The greater than or equal (>=
) operator returns true if its left operand is greater than or equal to its right operand. It accepts numeric, string, date, and Boolean operands.
number >= number
string >= string
Boolean >= Boolean
date >= date
Code language: JavaScript (javascript)
Example Formulas
2 >= 1 /* Output: true */
42 >= 42 /* Output: true */
"b" >= "a" /* Output: true */
/* Boolean values equate to 1 (true) and 0 (false). */
true >= false /* Output: true */
true >= true /* Output: true */
/* For dates, "less than" equates to "before". */
now() >= now() /* Output: true */
Code language: JavaScript (javascript)
Good to know: When comparing dates, “greater” = “later”.
Good to know: The greater than pr equal (>=
) operator cannot be chained in a Notion formula. A formula like 3 >= 2 >= 1
won’t work. Use the and operator to get around this – e.g. 3
>= 2 and 2 >= 1
.
Example Database
This example database records the powerlifting totals for a few lifters and compares them against the USPA standards for different lifting levels. The Level formula outputs the lifter’s current level.
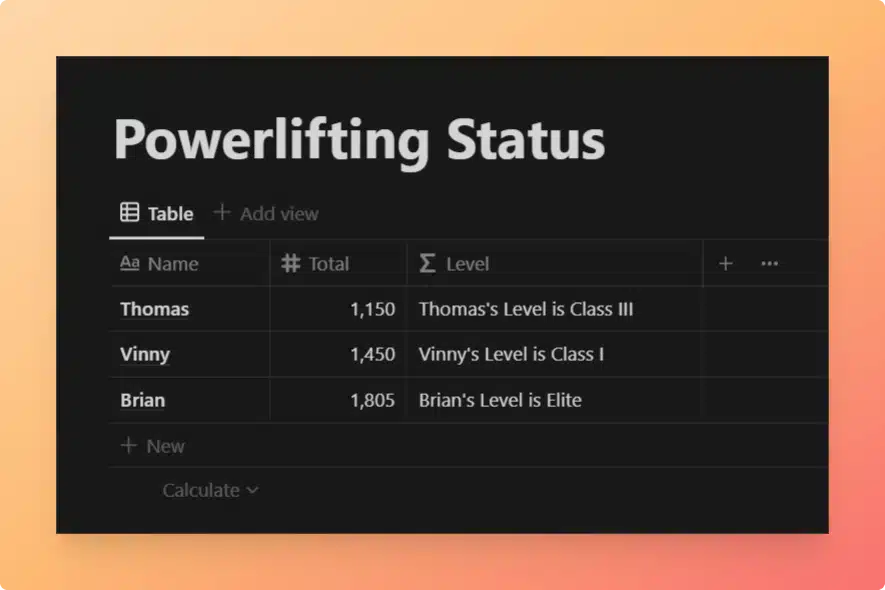
View and Duplicate Database
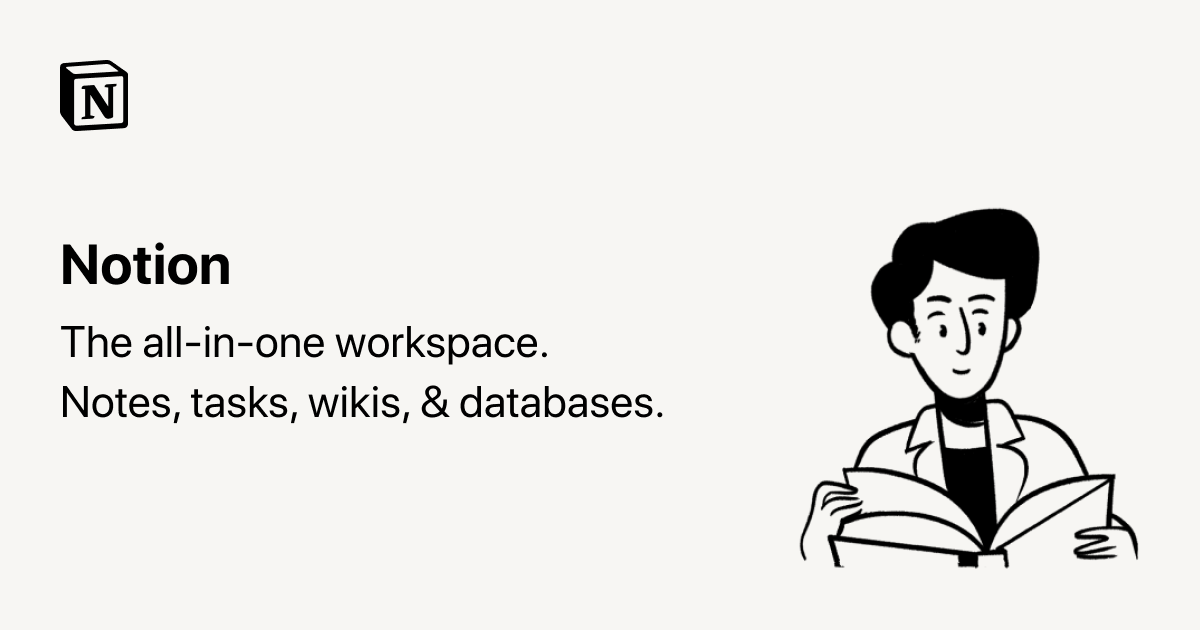
Explanation
// Compressed
prop("Name") + "'s Level is " + ((prop("Total") >= 1642) ? "Elite" : ((prop("Total") >= 1508) ? "Master" : ((prop("Total") >= 1355) ? "Class I" : ((prop("Total") >= 1191) ? "Class II" : ((prop("Total") >= 1041) ? "Class III" : ((prop("Total") >= 903) ? "Class IV" : "Novice"))))))
