The less than (<
) operator returns true if its left operand is less than its right operand. It accepts numeric, string, date, and Boolean operands.
number < number
string < string
Boolean < Boolean
date < date
Code language: JavaScript (javascript)
Example Formulas
2 < 1 /* Output: false */
42 < 50 /* Output: true */
"a" < "b" /* Output: true */
/* Boolean values equate to 1 (true) and 0 (false). */
false < true /* Output: true */
true < true /* Output: false */
/* For dates, "less than" equates to "before". */
now() < dateAdd(now(), 1, "months") /* Output: true */
Code language: JavaScript (javascript)
Good to know: When comparing dates, “greater” = “later”.
Good to know: The less than (<
) operator cannot be chained in a Notion formula. A formula like 1 < 2 < 3
won’t work. Use the and operator to get around this – e.g. 1 < 2 and 2 < 3
.
Example Database
The example database below tracks votes amongst a pirate crew. For each issue, a quorum must be reached; at least 3 members must vote. Once a quorum is reached, only proposals that receive more Yays than Nays will be passed and enacted.
The Result formula displays the status of each proposal.
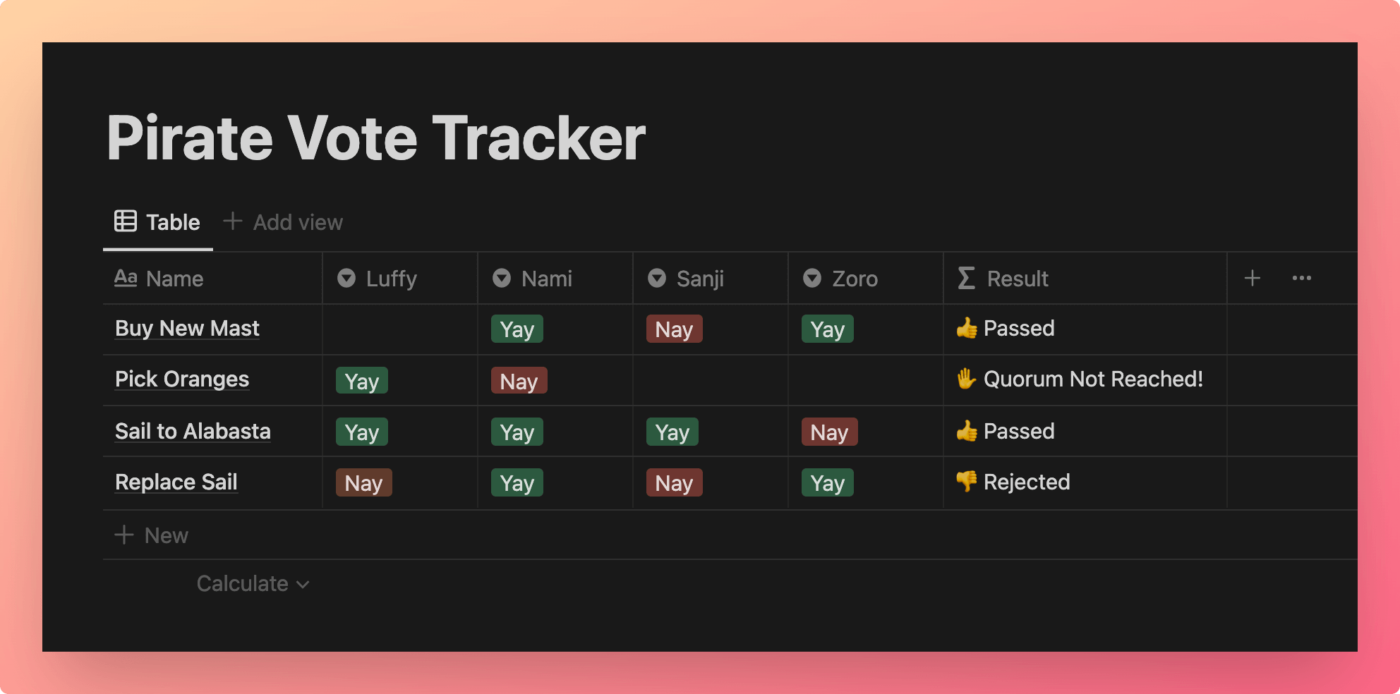
View and Duplicate Database
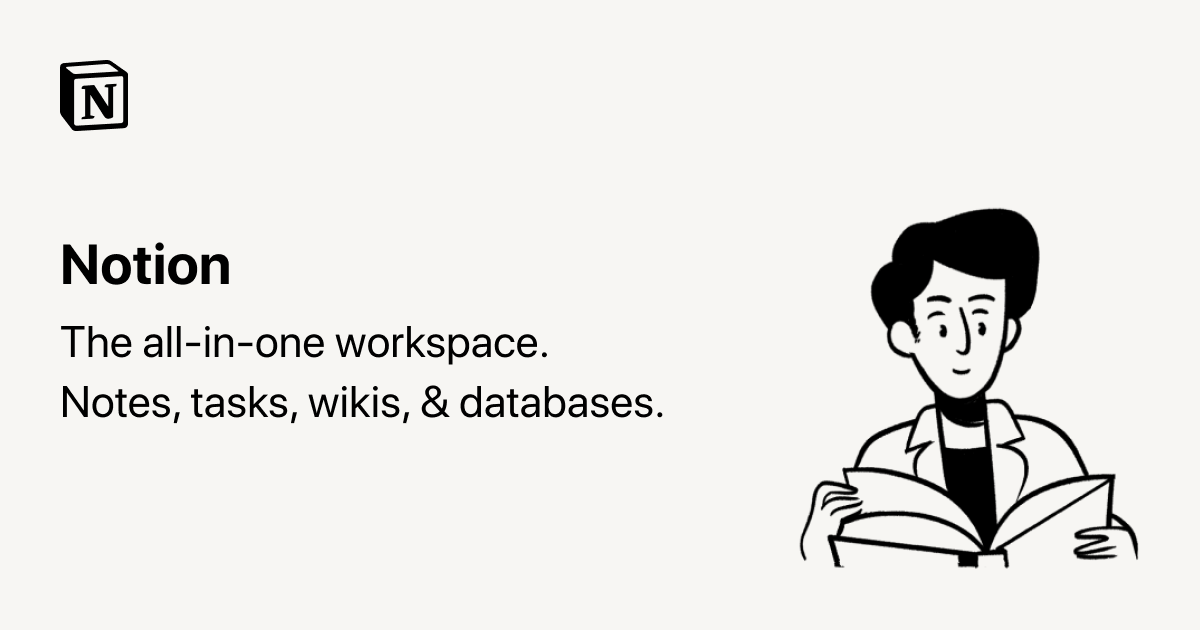
“Result” Property Formula
// Compressed
