The round()
function rounds its argument to the nearest integer (whole number).
round(number)
number.(round)
Code language: JavaScript (javascript)
The exact midpoint between two whole numbers will round up towards positive infinity, not “away from zero”.
For example: round(-4.5)
will round up to 4
.
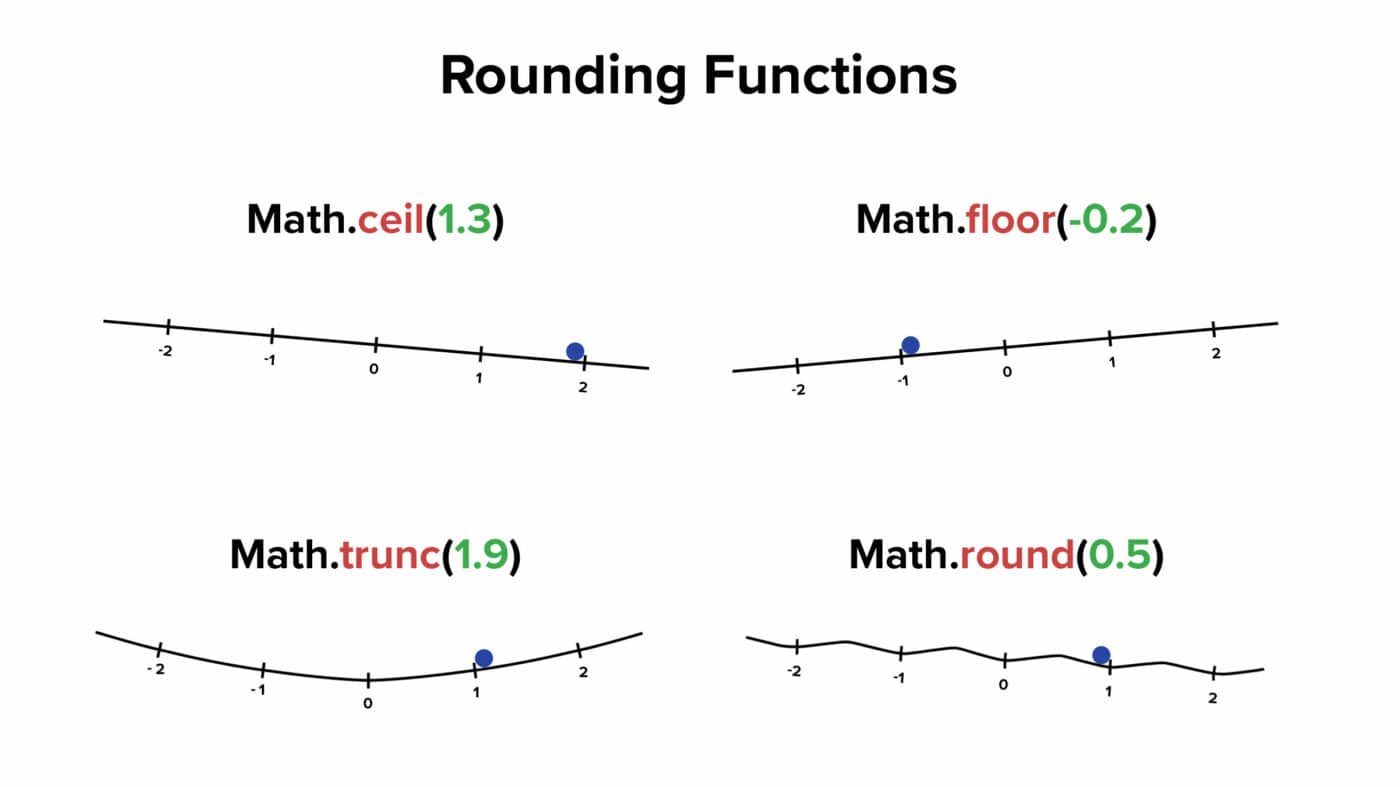
Example Formulas
round(4.5) /* Output: 5 */
4.49.round() /* Output: 4 */
round(-4.49) /* Output: -4 */
-4.5.round() /* Output: -4 */
round(-4.51) /* Output: -5 */
Code language: JavaScript (javascript)
Rounding to Specific Decimal Places
The round()
function doesn’t accept additional arguments, so it can’t natively round a number to a specific decimal place.
