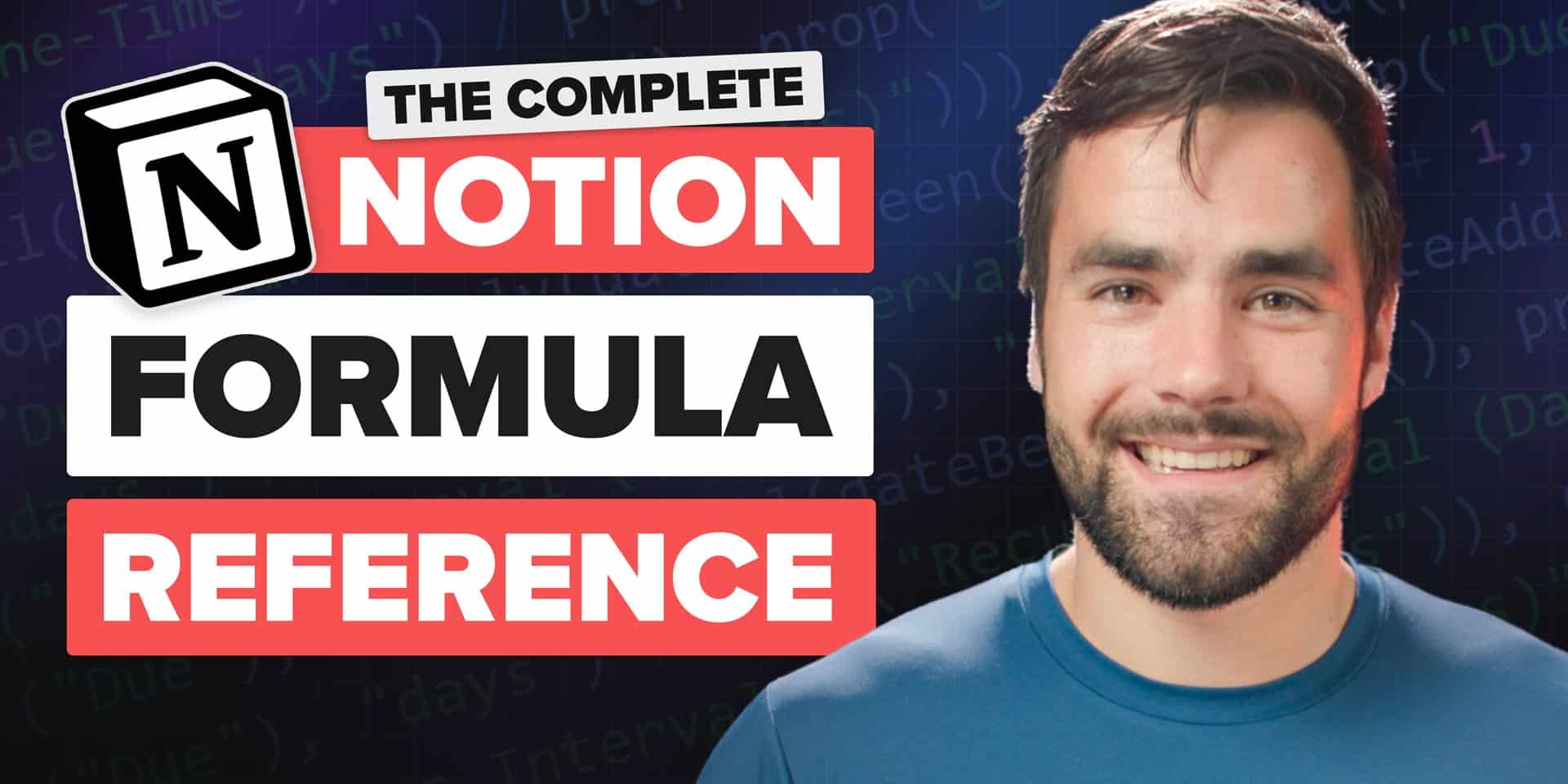