(+((not empty(prop("Luffy"))) ? true : false) + +((not empty(prop("Nami"))) ? true : false) + +((not empty(prop("Sanji"))) ? true : false) + +((not empty(prop("Zoro"))) ? true : false) < 3) ? "✋ Quorum Not Reached!" : ((+replaceAll(replaceAll(prop("Luffy"), "Nay", "-1"), "Yay", "1") + +replaceAll(replaceAll(prop("Nami"), "Nay", "-1"), "Yay", "1") + +replaceAll(replaceAll(prop("Sanji"), "Nay", "-1"), "Yay", "1") + +replaceAll(replaceAll(prop("Zoro"), "Nay", "-1"), "Yay", "1") < 1) ? "👎 Rejected" : "👍 Passed")
// Expanded
(
+((not empty(prop("Luffy"))) ? true : false) +
+((not empty(prop("Nami"))) ? true : false) +
+((not empty(prop("Sanji"))) ? true : false) +
+((not empty(prop("Zoro"))) ? true : false) < 3
) ?
"✋ Quorum Not Reached!" :
(
(+replaceAll(replaceAll(prop("Luffy"), "Nay", "-1"), "Yay", "1") +
+replaceAll(replaceAll(prop("Nami"), "Nay", "-1"), "Yay", "1") +
+replaceAll(replaceAll(prop("Sanji"), "Nay", "-1"), "Yay", "1") +
+replaceAll(replaceAll(prop("Zoro"), "Nay", "-1"), "Yay", "1") < 1) ?
"👎 Rejected" :
"👍 Passed"
)
Code language: JavaScript (javascript)
This vote tracker works by giving each group member their own Yay/Nay property. A Select property is used because there are actually three potential states for each crew member’s vote:
- Yay
- Nay
- Abstained/didn’t vote
Therefore, a Boolean/Checkbox property won’t work here.