However, you can accomplish this using the following formula:
/* Round to two decimal places */
round([number] * 100) / 100
/* Round to three decimal places */
round([number] * 1000) / 1000
Code language: JavaScript (javascript)
The number of zeroes corresponds to the number of decimal places to which your number will be rounded.
round(9.24551 * 10) / 10 /* Output: 9.2 */
(4.158015 * 100).round() / 100 /* Output: 4.16 */
round(5145.018394 * 10000) / 10000 /* Output: 5145.0184 */
Code language: JavaScript (javascript)
Example Database
The example database below contains a formula that rounds the input number to several different decimal places.
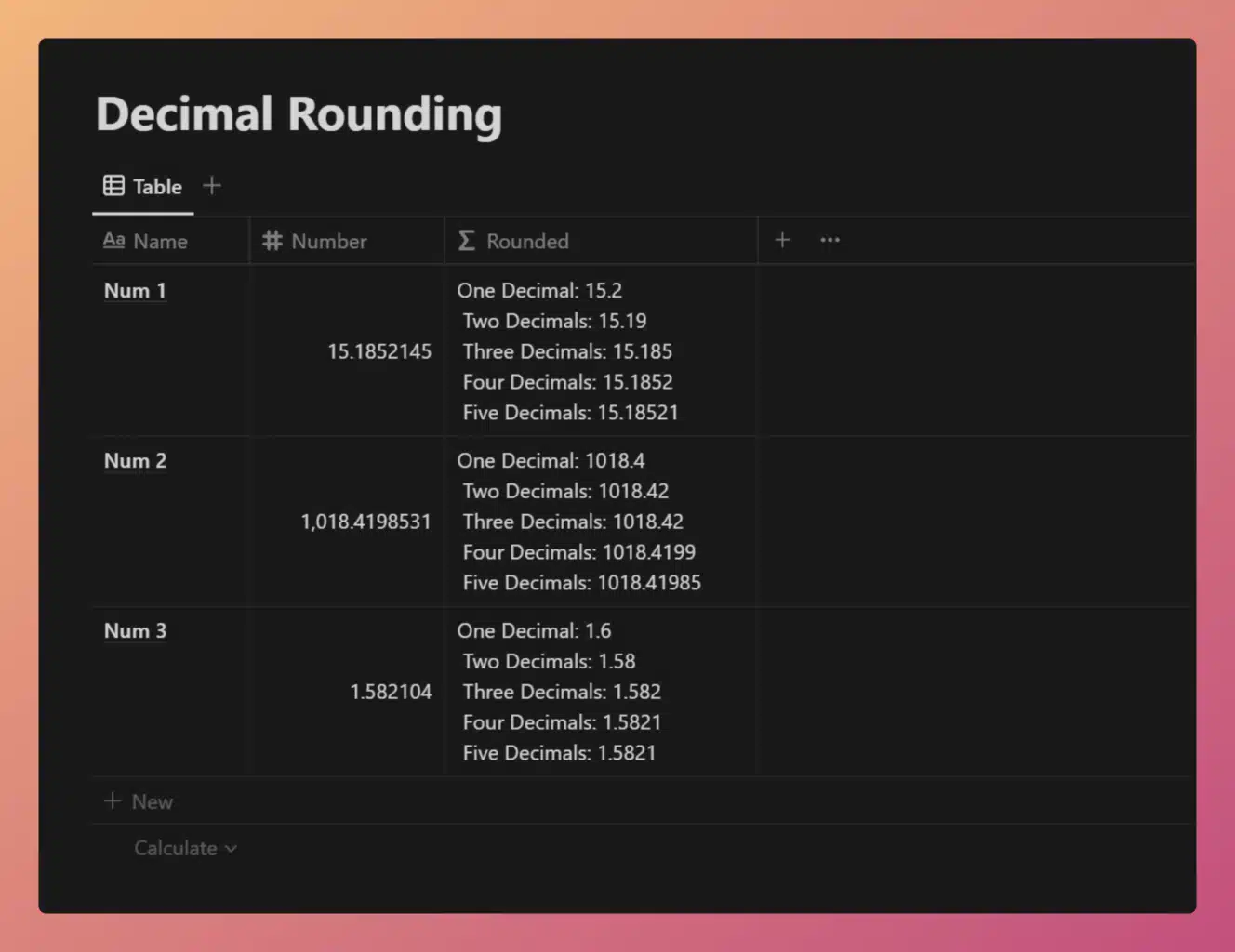
View and Duplicate Database
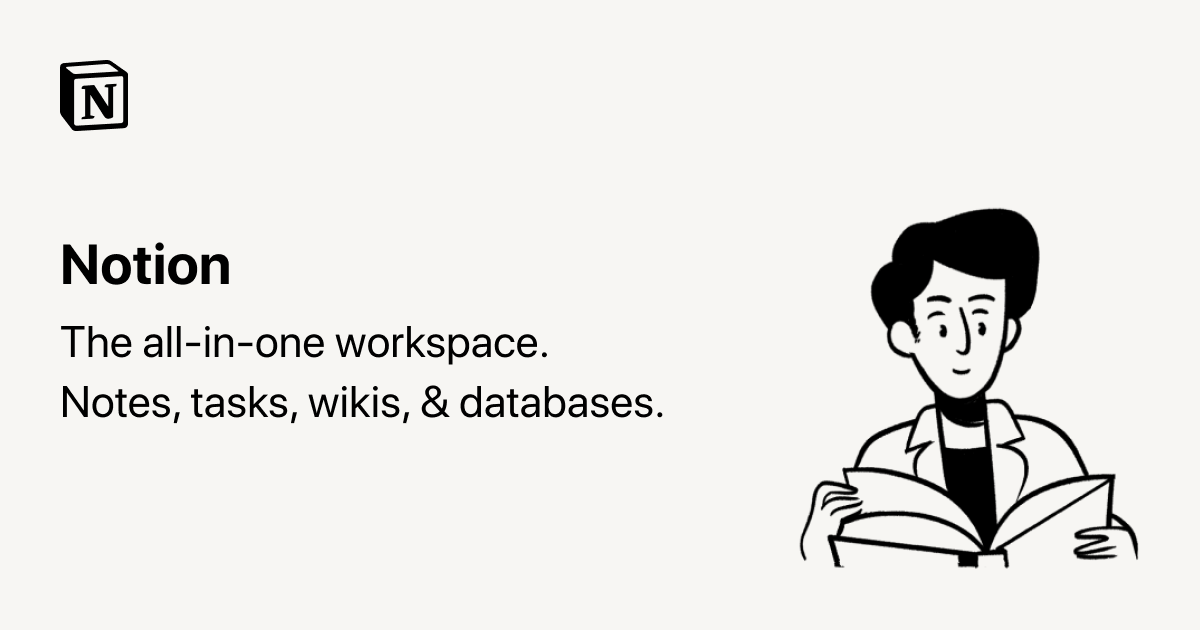
“Rounded” Property Formula
"One Decimal: " + (round(prop("Number") * 10) / 10) +
"\nTwo Decimals: " + (round(prop("Number") * 100) / 100) +
"\nThree Decimals: " + (round(prop("Number") * 1000) / 1000) +
"\nFour Decimals: " + (round(prop("Number") * 10000) / 10000) +
"\nFive Decimals: " + (round(prop("Number") * 100000) / 100000)
Code language: JavaScript (javascript)
This formula demonstrates decimal-rounding to five different places.
Each rounded number is then converted to a string using the format function, allowing us to craft a five-line block of text.