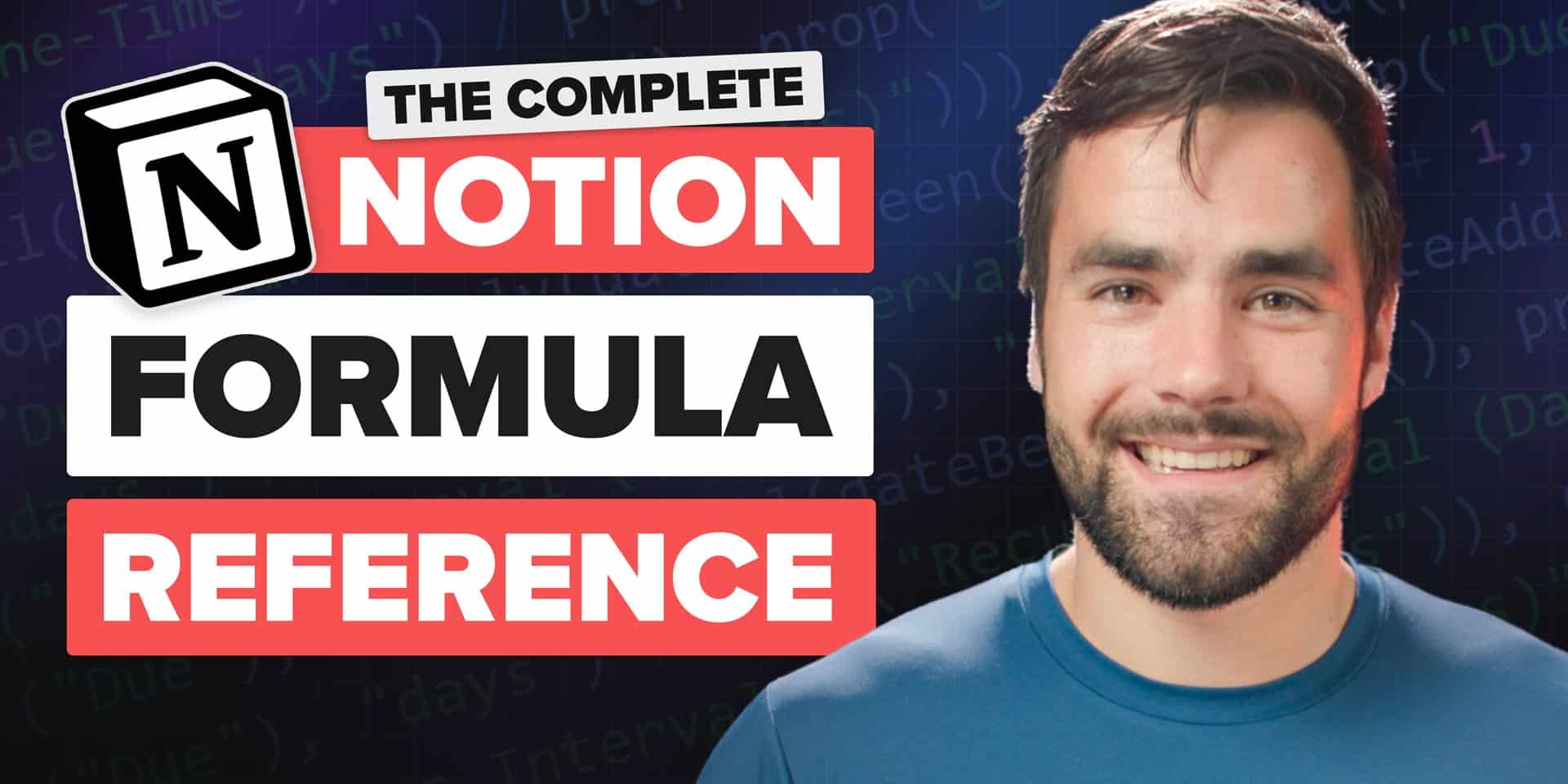