The formula first checks that each member’s vote is not empty, using the empty function and the not operator. “Not empty” returns a value of true
, which is converted to a score of 1
using unaryPlus. Similarly, an empty property will return a false
value, which is converted to 0
.
The scores are added up, and if they total less than three (< 3
), the formula outputs “✋ Quorum Not Reached!”
If they total 3 or higher, we move to the “then” clause, which is a nested if-then statement.
Here, we use a pair of nested replaceAll functions to convert each member’s vote into a score: (+replaceAll(replaceAll(prop("Luffy"), "Nay", "-1"), "Yay", "1")
.
replaceAll()
is a function that searches a string for a pattern, then replaces all instances of that pattern with another pattern:
- The first
replaceAll()
searches the voter’s property (e.g.prop("Luffy")
) for “Nay”. If found, “Nay” is converted to “-1” (which is a string value at this point). - The second (outer)
replaceAll()
searches the result of the innerreplaceAll()
(which will either contain “Yay” or “-1” at this point) for “Yay”. If found, “Yay” is converted to “1”. - Now the vote’s text has been turned either to “-1” or “1”.
Next, the +
(unaryPlus) operator converts this numeric string to an actual number. This is done for each voter’s property, and finally all the scores are added up.
If the score is less than 1 (<1
), then the formula outputs, “👎 Rejected”. If it is 1 or greater, it outputs, “👍 Passed”.
Other formula components used in this example:
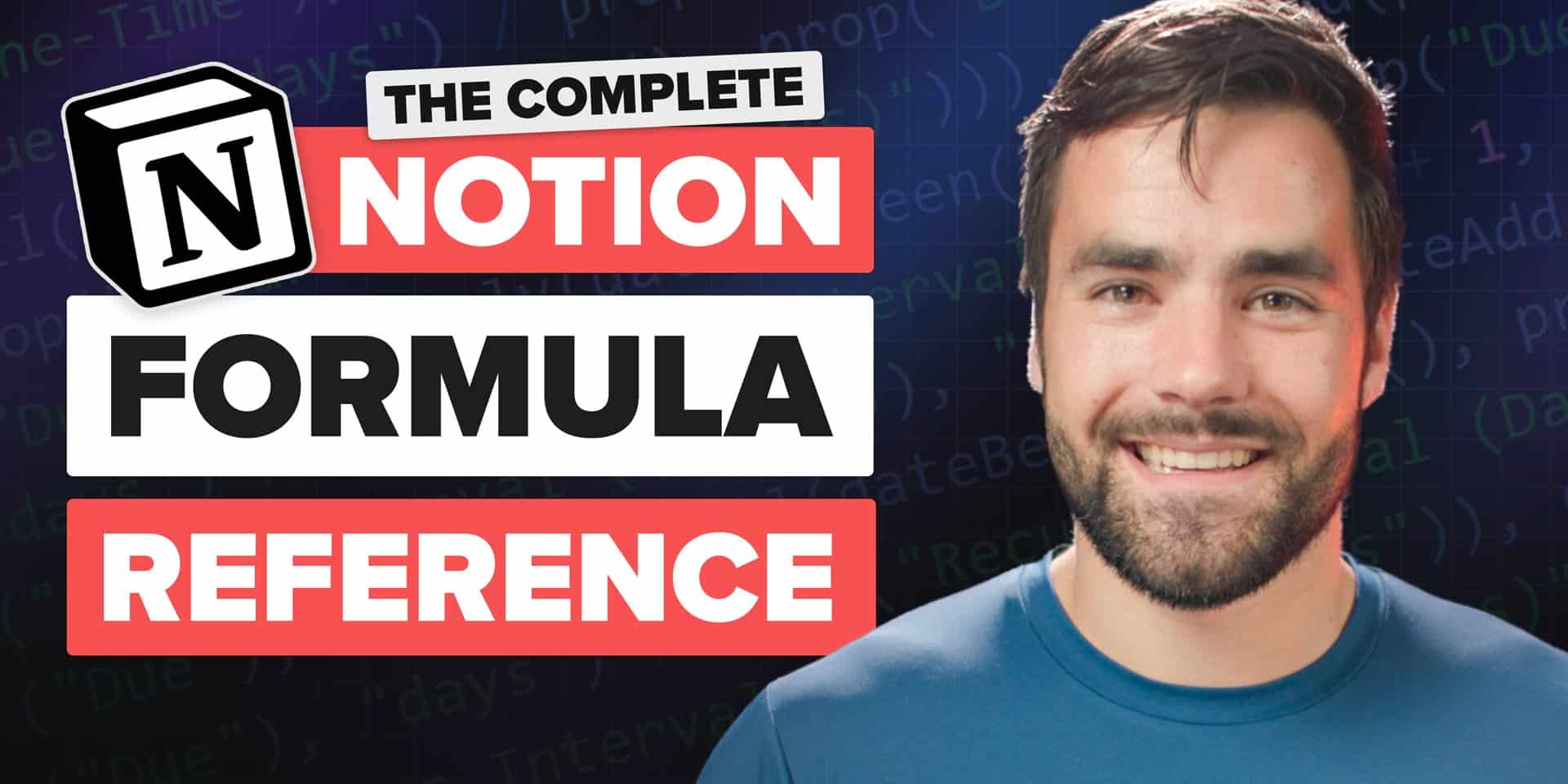
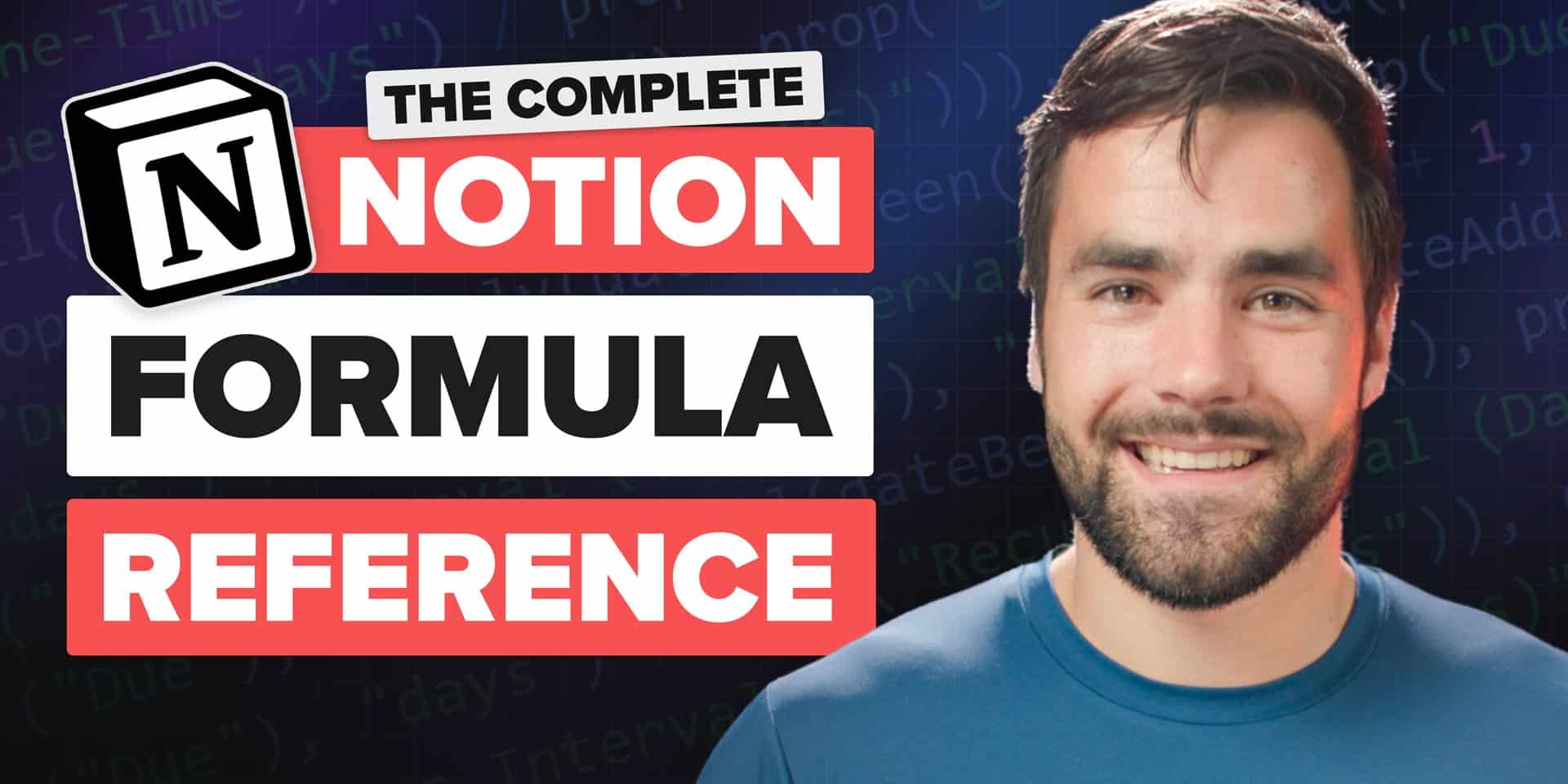
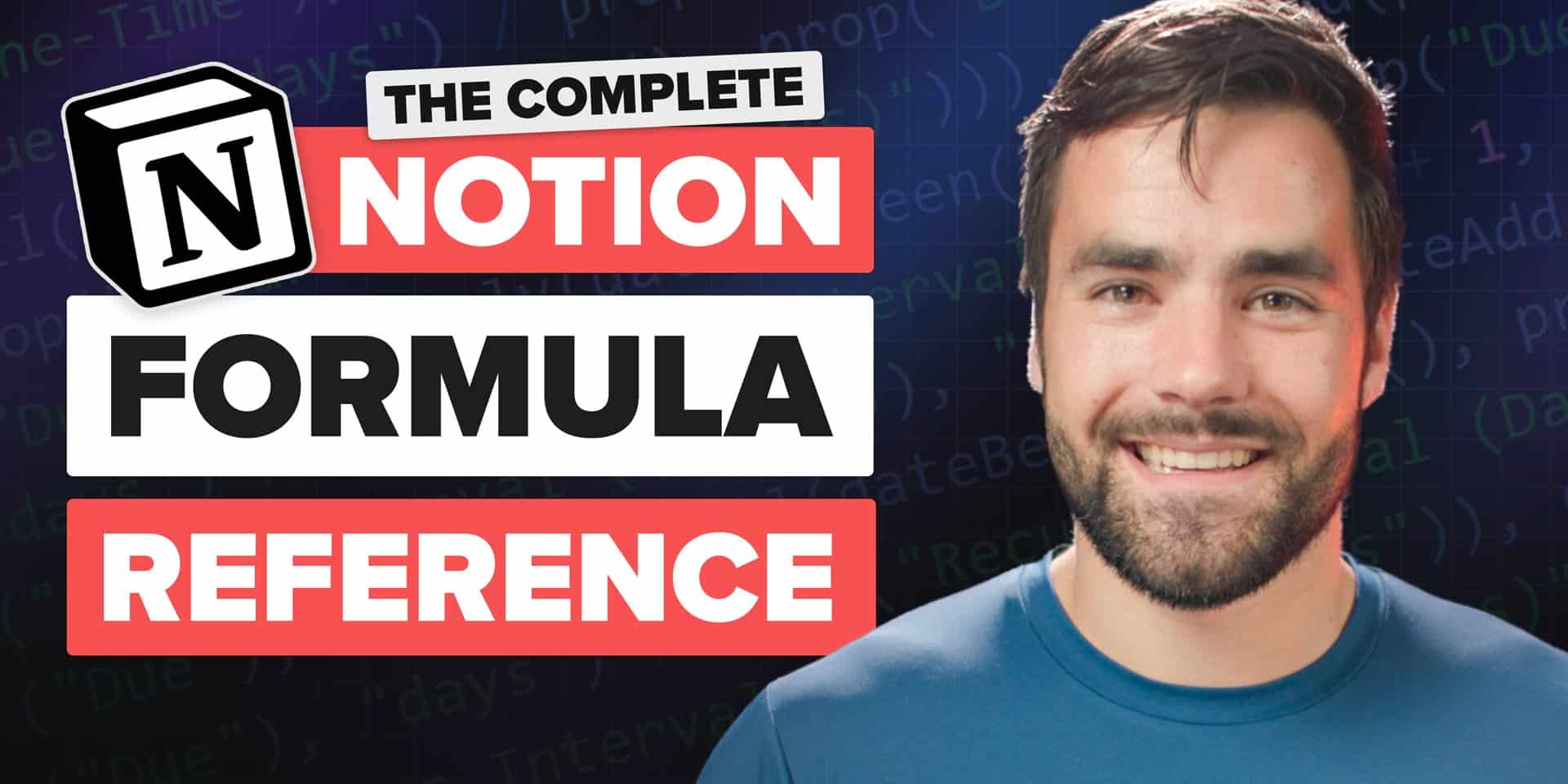
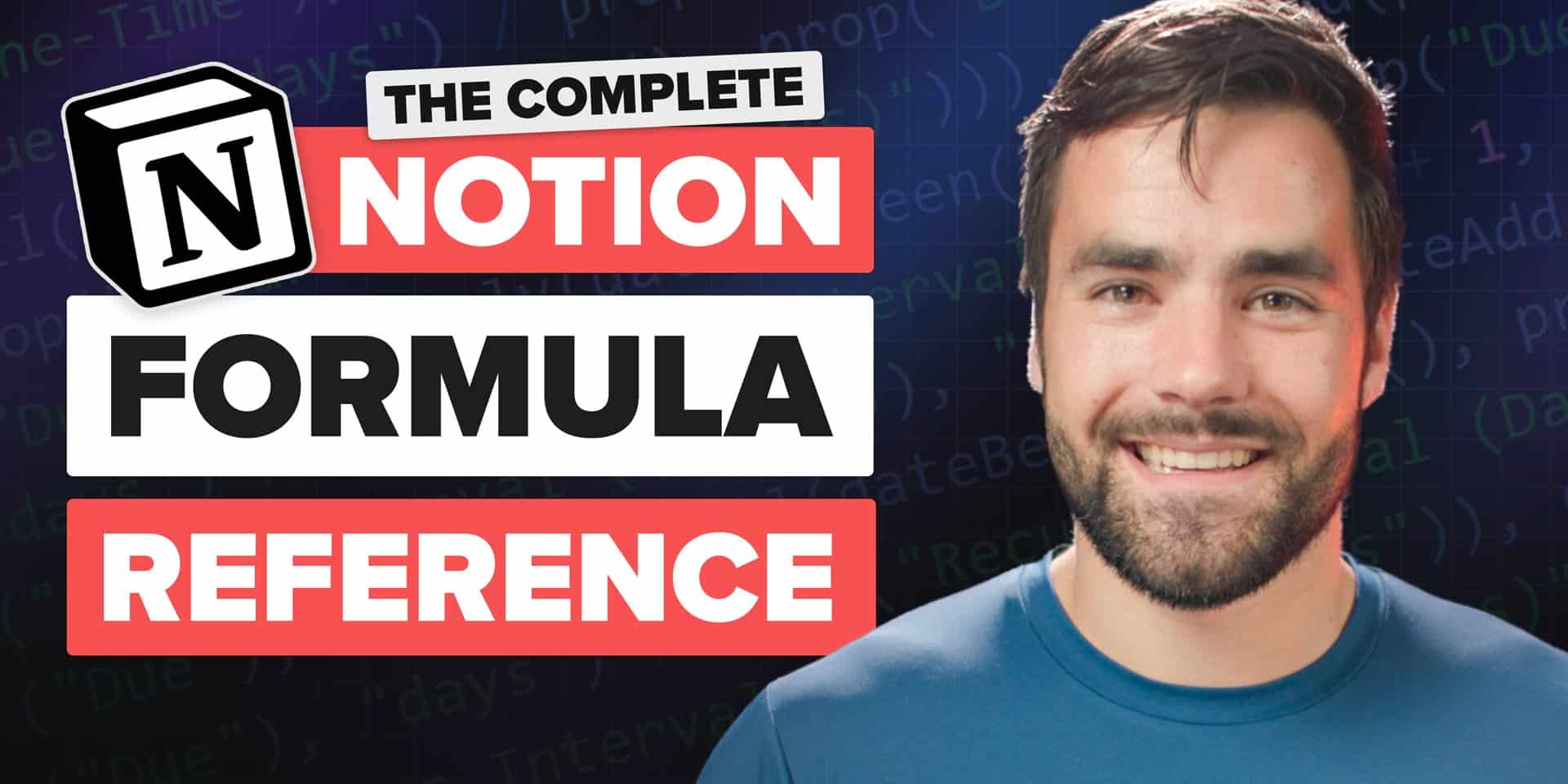
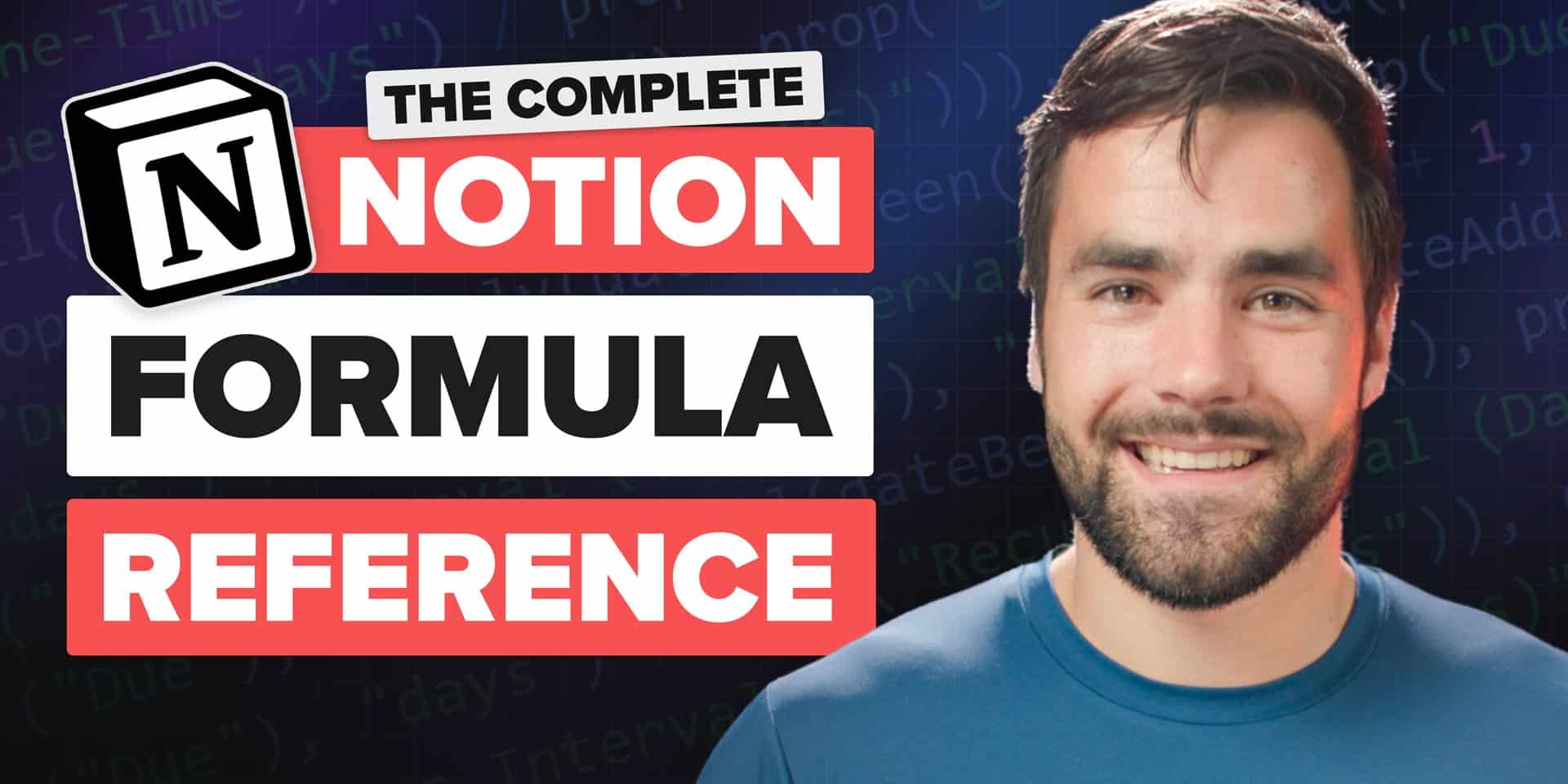