Note how \n
allows us to create a new line in the formula output.
Other formula components used in this example:
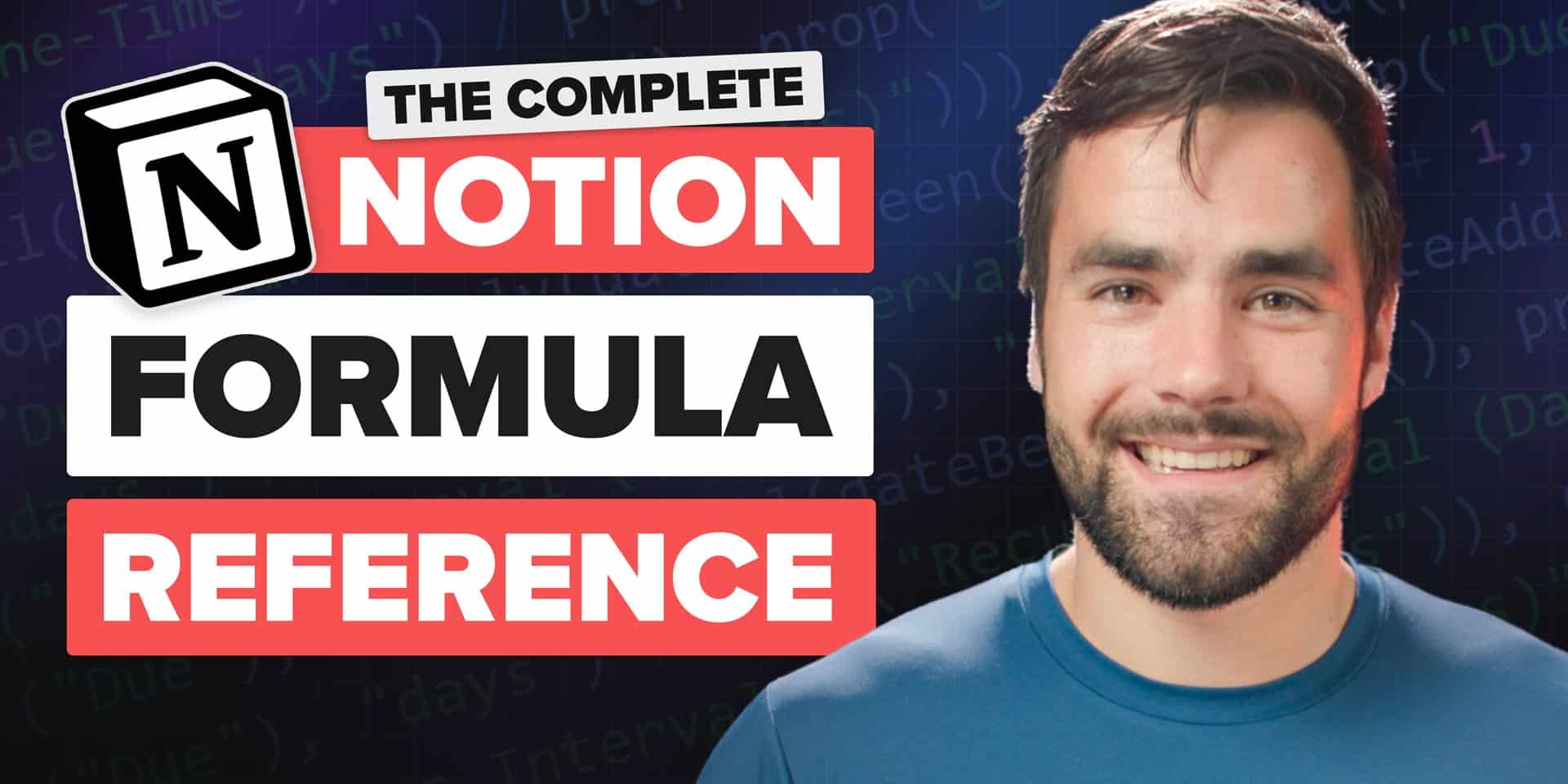
