Unlike Javascript, Notion formulas do not have a null data type. However, it is possible to make a formula return a null/empty value using some easy workarounds.
To return a null/empty string, use a pair of double quotes ""
:
""
/* Testing emptiness */
empty("") /* Output: true */
Code language: JavaScript (javascript)
To return a null/empty number, use the following formula (credit to aNotioneer on Twitter for this number workaround):
toNumber("")
/* Testing emptiness */
empty(toNumber("")) /* Output: true */
Code language: JavaScript (javascript)
Likewise, you can return a null/empty date object using this formula:
parseDate("")
/* Testing emptiness */
empty(parseDate("")) /* Output: true */
Code language: JavaScript (javascript)
There is no possible null/empty state for Booleans/Checkboxes. However, you can convert Booleans to strings with format in order to create a setup where true/false/empty is possible:
/* Assume "Checkbox" is a Boolean/Checkbox property.
/* Invalid; will throw an error: */
if( 1 > 2, prop("Checkbox"), "")
/* Valid. Will output "true", "false", or an empty value. */
if( 1 > 2, format(prop("Checkbox")), "")
Code language: JavaScript (javascript)
You can return an empty list by using the following formula:
[]
/* Testing emptiness */
empty([]) /* Output: true */
/* Adding an empty string, number, boolean, or list inside the
square brackets will mean the list is no longer considered empty */
[""]
[0]
[false]
[[]]
/* Testing emptiness */
empty([""]) /* Output: false */
empty([0]) /* Output: false */
empty([false]) /* Output: false */
empty([[]]) /* Output: false */
Code language: JavaScript (javascript)
Example Database
This example database demonstrates how outputting null/empty values from several formulas allows us to calculate a per-genre average rating inside a Movies database.
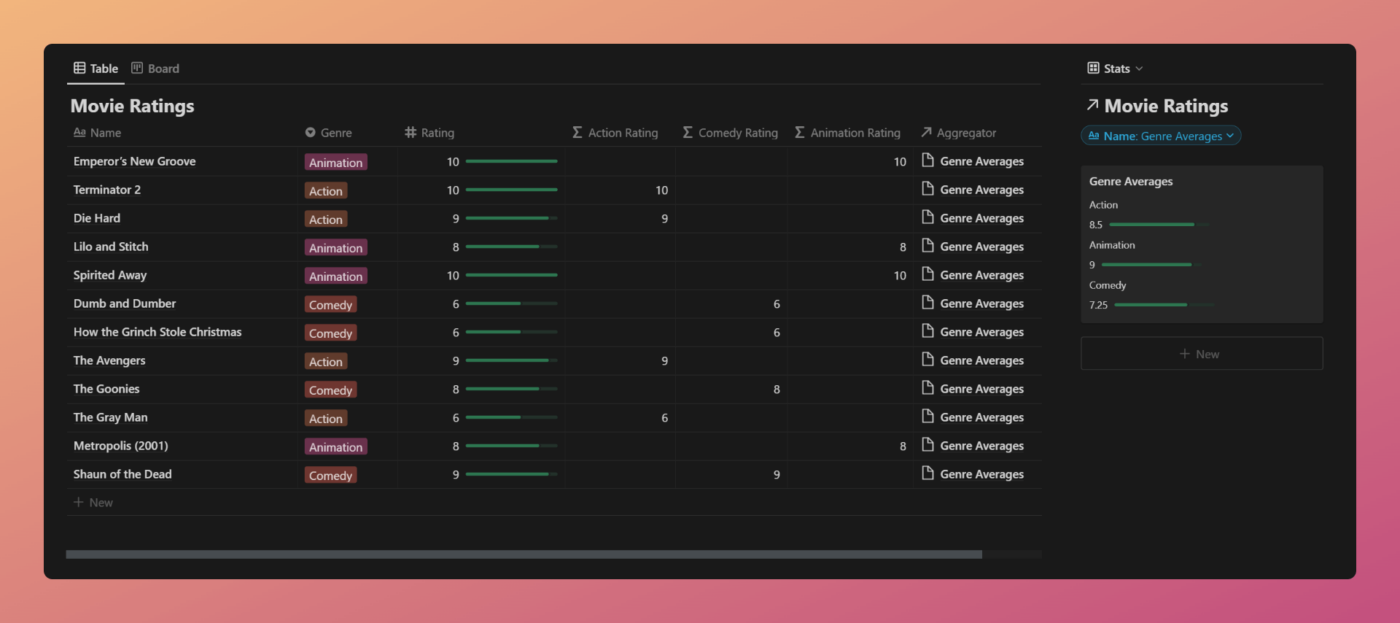