The sort()
function accepts a list and returns the same list in a sorted order.
sort(list)
list.sort()
sort(list, expression)
list.sort(expression)
Code language: JavaScript (javascript)
By default, sort()
will sort the input list based on data type of each list item:
- String: Alphabetical (A-Z)
- Number: Ascending (
0
,1
) - Boolean:
false
,true
(i.e.0
,1
) - Date: Earlier, Later
- List: List is treated as a string of comma-separated values
- Pages: Treated as strings
- People: Treated as strings
When multiple data types are present in the input list, all data types are treated as strings for the purposes of determining the sort order. The items in the returned list will retain their original data types.
You can also pass an expression that defines a custom comparator. Just like filter, map, and other list functions, you can use the current
keyword to represent each list item, then chain methods onto it in order to define your comparator.
[1, 2, 3, 4, 5, 6, 7, 8, 9].sort(
current % 2
)
/* Output: [2, 4, 6, 8, 1, 3, 5, 7, 9]
Code language: JavaScript (javascript)
Example Formulas
["Monkey D. Luffy", "Zoro", "Nami", "Chopper", "Usopp"].sort()
/* Output: ["Chopper", "Monkey D. Luffy", "Nami", "Usopp", "Zoro"] */
[5, 2, 8, 3, 1].sort().reverse()
/* Output: [8, 5, 3, 2, 1] */
["Monkey D. Luffy", "Zoro", "Nami", "Chopper", "Usopp"].sort(
current.length()
)
/* Output: ["Nami", "Zoro", "Usopp", "Chopper", "Monkey D. Luffy"] */
[now(), today()].sort()
/* Output: [December 26, 2024, December 26, 2024 5:55PM] */
/* In a Projects database with a Tasks relation */
prop("Task").sort(
current.prop("Due")
)
/* Output: Returns a list of Task pages, sorted by Due date in ascending order */
/* Sorting by a specific element in each internal list */
[
[ "Luffy", 582 ],
[ "Goku", 9001 ],
[ "Captain America", 65]
].sort(
current.at(1)
)
/* Output: List is sorted by power level in ascending order */
Code language: JavaScript (javascript)
Example Database
This example database contains many examples of the sort()
function in action.
View and Duplicate Database
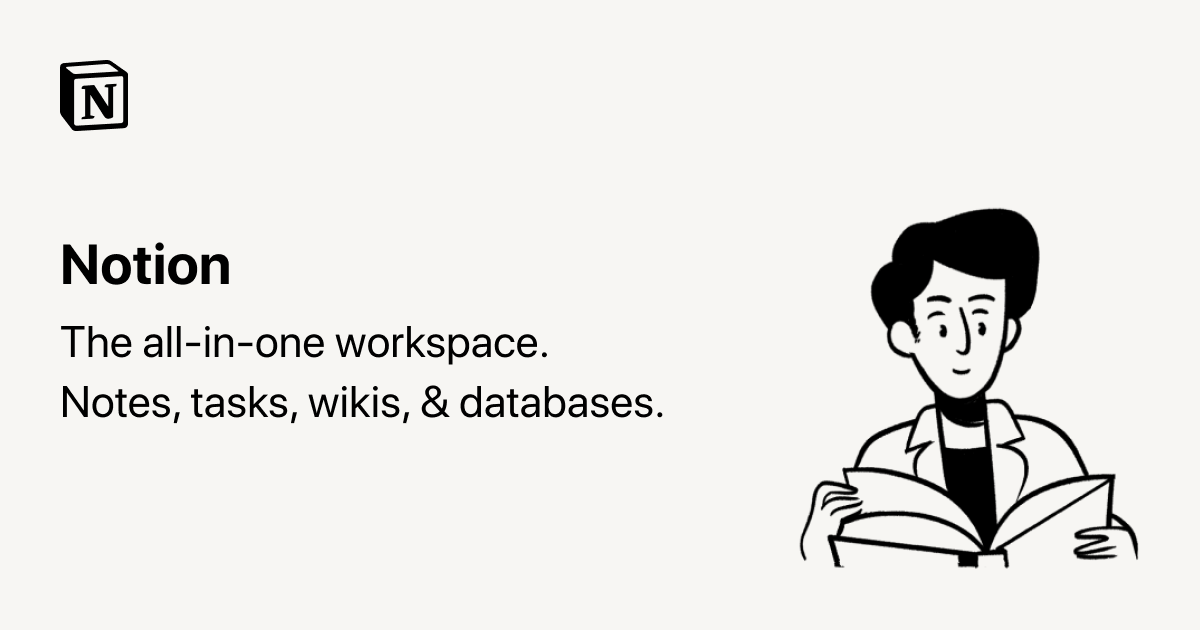
“Last Completed” Property Formula
prop("Task").filter(
current.prop("Completed")
).sort(
current.prop("Completed")
).last()
Code language: JavaScript (javascript)
The Last Completed property in the example database is a good example of a formula that combines the sort()
function with the filter function.
First, the list of Pages in the Task relation property is passed through filter()
, returning a new list that only contains tasks that have a Completed property value. Simply using current.prop("Complete")
constitutes an empty/not empty comparison.
Next, the list of completed tasks is passed into sort()
, where the Completed property value of each task is used as the comparator for sorting.
Finally, we use the last function to access the most recently completed task (e.g., the one with the latest Completion date value).
Note that we could have also passed the result of sort() into reverse, and then into first, to get the same result.