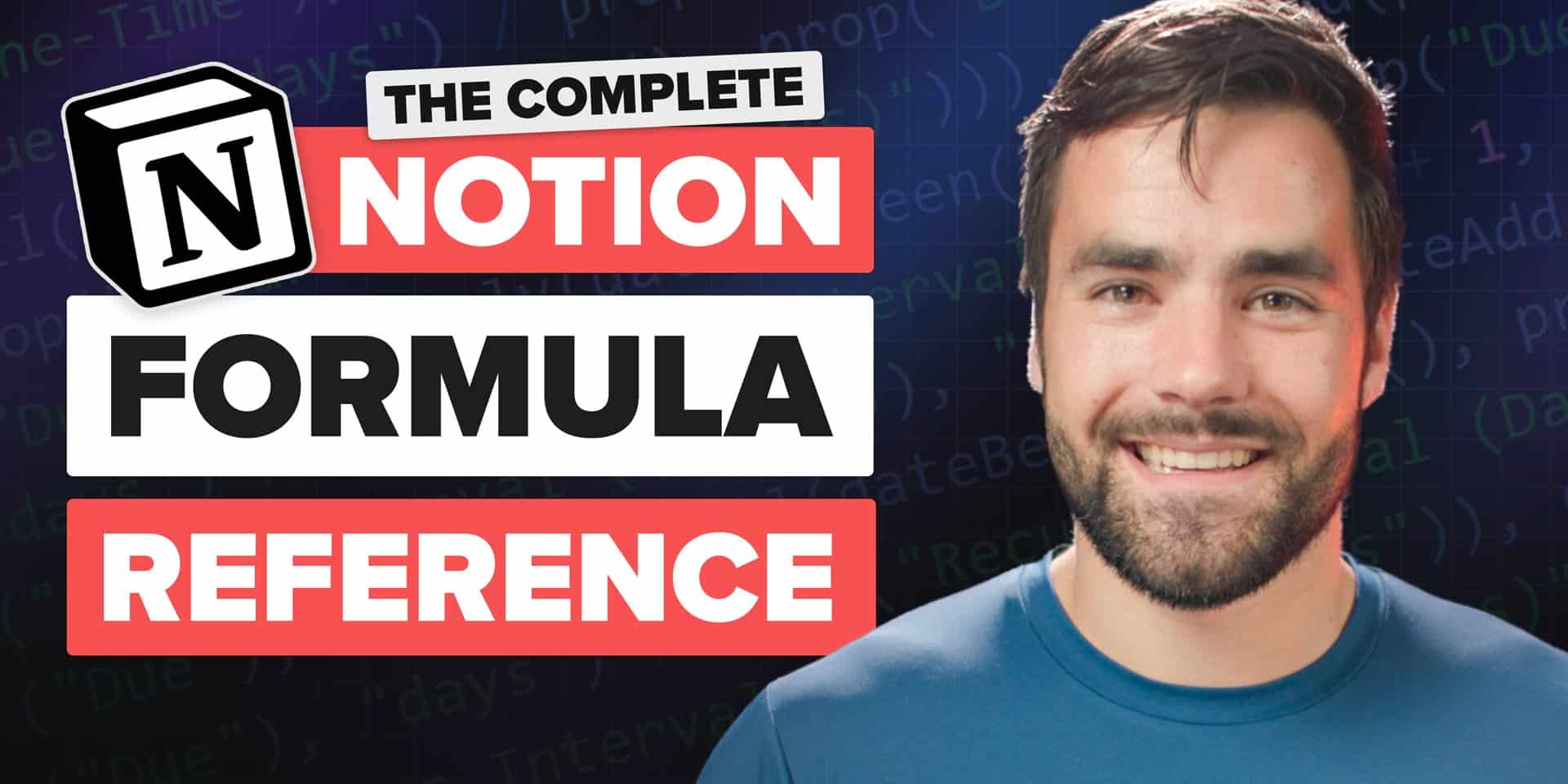