// Expanded
prop("Name") + "'s Level is " + (
(prop("Total") >= 1642) ? "Elite" :
((prop("Total") >= 1508) ? "Master" :
((prop("Total") >= 1355) ? "Class I" :
((prop("Total") >= 1191) ? "Class II" :
((prop("Total") >= 1041) ? "Class III" :
((prop("Total") >= 903) ? "Class IV" : "Novice")
)))))
Code language: JavaScript (javascript)
This formula uses a series of nested if-then statements (using the conditional operators ?
and :
) to check the numeric value in the Total property against the minimum requirements for USPA powerlifting levels (specifically, those for the raw 220 weight class).
Other formula components used in this example:
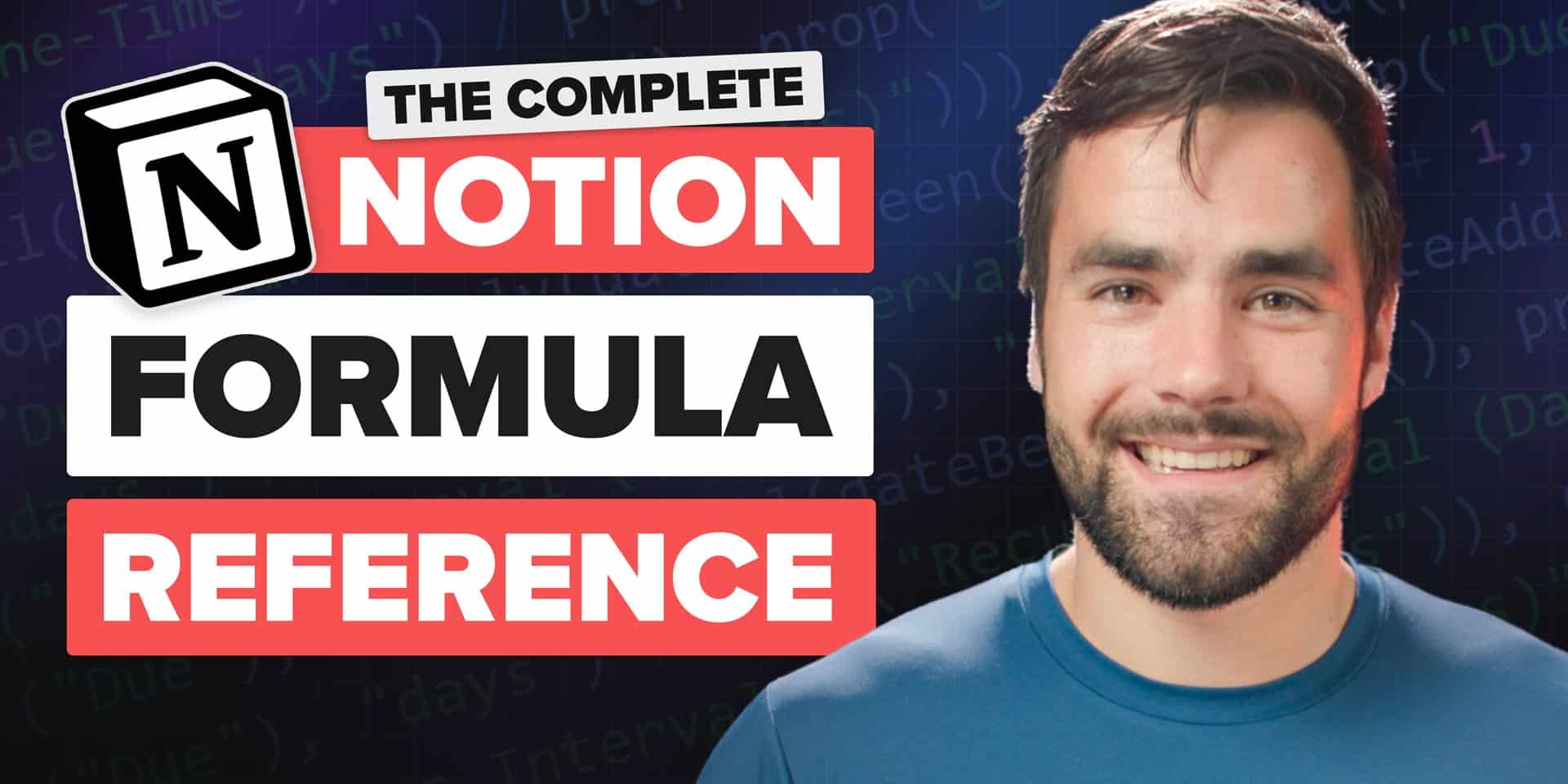
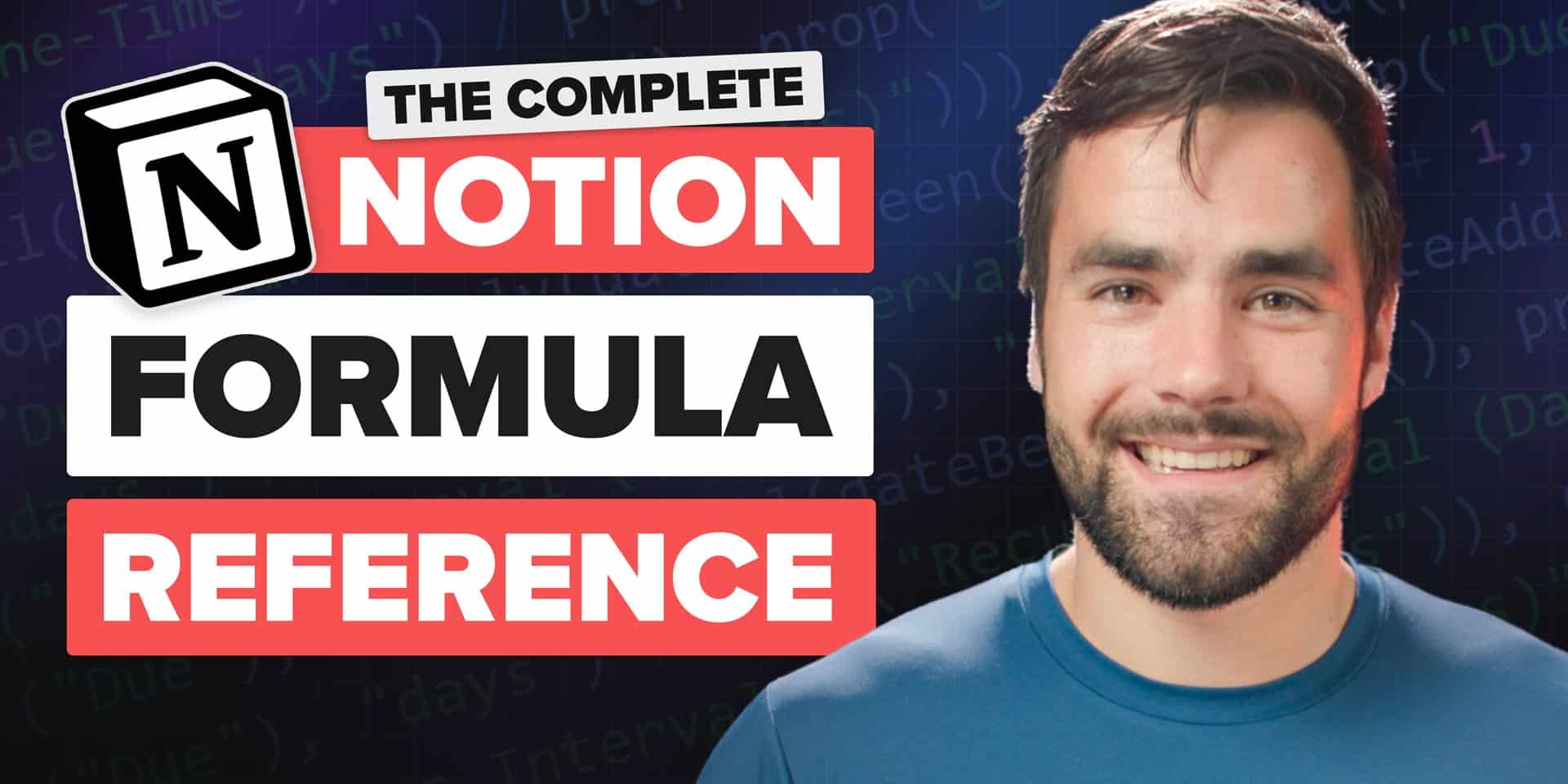