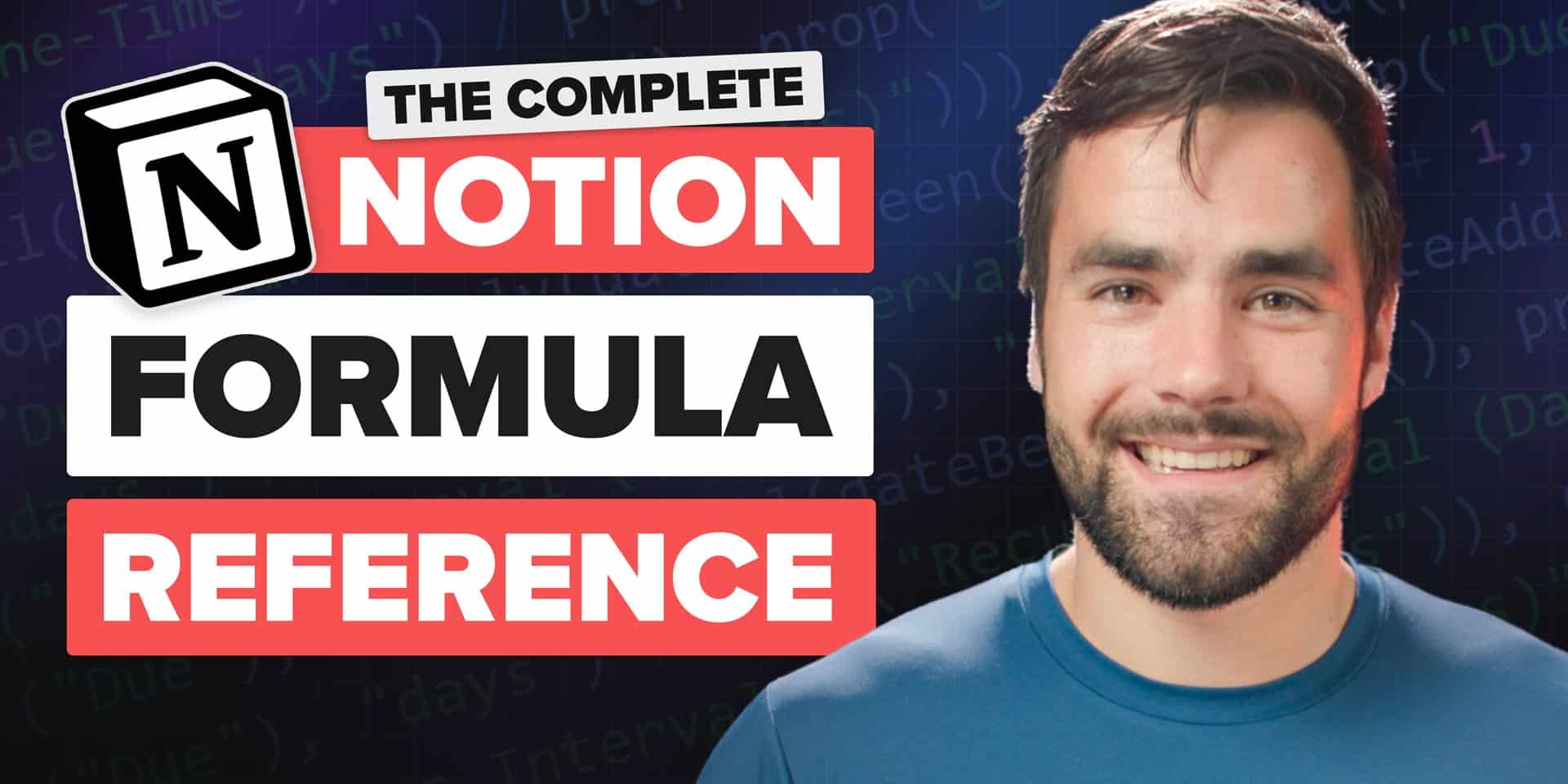
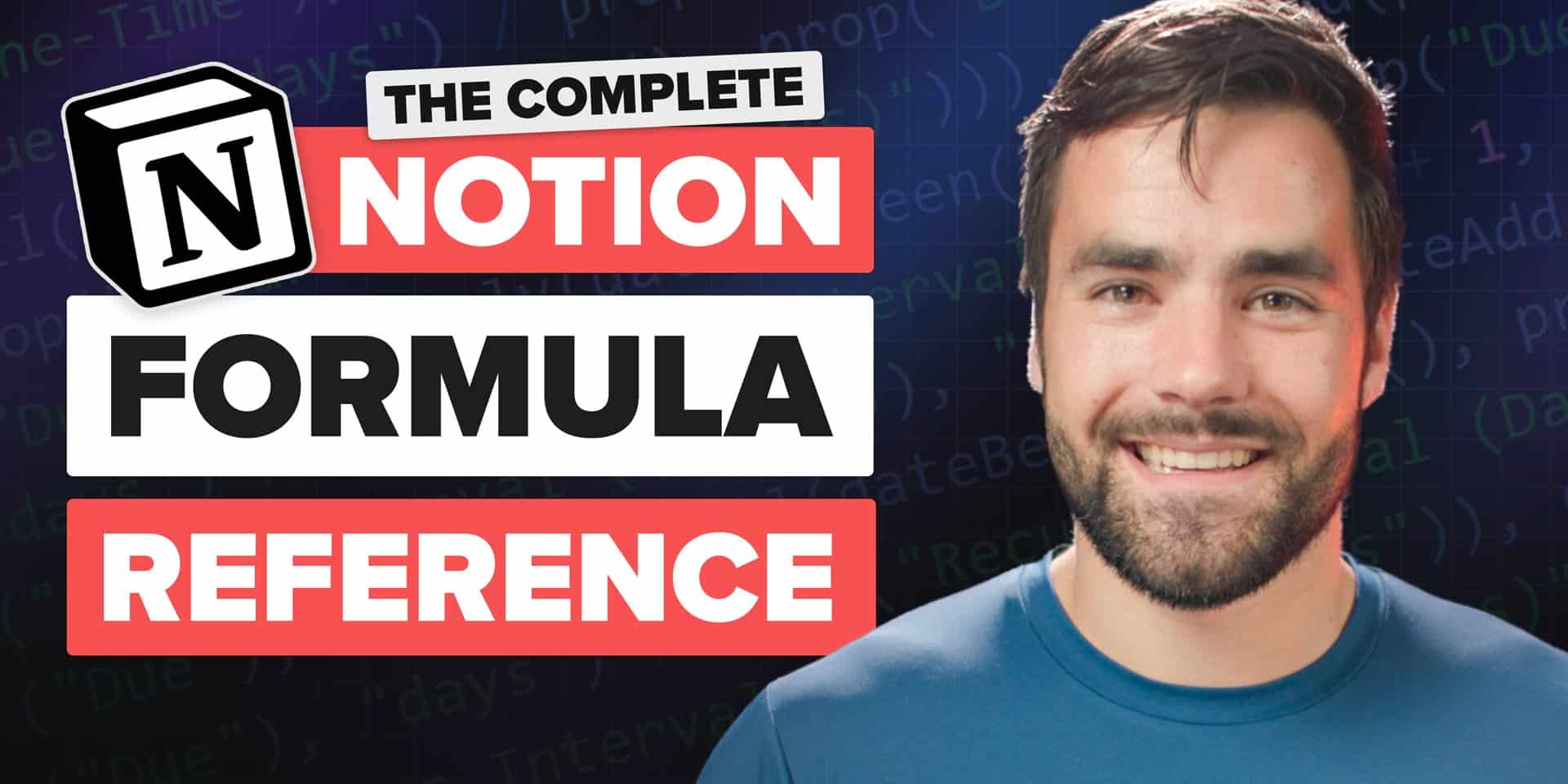